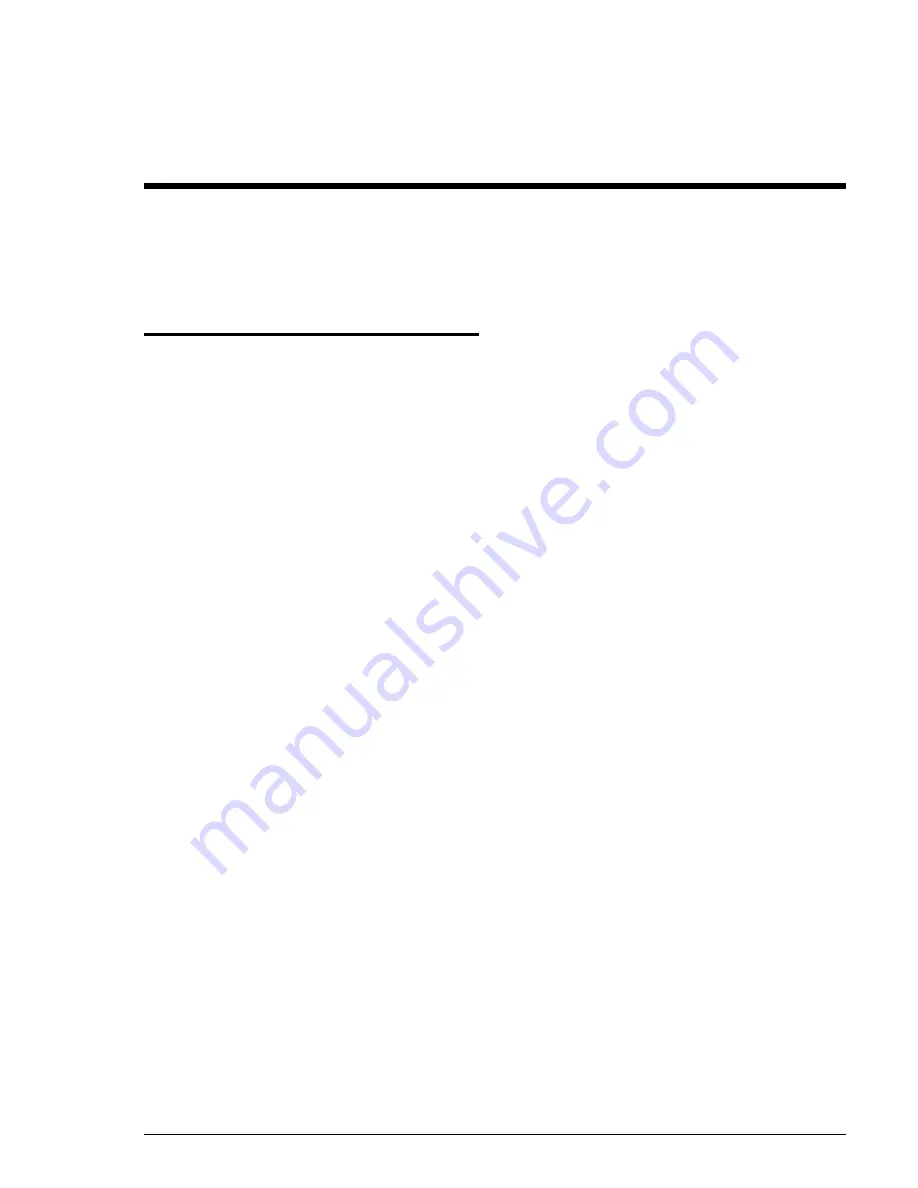
VL-1225/6 Analog Input/Output Board
6-1
Software Examples
This section shows some software examples written in Microsoft MASM 5.0 assembly language to assist
you in constructing your own software routines. The interrupt code example is written specifically for
use with VersaLogic’s 80188 CPU card, VL-188.
Polled Mode Analog Input
The following example reads channel 0 into the AX register. It is assumed that the board is addressed
at I/O location 0300H.
The key program sections are:
READ
Reads A/D channel 0 into AX register.
BUSY
Location where program loops waiting for A/D conversion to complete.
;VL-1225/6 REGISTER ADDRESSES
= 0300
istat
equ
00300h
;Interrupt Status Register
= 0300
ictrl
equ
00300h
;Interrupt Control Register
= 0303
select
equ
00303h
;Input Channel Select Register
= 0304
idlow
equ
00304h
;Input Data Low Register
= 0305
idhigh
equ
00305h
;Input Data High Register
= 0306
od0
equ
00306h
;Channel 0 Output Data Register
= 0307
od1
equ
00307h
;Channel 1 Output Data Register
0000
code
segment
para public ‘CODE’
assume
cs:code
0000
read:
;READ CHANNEL 0 INTO AX REGISTER
0000 BA 0303
mov
dx,select ;Select channel 0 and Trigger
0003 B0 00
mov
al,00h
0005 EE
out
dx,al
0006 BA 0305
mov
dx,idhigh ;Read BUSY bit and High Data
0009 EC
busy:
in
al,dx
000A A8 80
test
al,10000000b
000C 75 FB
jnz
busy
;Loop if BUSY = 1
000E 8A E0
mov
ah,al
;Mask off unused high-order bits
0010 80 E4 03
and
ah,03h
;(Use ‘ah,07h’ for 11 bit mode)
0013 BA 0304
mov
dx,idlow ;Read Data Low register second
0016 EC
in
al,dx
0017
signex:
;Sign extend to fill 16-bit register
;The following 3 instructions are
;optional. They are used in
;two’s complement mode only.
0017 B1 06
mov
cl,6
;Shift count (use ‘cl,5’ for 11-bit mode)
0019 D3 E0
sal
ax,cl
;Shift AD10 or AD11 into bit position D7
001B D3 F8
sar
ax,cl
;Shift it back, extending sign
001D
code
ends
;AX register contains A/D data
end
read
Software Examples