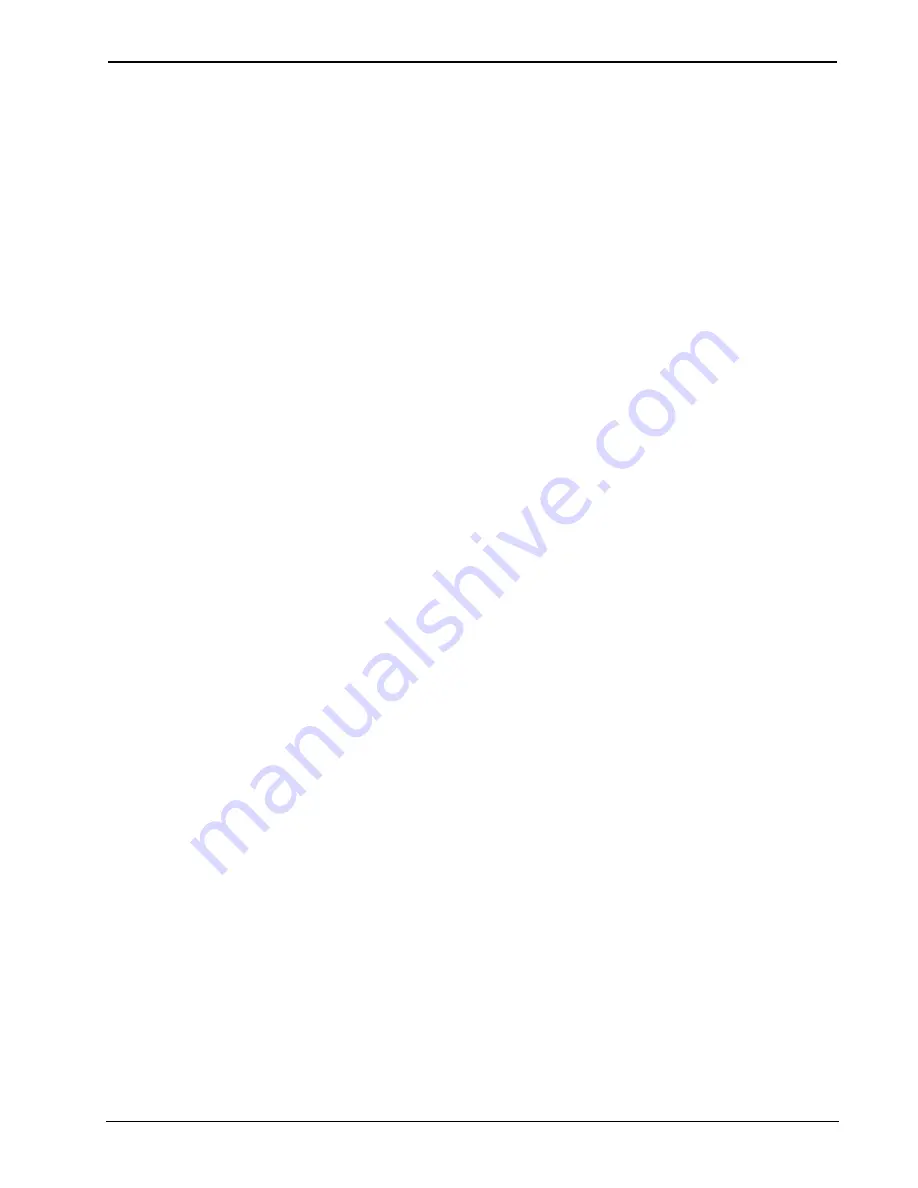
Software
Crestron
SIMPL+
Notice how the constant parts of the string are entered directly. The interesting thing
about the format specification is the %d sequences, which are known as “type
specifiers”.
Variable Scope
Variable declarations in a SIMPL+ program can be either global or local. Global
variables are defined in the "Define Variables" section of the code, and "exist"
throughout the entire SIMPL+ program. This means that any event function or user-
defined function can reference and modify global variables. When the value of a
global variable is being set or modified, it is reflected throughout the entire program.
Local variables are defined inside a function declaration and exist only inside that
particular function. In other words, if a local variable,
byteCount
, were defined inside
of a function,
CalcChecksum
, any reference to
byteCount
, outside of the scope of this
function (e.g. in another function) will result in a compiler syntax error. Note that
different functions can use the same variable names when defining local variables.
Take a look at the following example:
// simple function to add up all the bytes in a
// string and append the sum as a single byte
// onto the original string.
String_Function CalcChecksum(STRING argData)
{
INTEGER i, checksum;
checksum = 0;
for (i = 1 to len(argData))
checksum = ch byte(argData,i);
return (a chr(checksum));
}
In this example,
i
and
checksum
are local variables that only exist inside the function,
CalcChecksum
. This example also introduces an additional way to implement a local
variable: by passing it as an argument to the function, as was done with the STRING
variable,
argData
. The concept of local variables and argument passing is discussed
in detail in the section "User-Defined Functions" on page 31.
While the use of global variables may seem simpler, local variables can help keep
your programs better organized and easier to debug. A significant disadvantage of
global variables is that you must be careful each time you use or modify a variable
that it does not have an adverse effect on another part of the program. Since local
variables can only be used inside of a function, this is not a concern.
Arrays
When INTEGER or STRING variables are declared, the user may also declare them
as one- or two-dimensional (INTEGERs only) arrays. An array is a group of data of
the same type arranged in a table. A one-dimensional array can be thought of as a
single row with two or more columns, while a two-dimensional array can be thought
of as a table with multiple rows . In SIMPL+, arrays are declared as follows.
INTEGER myArray1[15] // 1-D integer array with 16 elements
INTEGER myArray2[10][3] // 2-D integer array with 11x4 elements
STRING myArray3[50][8] // 1-D string array with 9 elements
20
•
SIMPL+
Programming Guide – DOC. 5789A