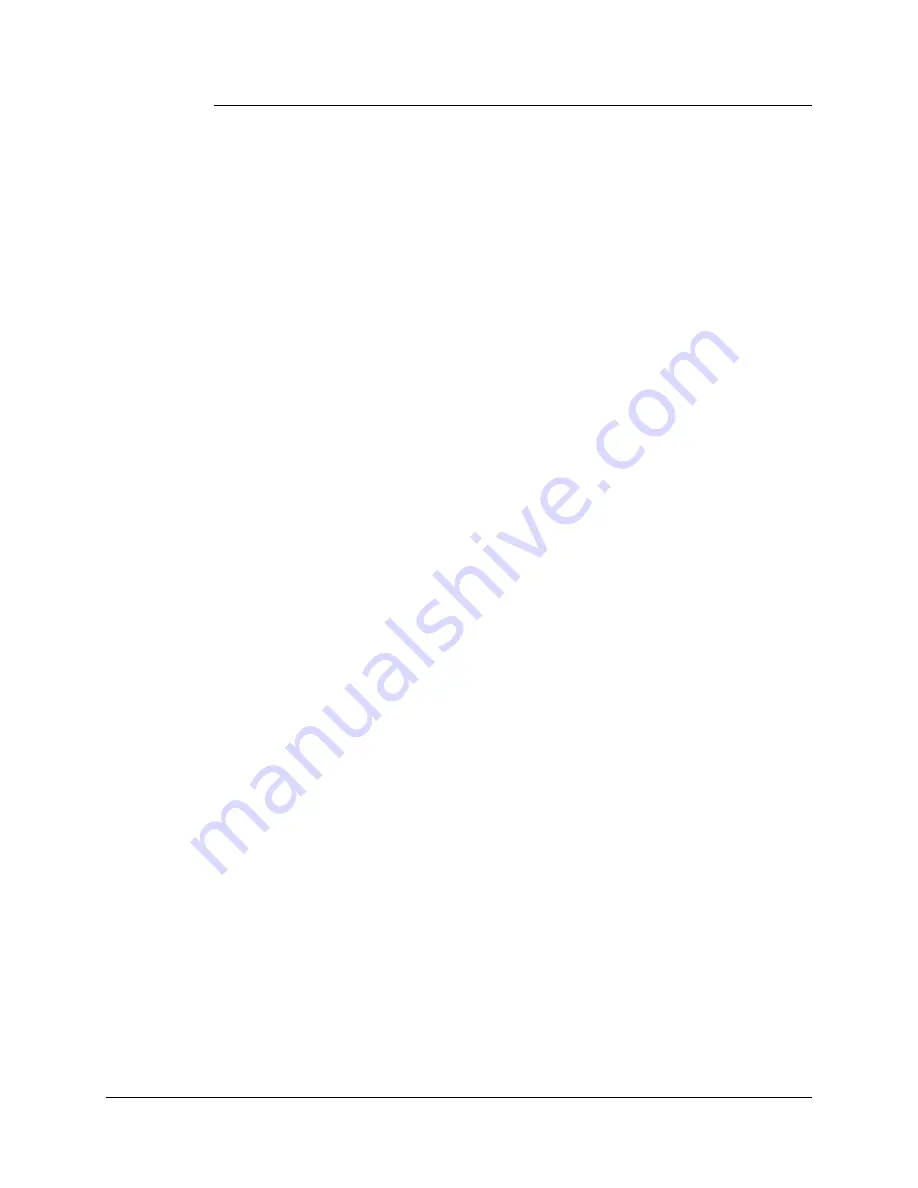
VTI Instruments Corp.
APPENDIX C
100
READ_SCRATCHPAD_2430
The READ_SCRATCHPAD command is nearly identical between the DS2430 and DS2431, but
some differences exist. The DS2430 will be covered first.
int
read_scratchpad_2430(IVTEXDigitizerPtr digitizer)
{
int
recLen = 0;
CComSafeArray<
short
> WriteBuf;
WriteBuf.Create();
WriteBuf.Add((
short
)0);
// reserve first byte for length
// access the current device with address in DATA_ID
WriteBuf.Add(CMD_ML_ACCESS);
// construct a block of communication to MicroLAN
WriteBuf.Add(CMD_ML_DATA);
WriteBuf.Add(3);
// block length
WriteBuf.Add(2+DS2430_SCRATCHPAD_LEN);
// data length of read
// send the read scratchpad command
WriteBuf.Add(READ_SCRATCHPAD);
// send the address byte
WriteBuf.Add((
short
)0);
// request the result buffer as the last command
WriteBuf.Add(CMD_GETBUF);
// set the length
WriteBuf[0] = (
short
)WriteBuf.GetCount() - 1;
// send the commands
digitizer->Channels->Item[name]->TEDS-
>WriteTEDS(WriteBuf.GetSafeArrayPtr());
std::cout <<
"DS2430 Read Scratchpad (WriteData)"
<<
std::endl;
PrintPacket(WriteBuf);
// retrieve the response
CComSafeArray<
short
> ReadBuf;
SAFEARRAY* psaReadBuf;
digitizer->Channels->Item[name]->TEDS->ReadTEDS(&psaReadBuf);
std::cout <<
"DS2430 Read Scratchpad (ReadData)"
<<
std::endl;
ReadBuf.Attach(psaReadBuf);
PrintPacket(ReadBuf);
recLen = (
int
)ReadBuf.GetCount();
return
recLen;
}
The first three commands are the same as the WRITE_SCRATCHPAD command. The device must
still be accessed and the controller must be put into data access mode. The block length, this time,
is only 3, because the expected buffer size, the READ_SCRATCHPAD command, and the address
to read from (always “0” for this example, but if less than the scratchpad length is read, this could
be incremented and read multiple times) is all that must be sent.
The expected data length is
2+SCRATCHPAD_LEN
. See WRITE_SCRATCHPAD_2430 for why
this length is used.