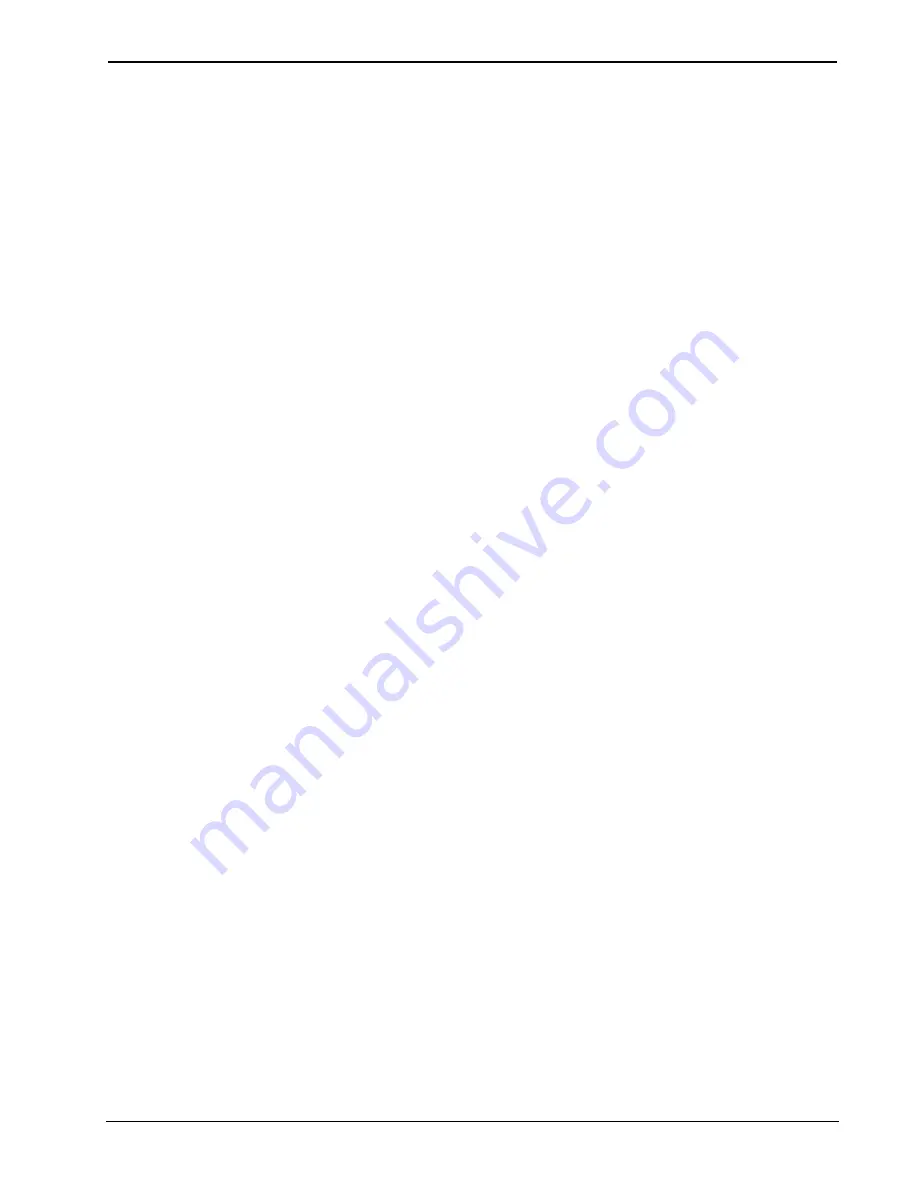
Crestron
SIMPL+
Software
name, as was done when it was declared. When assigning a value to a string, that
value is always assigned starting at the first character position.
It is important to note that the length of the this value does not exceed total length
allocated for
myString
(in this case, 50 characters). If the declared variable is not
large enough to hold the data being assigned to it, the data is truncated to as many
characters as the string can hold. Also, when the program is being executed, a string
overflow error will be reported within the control system’s error log.
The example above is useful, but does not really begin to tap the enormous string-
generating capabilities of SIMPL+. A common task in control system programming
is the control of an audio/video matrix router via RS-232. To control such a router,
often it is necessary to specify the input and output which make up the desired matrix
crosspoint. As an example, assume the device to be controlled expects to see a
command in a format, shown as follows:
IN<input#>OUT<output#><CR><LF>
This protocol allows the input and output to be specified as numbers. For example, to
switch input 4 to output 10, the command would be as follows:
IN4OUT10<CR><LF>
Where <CR><LF> represents the carriage return and line feed characters. Obviously,
for a router of any significant size, the number of possible crosspoints can grow very
large. Thus creating a literal string expression for each case would be very
inefficient. Instead, build the control string dynamically as the program runs. An
easy way to do this is through the use of the “string concatenation” operator (+).
Note that this is identical to the addition operator, but the SIMPL+ compiler is smart
enough to know whether integers are added or string expressions are concatenated.
This is addressed in the following code:
DIGITAL_INPUT do_switch;
STRING_OUTPUT switcher_out[10];
INTEGER input, output;
PUSH do_switch
{
switcher_out = "IN" + itoa(input) + "OUT" + itoa(output) +
"\n";
}
In this example, the + operator is used to concatenate multiple string expressions.
The
itoa
function has been used, which converts an integer value (in this case from
analog input signals) into a string representation of that number (e.g. 23 becomes
“23”).
There is an alternate way to build strings in SIMPL+. The
MakeString
function
provides functionality similar to the concatenation operator, while providing a bit
more power and flexibility. The following line is equivalent to the concatenation
statement above:
MakeString( switcher_out, "IN%dOUT%d\n", input, output );
This syntax is a bit more confusing. The first argument to the
MakeString
function,
switcher_out, is the destination string. This is where the resulting string created by
MakeString
is placed. The second argument, the part embedded in double-quotation
marks, is the “format specification”. This determines the general form of the data.
Programming Guide – DOC. 5789A
SIMPL+
•
19