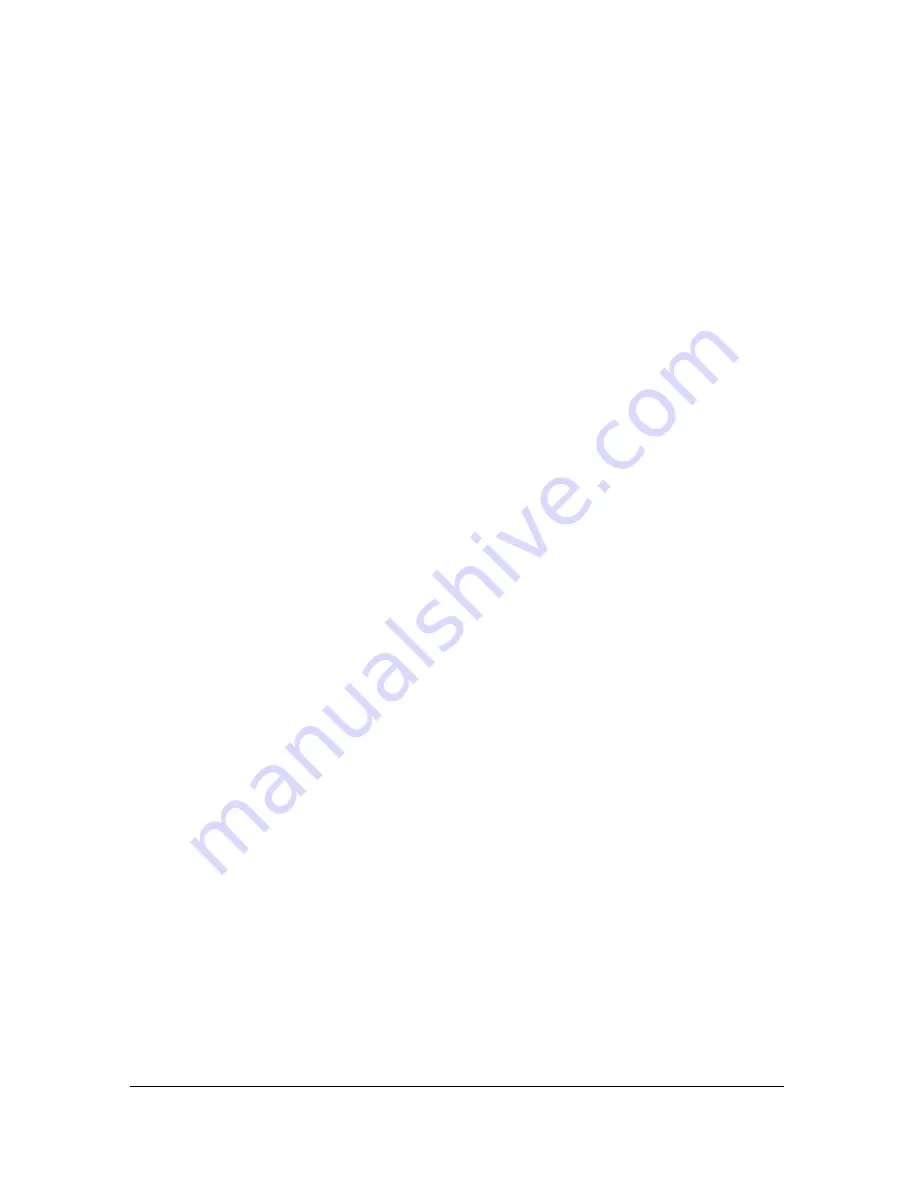
232
ActionScript language elements
myFunction = function () {
trace("this is myFunction");
};
try {
myInterval = setInterval(this, "myFunction", 1000);
throw new Error("my error");
}
catch (myError:Error) {
trace("error caught: "+myError);
}
finally {
clearInterval(myInterval);
trace("error is cleared");
}
In the following example, the
finally
block is used to delete an ActionScript object,
regardless of whether or not an error occurred. Create a new AS file called Account.as:
class Account {
var balance:Number = 1000;
function getAccountInfo():Number {
return (Math.round(Math.random() * 10) % 2);
}
}
In the same directory as Account.as, create a new AS or FLA document and enter the
following ActionScript in Frame 1 of the Timeline:
import Account;
var account:Account = new Account();
try {
var returnVal = account.getAccountInfo();
if (returnVal != 0) {
throw new Error("Error getting account information.");
}
}
finally {
if (account != null) {
delete account;
}
}
The following example demonstrates a
try..catch
statement. The code in the
try
block is
executed. If an exception is thrown by any code in the
try
block, control passes to the
catch
block, which shows the error message in a text field by using the
Error.toString()
method.
In the same directory as Account.as, create a new FLA document and enter the following
ActionScript in Frame 1 of the Timeline:
import Account;
var account:Account = new Account();
try {
Summary of Contents for FLASH 8-ACTIONSCRIPT 2.0 LANGUAGE
Page 1: ...ActionScript 2 0 Language Reference ...
Page 1352: ...1352 ActionScript classes ...