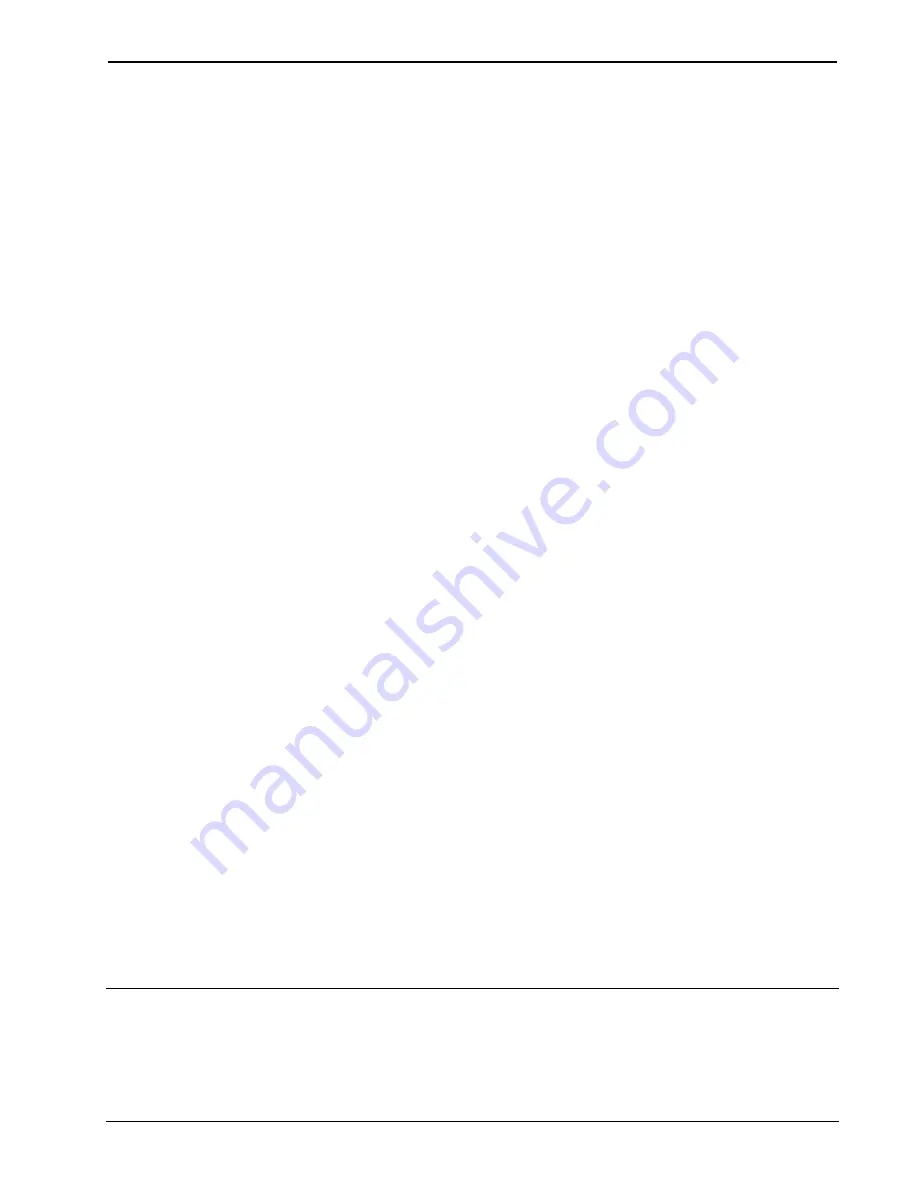
Software
Crestron
SIMPL+
this code, the value of this expression determines whether or not the code should be
executed again. Here lies the other difference between the
while
and
do-until
. The
while
loop executes as long as the expression remains TRUE. A
do-until
loop
executes until an expression becomes TRUE.
When deciding which type of loop should be used, first understand that using any of
the three types of loops discussed here can solve many problems. However, one
particular loop is usually better suited for a given application than the others. As a
general rule of thumb, when the number of iterations the code should execute is
known, a
for
loop is preferred. A
while
or a
do-until
loop is ideal to execute a section
of code continuously based on the value of some expression.
Once a
while
or a
do-until
loop is determined suitable for a particular application, the
question becomes which one of the two should be used? Once again realize that
either one can usually accomplish the goal, but one type of loop may require less
coding or be more readable in some cases. The basic question to ask is whether or
not the loop needs to run through at least one time. If so, a
do-until
is the best choice.
If instead, the value of an expression should be checked, then use the
while
loop.
Exiting from Loops Early
All three loops discussed above have built-in ways to exit. The
for
loop exits when
the index variable reaches the stated maximum. The
while
loop exits when the
expression becomes FALSE. The
do-until
loop exits when the expression becomes
TRUE.
Sometimes programming tasks do not always fall neatly into place regarding loops
and it may be desirable (or necessary) to exit a loop prematurely. Consider the
following example.
INTEGER x,y;
for (x=3 to z)
{
y = y + x*3 – z*z;
if (y = 0)
break;
}
Notice that in most (if not all) cases, the need for the break statement could be
avoided by the use of a different type of loop. In the example above, this could be
accomplished by using a
do-until
loop. Consider the following.
x = 3;
do
{
y = y + x*3 – z*z;
x = x + 1;
} until ((y = 0) || (x = z))
Either technique would be considered acceptable.
Using System Functions
In order to make programming in SIMPL+ simpler and more powerful, the concept
of functions is introduced. A function is essentially a more complicated
30
•
SIMPL+
Programming Guide – DOC. 5789A