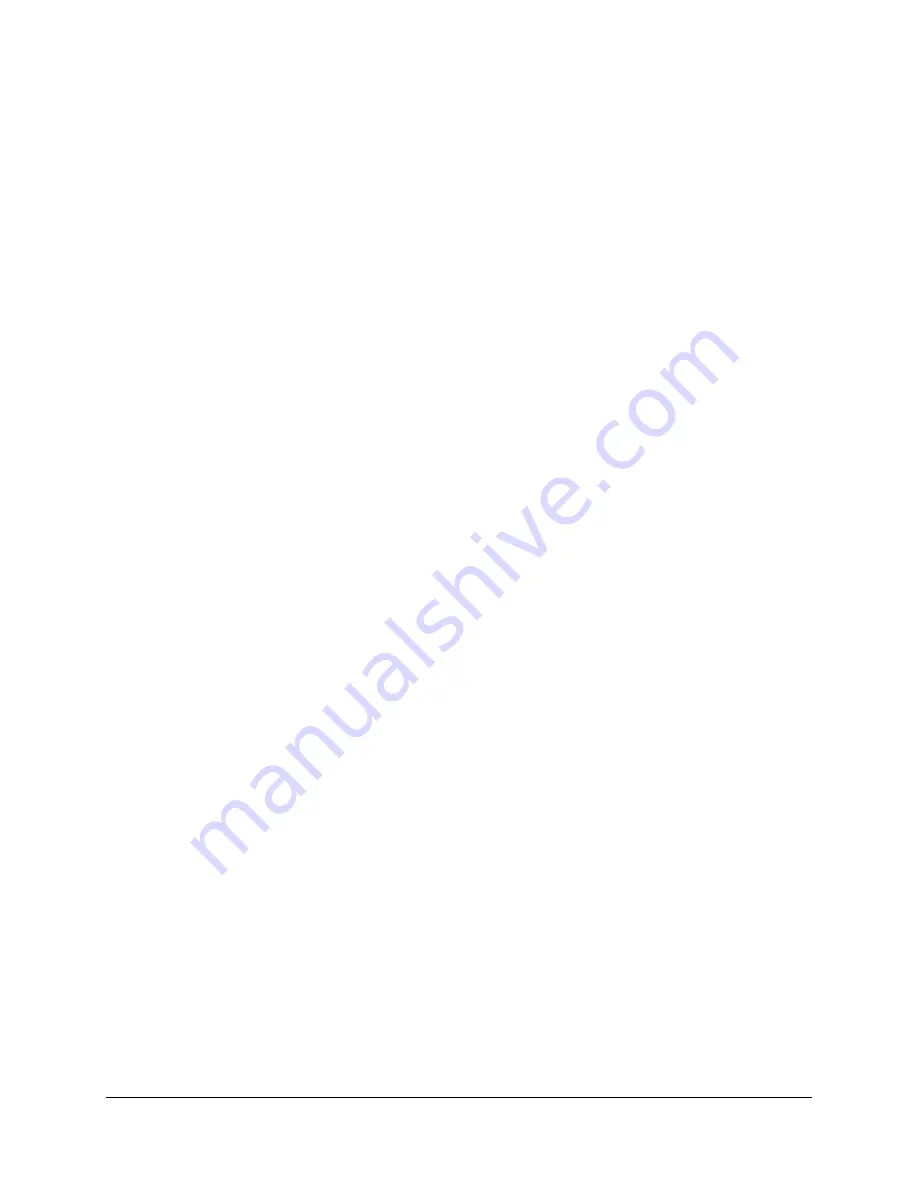
www.vtiinstruments.com
APPENDIX C
95
These are the actual opcodes for four of the five functions we outlined above. They are 1-wire bus
commands that are sent to the 1-wire (TEDS) devices. The GET_URN function is not listed because
it is not a single opcode that modifies data on the device or returns data except for the serial
number/URN.
//Device-specific values
#define DS2430_SCRATCHPAD_LEN 32
#define DS2431_SCRATCHPAD_LEN 8
#define DS2430_MEMORY_LEN 32
#define DS2431_MEMORY_LEN 144
uint8_t SendPkt[256];
uint8_t RecPkt[256];
These are global buffers which will be used to store the sent and received packets.
Before beginning with the listed functions, a short example will be examined and described in detail.
int example_function(
string
name)
{
CComSafeArray<
short
> WriteBuf;
WriteBuf.Create();
WriteBuf.Add((
short
)0);
// reserve first byte for length
// clear the search state so we find the 'first' device
WriteBuf.Add(CMD_RESET);
WriteBuf.Add(CMD_GETBUF);
// set the length
WriteBuf[0] = (
short
)WriteBuf.GetCount() - 1;
// send the commands
digitizer->Channels->Item[name]->TEDS-
>WriteTEDS(WriteBuf.GetSafeArrayPtr());
// retrieve the response
CComSafeArray<
short
> ReadBuf;
SAFEARRAY* psaReadBuf;
digitizer->Channels->Item[name]->TEDS-
>ReadTEDS(&psaReadBuf);
}
This function performs an MLAN bus master reset, that is, it resets the MLAN repeater inside the
instrument. As can be seen, the first byte of the packet is reserved for the length of the packet. This
not only defines a maximum size for an MLAN packet, but also tells the controller how much space
to allocate for it. This is done for every packet sent. The controller also uses the first byte as the
length of every packet received. Command and data bytes are appended to the byte array, with a
post-increment of the index (sendLen).
The first command sent is CMD_RESET, or 0x84.
This is the command that performs the bus
master reset. The next command is CMD_GETBUF, or 0x84. This returns the response buffer from
the repeater.
Here is the program’s output, given just this function:
sent packet without errors
Packet length: 3
02 84 85
got a packet without errors on receive
Packet length: 3