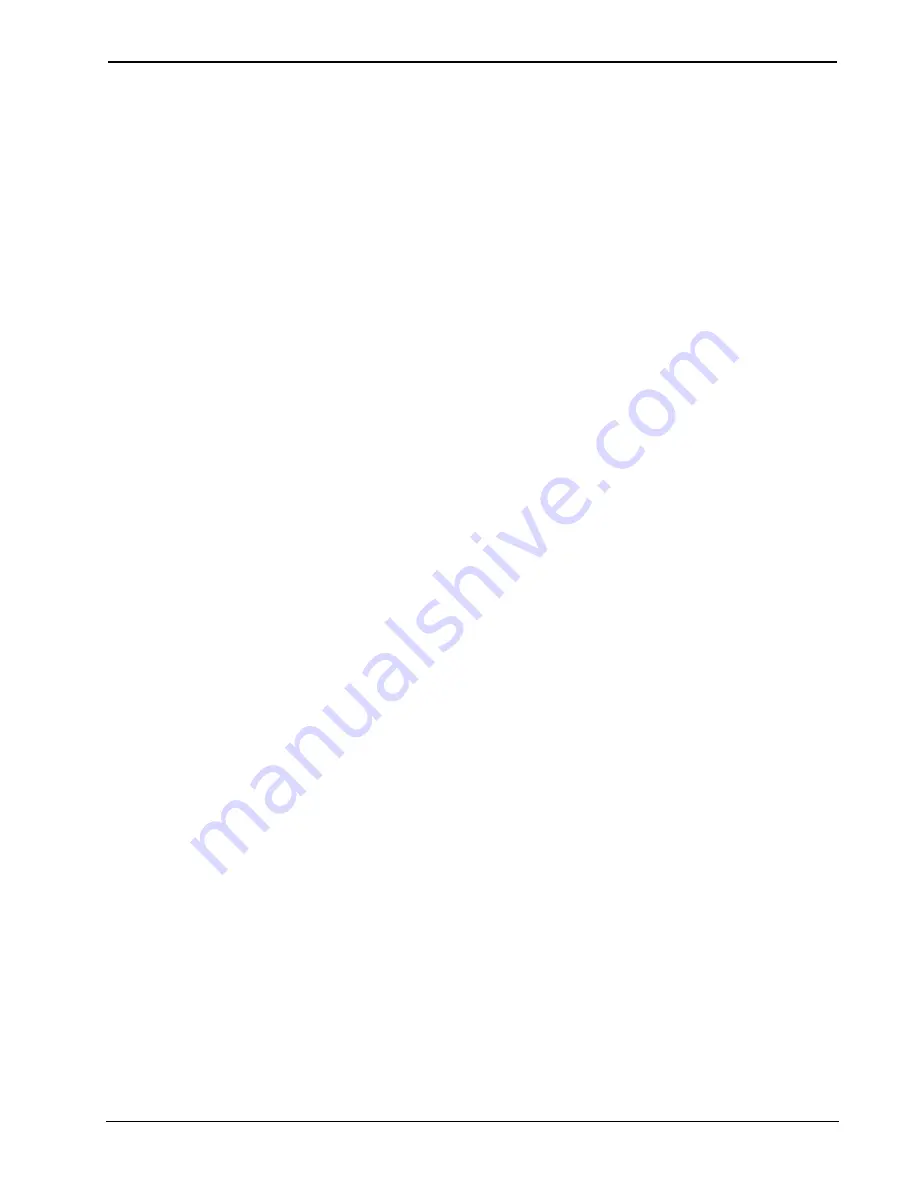
Crestron
SIMPL+
Software
Expressions
As reading through the later parts of this guide, as well as the latest revision of the
SIMPL+ Language Reference Guide (Doc. 5797), the term
expression
is mentioned
in many places. For example, in describing the syntax of the
if-else
construct, it may
be described as the following:
if (expression1)
{
// code to execute
}
else if (expression2)
{
// code the execute
}
In the above example, expression1 and expression2 can be any valid SIMPL+
expression. This section describes what is and what is not an expression.
An expression in SIMPL+ is anything that consists of operators and operands.
Operators were discussed previously in this section, and operands are simply the
things on which operators act. For example, refer to the following simple expression.
x + 5
In this expression the operator is the addition operator (+), and the operands are x
and 5. Expressions can contain constants, variables, and function calls in addition to
operators. One expression may be made up of many smaller expressions. The
following are all valid SIMPL+ expressions.
max(x,15)
y * x << z
a = 3
(26 + byte(aString,i) mod z = 25
Expressions can range from the very simple to the very complex. Also note that the
last two expressions contained an equal sign. It is very important to recognize that
this operator can have two different meanings based upon where it is used. In the
first example above, the equal sign can serve as an assignment operator (assign the
value 3 to the variable a) or as an equivalency comparison operator (does the
variable a equal 3?). However, an expression cannot contain an assignment (it would
then become a statement, discussed in “Statements” on page 24), so it is indeed
recognized as a comparison operation. In the second case, the equal sign also serves
as a equivalency comparison operator. Here there is no ambiguity since a value
cannot be assigned into an expression (as opposed to a variable).
Expressions always evaluate to either an integer or a string. Refer to the following
example.
x + 5
// this evaluates to an integer
chr(i) + myString
// evaluates to a string
a = 3
// evaluates to 1 if true, 0 if false
b < c
// evaluates to 1 if true, 0 if false
The last two expressions are comparisons. Comparison operations always result in a
true or false value. In SIMPL+, true expressions result in a value of 1 and false
expressions result in a value of 0. Understanding this concept is key to performing
decision making in SIMPL+. In reality, any expression that evaluates to a non-zero
value is considered TRUE. This concept is discussed in “Controlling Program Flow:
Programming Guide – DOC. 5789A
SIMPL+
•
23