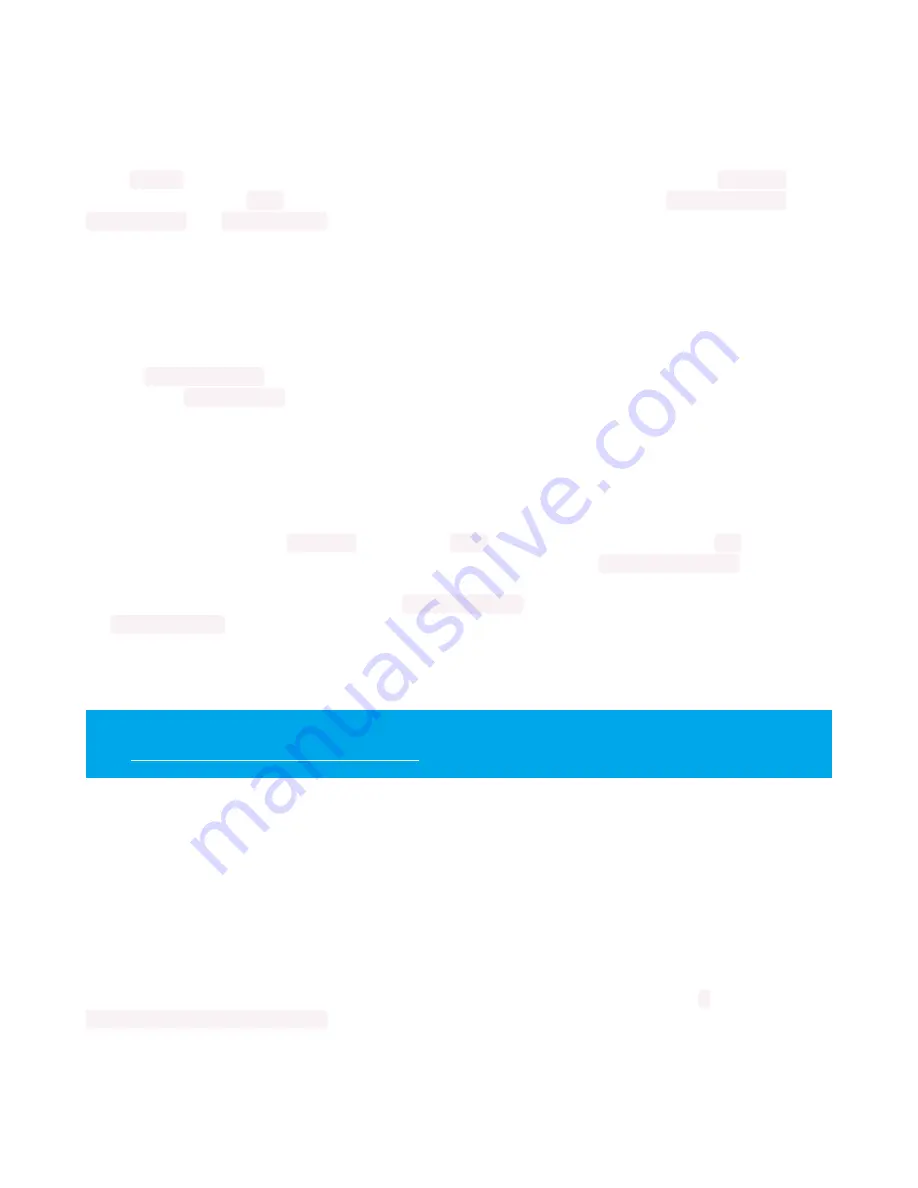
to your pixels. This makes your code more complicated, but it can make your LED animations faster!
DotStar Helpers
We've included a few helper functions to create the super fun visual effects found in this code.
First is
wheel()
which we just learned with the
Internal RGB LED
(https://adafru.it/Bel)
. Then we have
color_fill()
which
requires you to provide a
color
and the length of time you'd like it to be displayed. Next, are
slice_alternating()
,
slice_rainbow()
, and
rainbow_cycle()
which require you to provide the amount of time in seconds you'd between
each step of the animation.
Last, we've included a list of variables for our colors. This makes it much easier if to reuse the colors anywhere in the
code, as well as add more colors for use in multiple places. Assigning and using RGB colors is explained in
this section
of the CircuitPython Internal RGB LED page
(https://adafru.it/Bel)
.
The two slice helpers utilise a nifty feature of the DotStar library that allows us to use math to light up LEDs in repeating
patterns.
slice_alternating()
first lights up the even number LEDs and then the odd number LEDs and repeats this
back and forth.
slice_rainbow()
lights up every sixth LED with one of the six rainbow colors until the strip is filled. Both
use our handy color variables. This slice code only works when the total number of LEDs is divisible by the slice size, in
our case 2 and 6. DotStars come in strips of 30, 60, 72, and 144, all of which are divisible by 2 and 6. In the event that
you cut them into different sized strips, the code in this example may not work without modification. However, as long
as you provide a total number of LEDs that is divisible by the slices, the code will work.
Main Loop
Our main loop begins by calling
color_fill()
once for each
color
on our list and sets each to hold for
0.5
seconds. You
can change this number to change how fast each color is displayed. Next, we call
slice_alternating(0.1)
, which means
there's a 0.1 second delay between each change in the animation. Then, we fill the strip white to create a clean
backdrop for the rainbow to display. Then, we call
slice_rainbow(0.1)
, for a 0.1 second delay in the animation. Last we
call
rainbow_cycle(0)
, which means it's as fast as it can possibly be. Increase or decrease either of these numbers to
speed up or slow down the animations!
Note that the longer your strip of LEDs is, the longer it will take for the animations to complete.
Is it SPI?
We explained at the beginning of this section that the LEDs respond faster if you're using hardware SPI. On some of
the boards, there are HW SPI pins directly available in the form of MOSI and SCK. However, hardware SPI is available
on more than just those pins. But, how can you figure out which? Easy! We wrote a handy script.
We chose pins A1 and A2 for our example code. To see if these are hardware SPI on the board you're using, copy and
paste the code into code.py using your favorite editor, and save the file. Then connect to the serial console to see the
results.
To check if other pin combinations have hardware SPI, change the pin names on the line reading:
if
is_hardware_SPI(board.A1, board.A2):
to the pins you want to use. Then, check the results in the serial console. Super
simple!
We have a ton more information on general purpose DotStar know-how at our DotStar UberGuide
https://learn.adafruit.com/adafruit-dotstar-leds
© Adafruit Industries
https://learn.adafruit.com/adafruit-feather-m4-express-atsamd51
Page 143 of 183