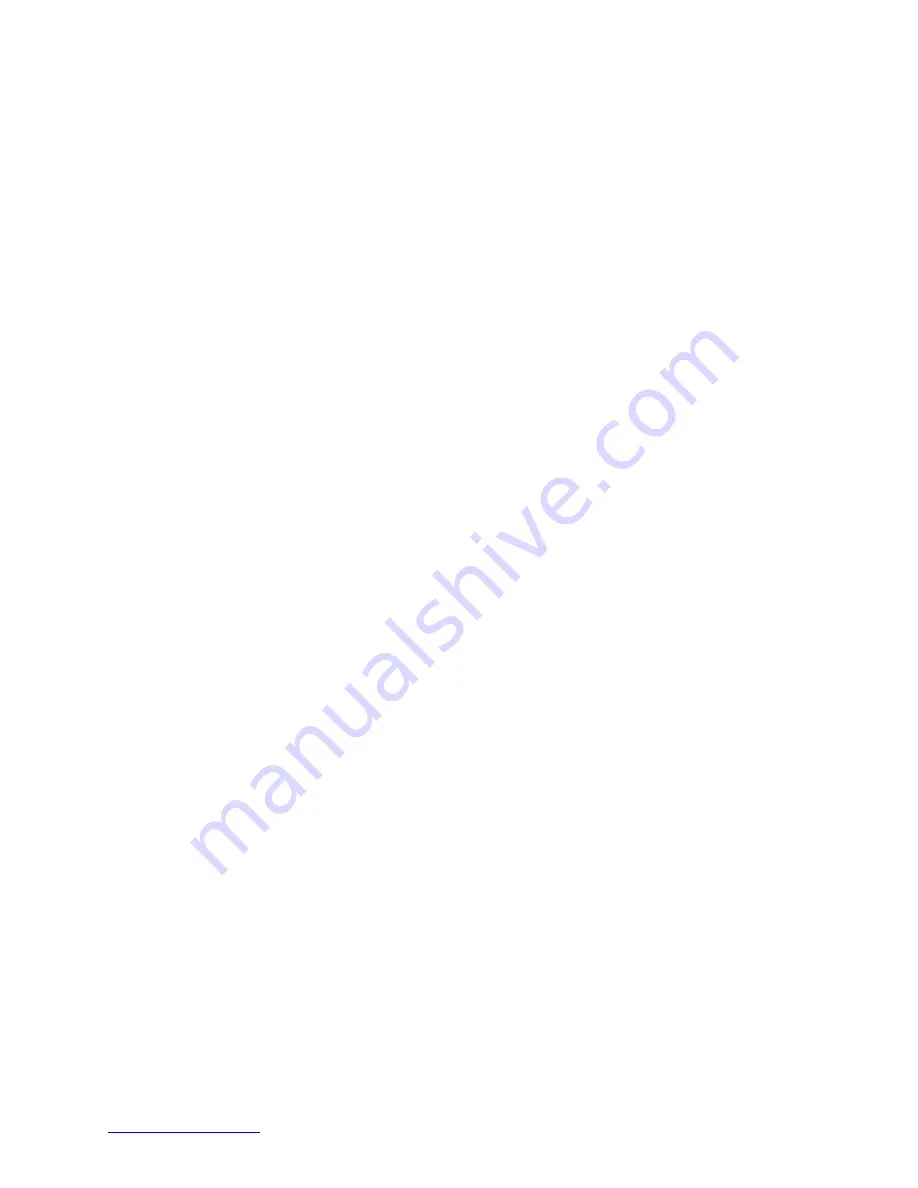
PulseBlasterESR-PRO
Making changes to an example program requires understanding of only a few lines of code. The
following C code example generates a 50% duty cycle square wave with a 400.0 ms period.
A breakdown of the previous C code segment is as follows:
•
Line 1:
Initialize communication with the selected board. This must be called before any other
functions that communicate with the board.
•
Line 2:
Set the internal block clock frequency (in MHz). This must be called to insure proper timings
in the pulse program.
•
Lines 7-10:
Programs the board's pulse program memory.
◦
Line 7
: pb_start_programming (PULSE_PROGRAM) must be called before using the
pb_inst(..) function.
◦
Line 8
, instruction 1: Turn on bit 0 for 200.0 ms then continue to the next instruction. The
address of this instruction is stored in the “start” variable.
▪
Note: If the output is high for more than 5 clock cycles, it is necessary to turn off the Short
Pulse feature by settings bits 21-23 of the flag bits to “111.” This can be easily accomplished
by using the C-macro “ON” defined in “spinapi.h.”
◦
Line 9
, instruction 2: All bits off for 200.0 ms, then branch to “start.”
◦
Line 10:
pb_stop_programming() must be called before calling any other SpinAPI functions.
•
Line 12:
Start the board executing the Pulse Program.
•
Line 13:
Close communication with the board (Pulse Program execution will continue).
2019/09/26
1: pb_init(); /*Initialize communication with the board*/
2: pb_core_clock (CLOCK); /*Set the internal clock frequency value – this
3: will be either 250, 300, 400, or 500 MHz
4: depending on your product */
5:
6: /*Start programming the Pulse Program*/
7: pb_start_programming (PULSE_PROGRAM);
8: start=pb_inst(ON|0x01, CONTINUE, 0, 200.0*ms); /*Bit 0 on, 200ms*/
9:
pb_inst(0x00, BRANCH, start, 200.0*ms);/*All bits off, 200ms*/
10: pb_stop_programming();
11:
12: pb_start(); /*Start the board executing*/
13: pb_close(); /*Close the communication with the board*/