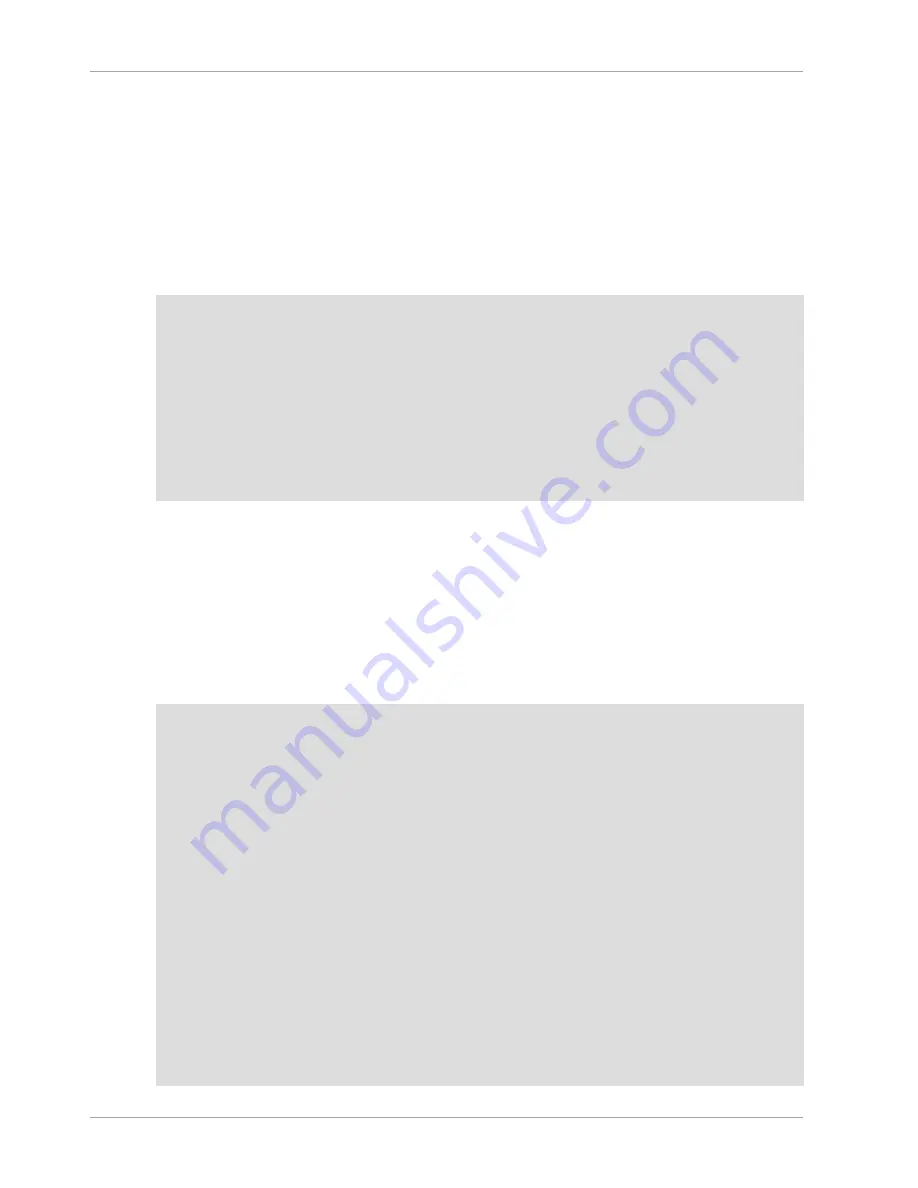
C API Function Descriptions
2164
In a nonmulti-threaded environment, the call to
mysql_library_init()
may be omitted, because
mysql_init()
will invoke it automatically as necessary. However,
mysql_library_init()
is
not thread-safe in a multi-threaded environment, and thus neither is
mysql_init()
, which calls
mysql_library_init()
. You must either call
mysql_library_init()
prior to spawning any
threads, or else use a mutex to protect the call, whether you invoke
mysql_library_init()
or
indirectly through
mysql_init()
. Do this prior to any other client library call.
The
argc
and
argv
arguments are analogous to the arguments to
main()
, and enable passing of
options to the embedded server. For convenience,
argc
may be
0
(zero) if there are no command-
line arguments for the server. This is the usual case for applications intended for use only as regular
(nonembedded) clients, and the call typically is written as
mysql_library_init(0, NULL, NULL)
.
#include <mysql.h>
#include <stdlib.h>
int main(void) {
if (mysql_library_init(0, NULL, NULL)) {
fprintf(stderr, "could not initialize MySQL library\n");
exit(1);
}
/* Use any MySQL API functions here */
mysql_library_end();
return EXIT_SUCCESS;
}
When arguments are to be passed (
argc
is greater than
0
), the first element of
argv
is ignored (it
typically contains the program name).
mysql_library_init()
makes a copy of the arguments so it
is safe to destroy
argv
or
groups
after the call.
For embedded applications, if you want to connect to an external server without starting the embedded
server, you have to specify a negative value for
argc
.
The
groups
argument is an array of strings that indicate the groups in option files from which to
read options. See
Section 4.2.3.3, “Using Option Files”
. Make the final entry in the array
NULL
. For
convenience, if the
groups
argument itself is
NULL
, the
[server]
and
[embedded]
groups are used
by default.
#include <mysql.h>
#include <stdlib.h>
static char *server_args[] = {
"this_program", /* this string is not used */
"--datadir=.",
"--key_buffer_size=32M"
};
static char *server_groups[] = {
"embedded",
"server",
"this_program_SERVER",
(char *)NULL
};
int main(void) {
if (mysql_library_init(sizeof(server_args) / sizeof(char *),
server_args, server_groups)) {
fprintf(stderr, "could not initialize MySQL library\n");
exit(1);
}
/* Use any MySQL API functions here */
mysql_library_end();
return EXIT_SUCCESS;
}
Содержание 5.0
Страница 1: ...MySQL 5 0 Reference Manual ...
Страница 18: ...xviii ...
Страница 60: ...40 ...
Страница 396: ...376 ...
Страница 578: ...558 ...
Страница 636: ...616 ...
Страница 844: ...824 ...
Страница 1234: ...1214 ...
Страница 1426: ...MySQL Proxy Scripting 1406 The following diagram shows an overview of the classes exposed by MySQL Proxy ...
Страница 1427: ...MySQL Proxy Scripting 1407 ...
Страница 1734: ...1714 ...
Страница 1752: ...1732 ...
Страница 1783: ...Configuring Connector ODBC 1763 ...
Страница 1793: ...Connector ODBC Examples 1773 ...
Страница 1839: ...Connector Net Installation 1819 2 You must choose the type of installation to perform ...
Страница 1842: ...Connector Net Installation 1822 5 Once the installation has been completed click Finish to exit the installer ...
Страница 1864: ...Connector Net Visual Studio Integration 1844 Figure 20 24 Debug Stepping Figure 20 25 Function Stepping 1 of 2 ...
Страница 2850: ...2830 ...
Страница 2854: ...2834 ...
Страница 2928: ...2908 ...
Страница 3000: ...2980 ...
Страница 3122: ...3102 ...
Страница 3126: ...3106 ...
Страница 3174: ...3154 ...
Страница 3232: ...3212 ...