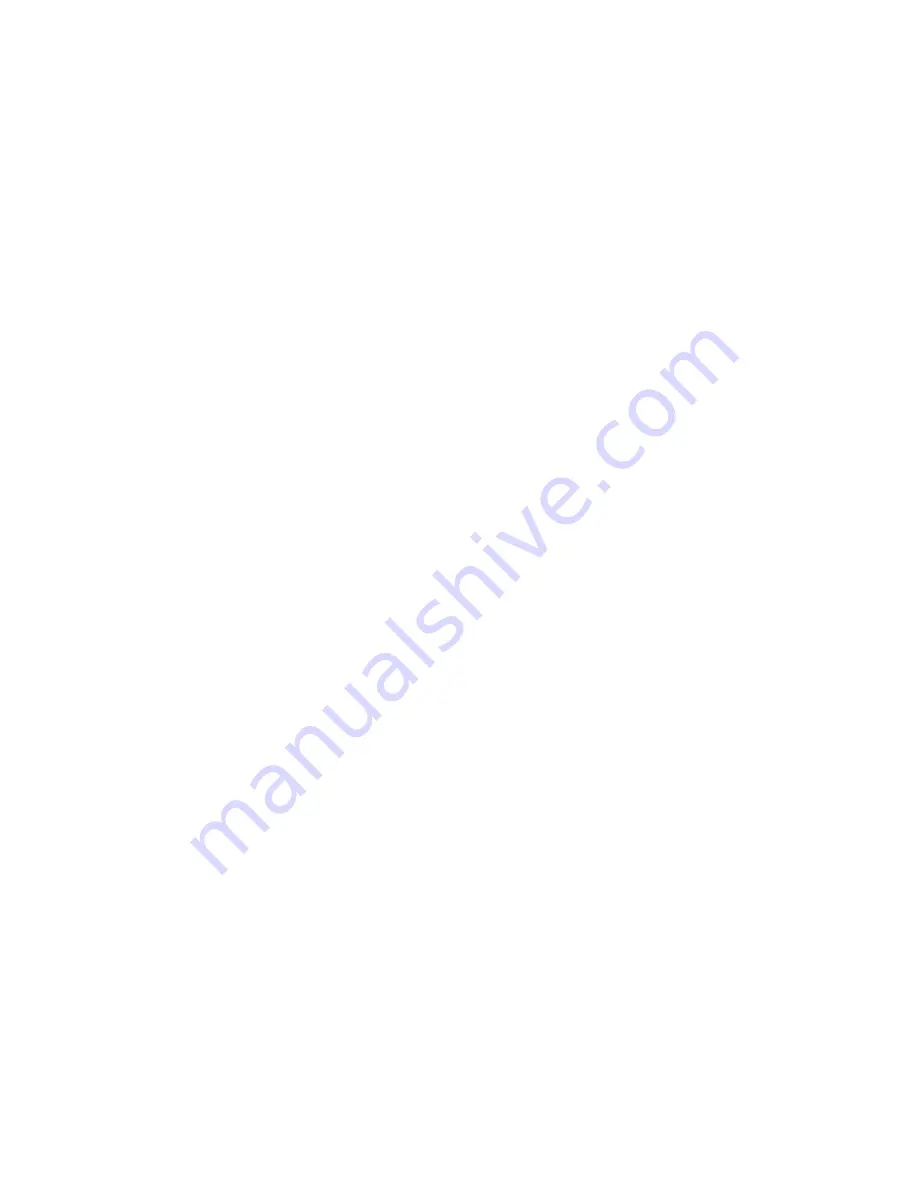
if((current_val[channel] ^ 0x8000) > (high_val
[channel] ^ 0x8000))
high_val[channel] = current_val[channel];
}
else
{
if(current_val[channel] < low_val[channel])
low_val[channel] = current_val[channel];
if(current_val[channel] > high_val[channel])
high_val[channel] = current_val[channel];
}
}
gotoxy(10,c 4);
printf("%04X %04X %04X ",current_val[channel],high_val
[channel],low_val[channel]);
printf("%04X ",high_val[channel] - low_val[channel]);
if(mode & 1) /* If signed mode, adjust values when printing */
{
printf("%8.4f ",(((float)(current_val[channel] ^ 0x8000)
/ (float)full_count) * full_scale) - offset_val);
printf("%8.4f ",(((float)(high_val[channel] ^ 0x8000) /
(float)full_count) * full_scale) - offset_val);
printf("%8.4f ",(((float)(low_val[channel] ^ 0x8000) /
(float)full_count) * full_scale) - offset_val);
printf("%8.4f ",((float)((high_val[channel] ^ 0x8000) -
(low_val[channel]) ^ 0x8000) / (float)full_count) * full_scale);
}
else
{
printf("%8.4f ",(((float)current_val[channel] / (float)
full_count) * full_scale) - offset_val);
printf("%8.4f ",(((float)high_val[channel] / (float)
full_count) * full_scale) - offset_val);
printf("%8.4f ",(((float)low_val[channel] / (float)
full_count) * full_scale) - offset_val);
printf("%8.4f ",((float)(high_val[channel] - low_val
[channel]) / (float)full_count) * full_scale);
}
if(channel < (max_channel-1))
printf(" ");
/* In interrupt mode, display a counter of the number of
interrupts that have occured.
*/
if(interrupt_mode)
{
gotoxy(65,25);
printf("%ld",interrupt_count);
}
}
}
/* Be sure to restore the original interrupt state before exit */
disable();
outportb(0x21,inportb(0x21) | IRQ_MASK);
setvect(IRQ_VECTOR,old_isr);
enable();