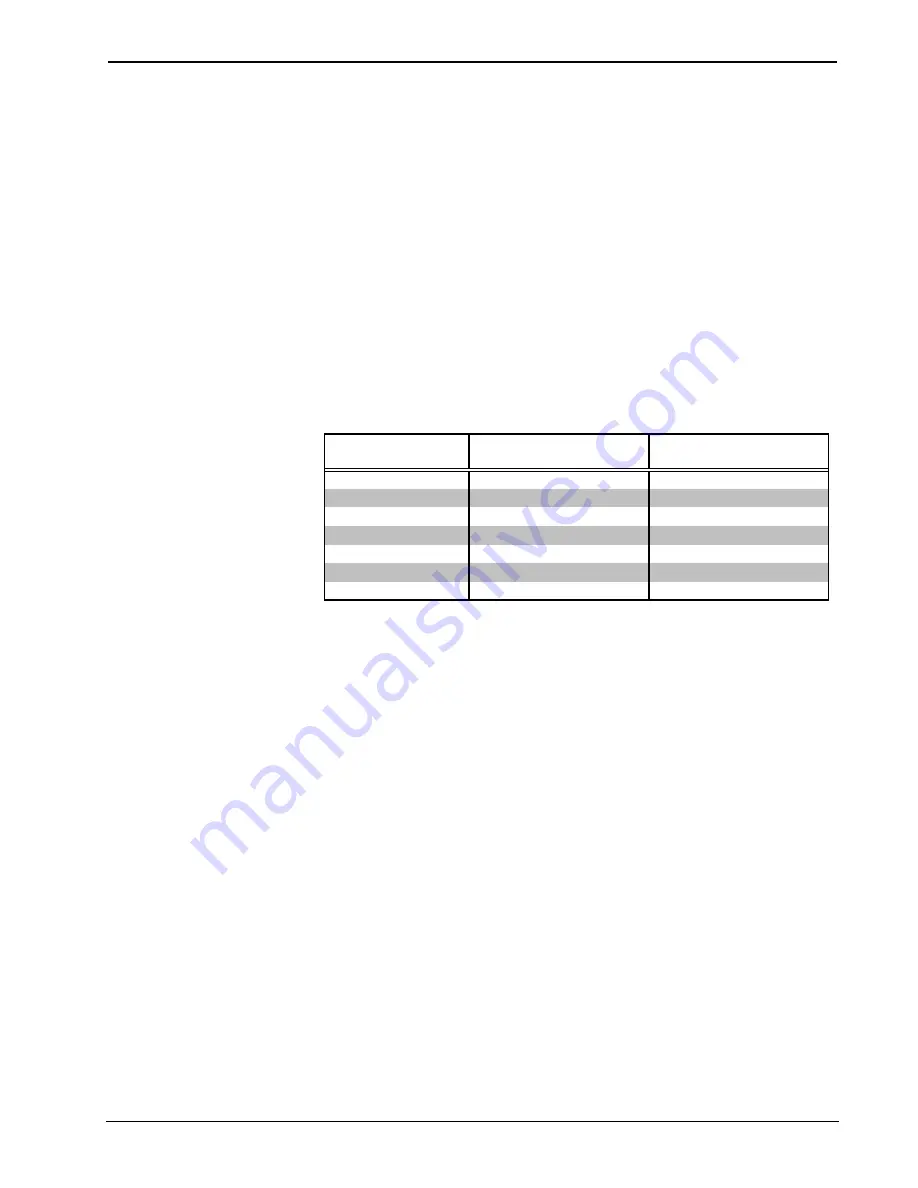
Crestron
SIMPL+
Software
When an integer has a value of between 0 and 32767, it is identical whether it is
considered signed or unsigned. However, numbers above 32767 may be treated as
negative numbers. If they are, they will have a value of x – 65536, where x is the
unsigned value of the number. This means that the value 65,535 has a signed value
of –1, 65534 is –2, etc. This scheme is referred to as two’s complement notation.
Why is all this signed/unsigned nonsense important? Well, in most cases, it can be
ignored and things work out fine. However, for those instances when it does make a
difference, it pays to understand how to debug programs that are not working as
expected.
In control system programming, often there is not a need for negative numbers (e.g.,
how often is a switcher switched to input number –12 ?). As a result, the most
common operations treat integers as unsigned and it becomes necessary to use
special “signed” operators or functions when treating numbers as signed. The table
after this paragraph lists operators and functions that are unsigned and those that are
signed. Any operators or functions that are not shown here do not need special
consideration.
Unsigned/Signed Operators and Functions
DESCRIPTION
UNSIGNED
OPERATORS/FUNCTIONS
SIGNED
OPERATORS/FUNCTIONS
Less than
<
S<
Less than or equal to
<=
S<=
Greater than
>
S>
Greater than or equal to
>=
S>=
Integer division
/
S/
Maximum
Max()
SMax()
Minimum
Min()
SMin()
Examine the following:
INTEGER j, k;
Function Main()
{
j = 2;
k = -1; // this is the same as k = 65535
if (j > k) // this will evaluate to FALSE
Print( “j is bigger as unsigned numbers\n” );
if (j S> k) // this will evaluate to TRUE
Print( “j is bigger as signed numbers\n” );
}
In this example, the first condition,
j > k
, evaluates to FALSE and the
statement does not execute. This is because the > operator performs an “unsigned
greater than” operation. If both
j
and
k
are converted to unsigned values,
j
remains at
2, but
k
becomes 65,535, and thus
k
is obviously not smaller than
j
.
The second condition,
j S> k
, evaluates to TRUE, because this time the “signed
greater than” operator was used.
Programming Guide – DOC. 5789A
SIMPL+
•
17