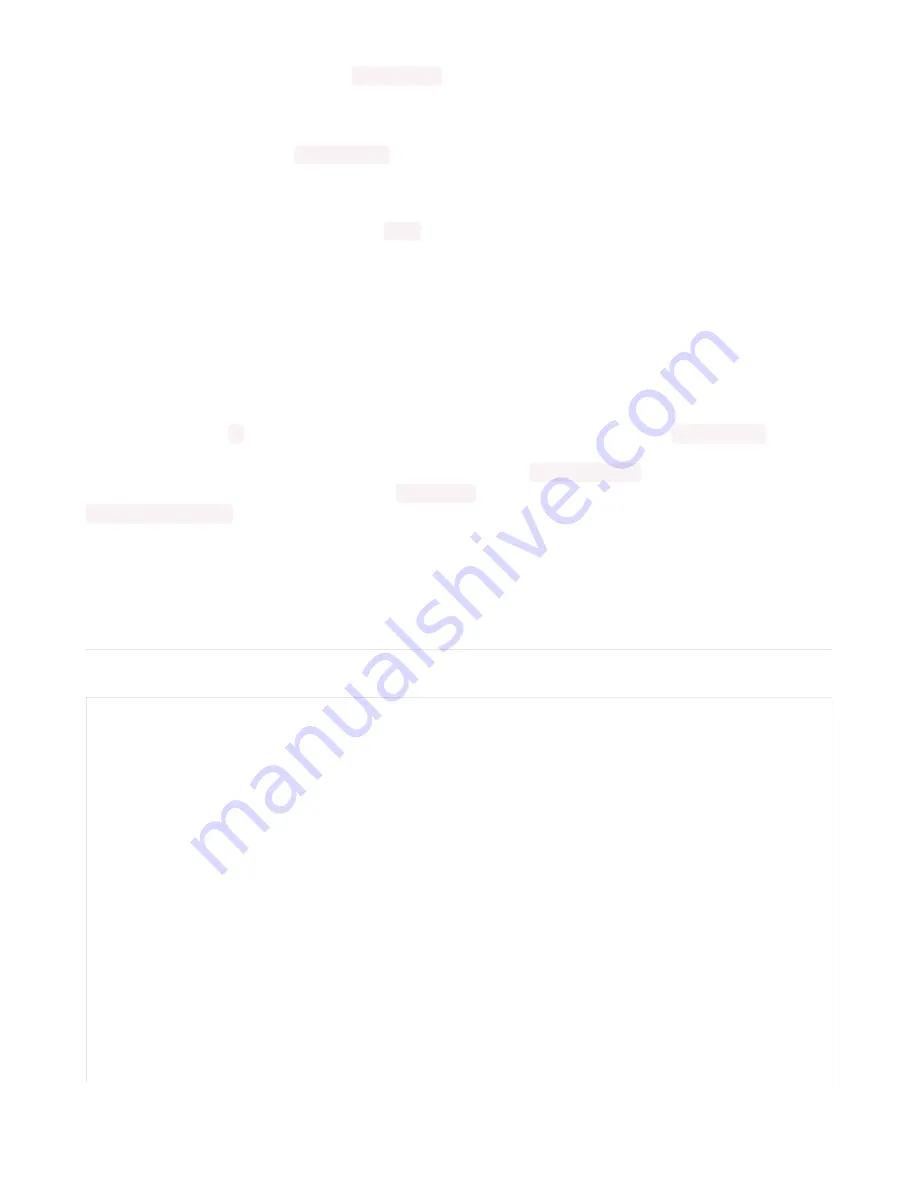
please submit a GitHub pull request!). The
time.sleep(1)
avoids an error that can happen if the program gets run as
soon as the board gets plugged in, before the host computer finishes connecting to the board.
Then we take the pins we chose above, and create the pin objects, set the direction and give them each a pullup.
Then we apply the pin objects to
key_pin_array
so we can use them later.
Next we set up the little red LED to so we can use it as a status light.
The last thing we do before we start our loop is
, "Waiting for key pin..." so you know the code is ready and
waiting!
The Main Loop
Inside the loop, we check each pin to see if the state has changed, i.e. you connected the pin to ground. Once it
changes, it prints, "Pin # grounded." to let you know the ground state has been detected. Then we turn on the red LED.
The code waits for the state to change again, i.e. it waits for you to unground the pin by disconnecting the wire
attached to the pin from ground.
Then the code gets the corresponding keys pressed from our array. If you grounded and ungrounded A1, the code
retrieves the keypress
a
, if you grounded and ungrounded A2, the code retrieves the string,
"Hello world!"
If the code finds that it's retrieved a string, it prints the string, using the
keyboard_layout
to determine the keypresses.
Otherwise, the code prints the keypress from the
control_key
and the keypress "a", which result in "A". Then it calls
keyboard.release_all()
. You always want to call this soon after a keypress or you'll end up with a stuck key which is
really annoying!
Instead of using a wire to ground the pins, you can try wiring up buttons like we did in
CircuitPython Digital In &
Out
(https://adafru.it/Beo)
. Try altering the code to add more pins for more keypress options!
CircuitPython Mouse Emulator
Copy and paste the code into code.py using your favorite editor, and save the file.
import time
import analogio
import board
import digitalio
from adafruit_hid.mouse import Mouse
mouse = Mouse()
x_axis = analogio.AnalogIn(board.A0)
y_axis = analogio.AnalogIn(board.A1)
select = digitalio.DigitalInOut(board.A2)
select.direction = digitalio.Direction.INPUT
select.pull = digitalio.Pull.UP
pot_min = 0.00
pot_max = 3.29
step = (pot_max - pot_min) / 20.0
def get_voltage(pin):
return (pin.value * 3.3) / 65536
© Adafruit Industries
https://learn.adafruit.com/adafruit-feather-m4-express-atsamd51
Page 162 of 183