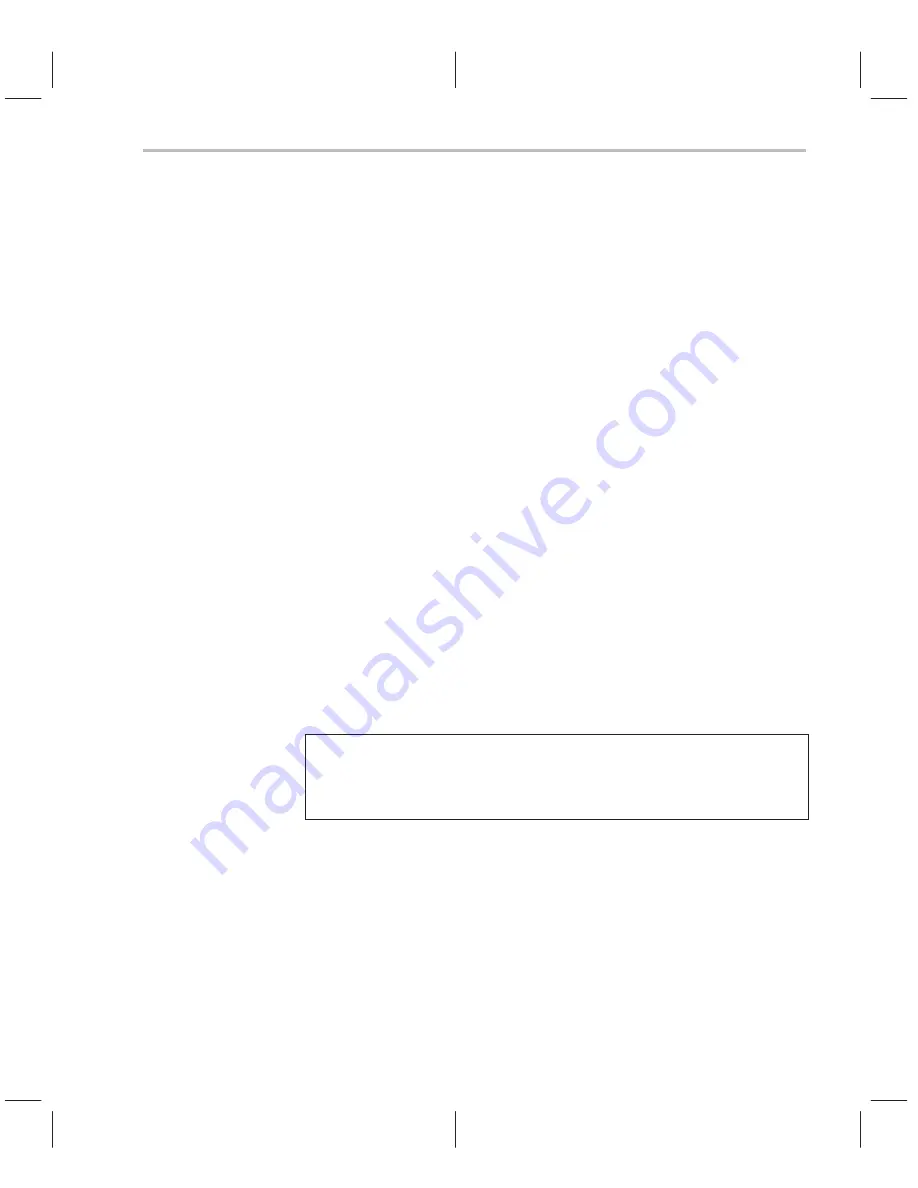
Compiling C/C++ Code
3-9
Optimizing C/C++ Code
The dependency graph in Figure 3–1 shows that:
-
The paths from sum[i] back to in1[i] and in2[i] indicate that writing to sum
may have an effect on the memory pointed to by either in1 or in2.
-
A read from in1 or
in2 cannot begin until the write to sum finishes, which
creates an aliasing problem. Aliasing occurs when two pointers can point
to the same memory location. For example, if vecsum( ) is called in a pro-
gram with the following statements, in1 and sum alias each other because
they both point to the same memory location:
short a[10], b[10];
vecsum(a, a, b, 10);
3.2.2.1
The Restrict Keyword
To help the compiler determine memory dependencies, you can qualify a
pointer, reference, or array with the restrict keyword. The restrict keyword is
a type qualifier that may be applied to pointers, references, and arrays. Its use
represents a guarantee by the programmer that within the scope of the pointer
declaration, the object pointed to can be accessed only by that pointer. Any
violation of this guarantee renders the program undefined. This practice helps
the compiler optimize certain sections of code because aliasing information
can be more easily determined.
In the example that follows, you can use the restrict keyword to tell the compiler
that a and b never point to the same object in foo (and the objects’ memory that
foo accesses does not overlap).
Example 3–2.Use of the Restrict Type Qualifier With Pointers
void foo(int * restrict a, int * restrict b)
{
/* foo’s code here */
}
This example is a use of the restrict keyword when passing arrays to a function.
Here, the arrays c and d should not overlap, nor should c and d point to the
same array.