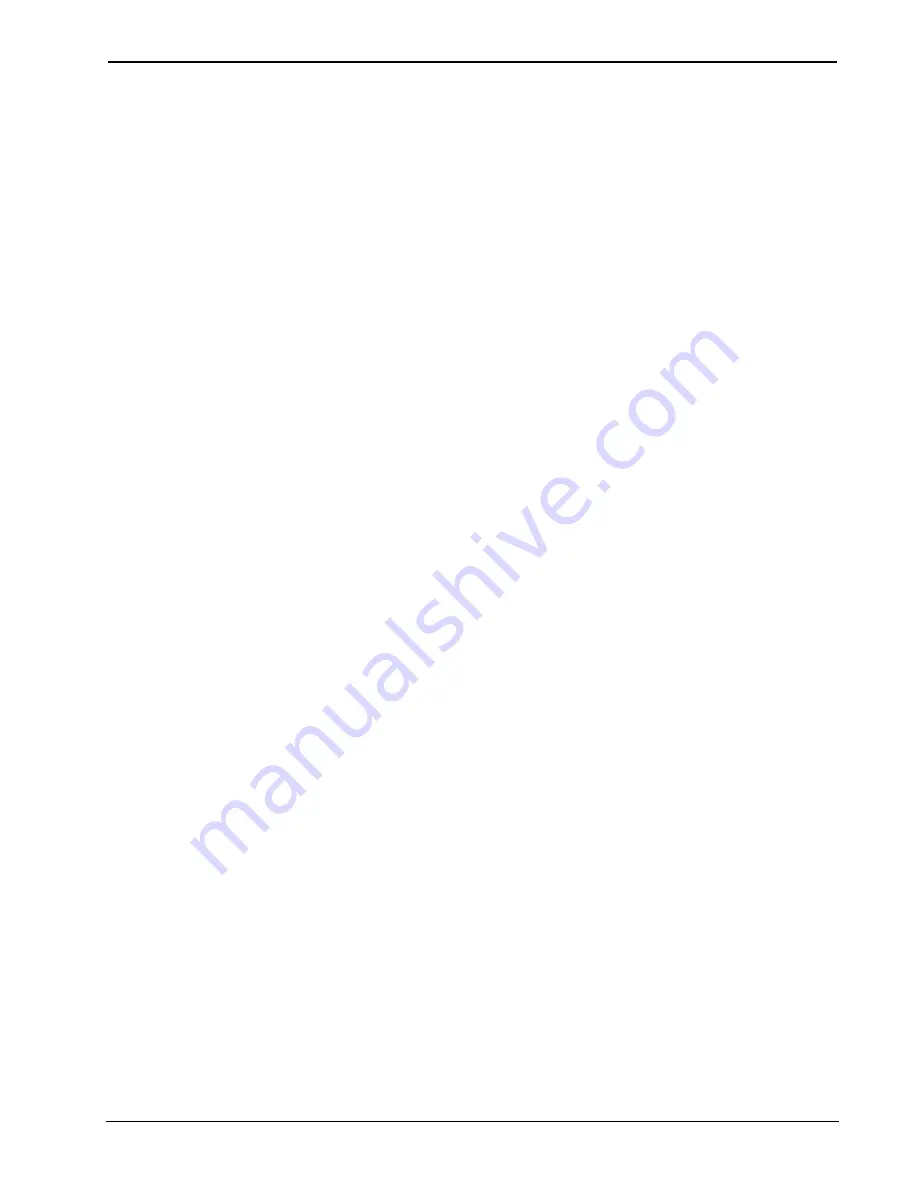
Software
Crestron
SIMPL+
To see an example of the
for
loop use the situation alluded to above. That is, a need
to clear each string element in a string array. A program to accomplish this might
look like the following.
DIGITAL_INPUT clearArray;
// a trigger signal
INTEGER
i;
//
the
index
variable
STRING stringArray[50][14];
// a 15-element array
PUSH
clearArray
//
event
function
{
for (i = 0 to 14)
{
stringArray[i] = "";
// set the ith element
//
to
an
empty
string
print("cleared element %d\n",i); // debug message
}
// this ends the for
//
loop
}
// this ends the push
//
function
In this example, the loop index
i
is set to run from 0 to 14, which represents the first
and last elements in
stringArray
respectively. Also notice that the step keyword is
omitted. This keyword is optional and if it is not used, the loop index increments by
1 each time through the loop. To clear only the even-numbered array elements, the
following could have used.
for (i = 0 to 14 step 2)
{ . . . }
The step value can also be negative, allowing the loop index to be reduced by some
value each time though the loop.
The
for
loop flexibility can be enhanced further by using expressions instead of
constant values for the start, end, and step values. For example, there might be a need
to add up the value of each byte in a string in order to calculate the value of a
checksum character. Since the length of the string can change as the program runs,
the number of iterations through the loop is unknown. The following code uses the
built-in function,
len
, to determine the length of the string and only run through the
for
loop the necessary number of times. System functions are described in detail in
“Using System Functions” on page 30.
checksum = 0; // initialize the chucksum variable
/* iterate through the string and add up the bytes.
Note that the { } braces are not needed here
because the contents of the for-loop is only
a single line of code */
for (i = 1 to len(someString))
checksum = ch byte(someString,i);
/* now add the checksum byte on to the string
using the chr function. Note that in this
example we only use the low-order byte from
the checksum variable */
someString = some chr(checksum);
28
•
SIMPL+
Programming Guide – DOC. 5789A