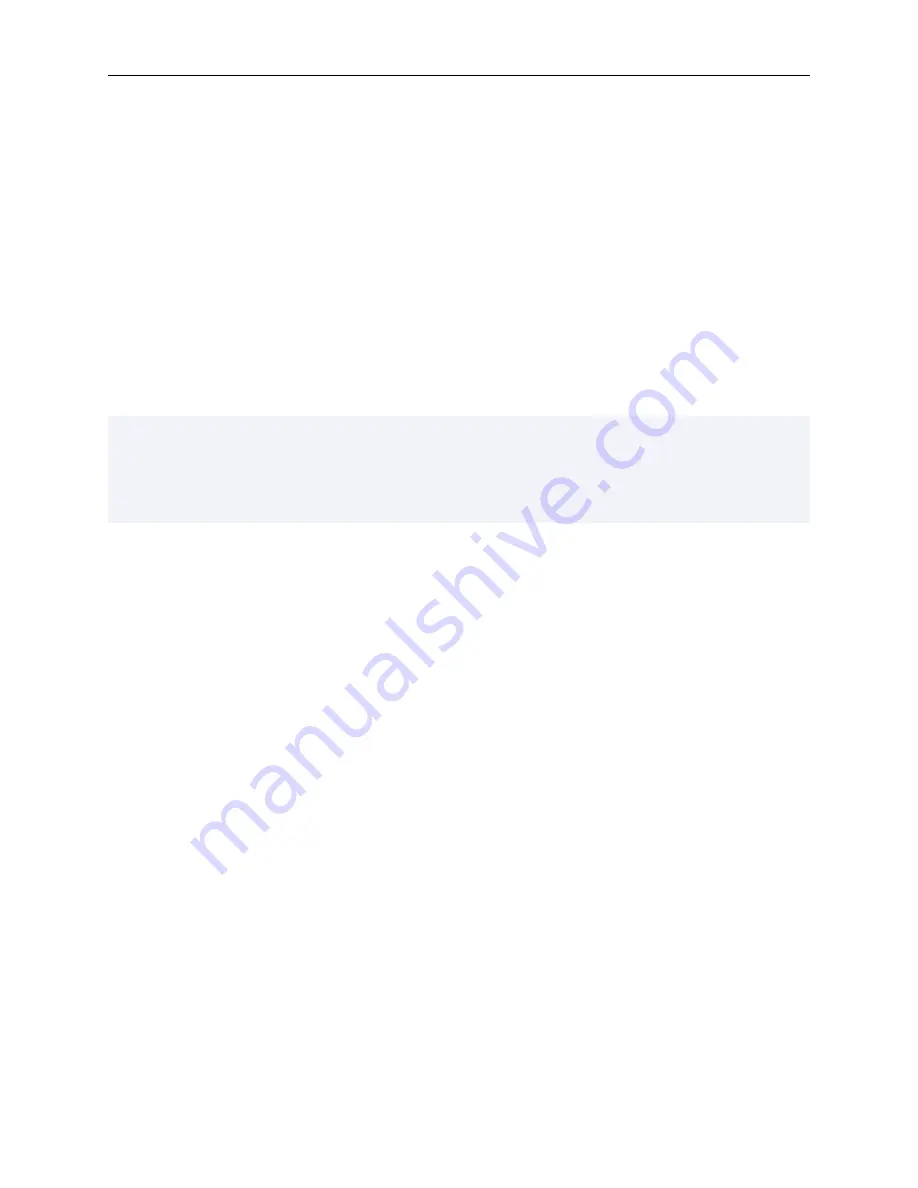
Memcheck: a memory error detector
The worst thing is that on Linux apparently it doesn’t matter if you do mix these up, but the same program may then
crash on a different platform, Solaris for example. So it’s best to fix it properly. According to the KDE folks "it’s
amazing how many C++ programmers don’t know this".
The reason behind the requirement is as follows. In some C++ implementations,
delete[]
must be used for objects
allocated by
new[]
because the compiler stores the size of the array and the pointer-to-member to the destructor of
the array’s content just before the pointer actually returned.
delete
doesn’t account for this and will get confused,
possibly corrupting the heap.
4.2.6. Overlapping source and destination blocks
The following C library functions copy some data from one memory block to another (or something similar):
memcpy
,
strcpy
,
strncpy
,
strcat
,
strncat
. The blocks pointed to by their
src
and
dst
pointers aren’t allowed to
overlap. The POSIX standards have wording along the lines "If copying takes place between objects that overlap, the
behavior is undefined." Therefore, Memcheck checks for this.
For example:
==27492== Source and destination overlap in memcpy(0xbffff294, 0xbffff280, 21)
==27492==
at 0x40026CDC: memcpy (mc_replace_strmem.c:71)
==27492==
by 0x804865A: main (overlap.c:40)
You don’t want the two blocks to overlap because one of them could get partially overwritten by the copying.
You might think that Memcheck is being overly pedantic reporting this in the case where
dst
is less than
src
.
For example, the obvious way to implement
memcpy
is by copying from the first byte to the last.
However, the
optimisation guides of some architectures recommend copying from the last byte down to the first. Also, some
implementations of
memcpy
zero
dst
before copying, because zeroing the destination’s cache line(s) can improve
performance.
The moral of the story is: if you want to write truly portable code, don’t make any assumptions about the language
implementation.
4.2.7. Memory leak detection
Memcheck keeps track of all heap blocks issued in response to calls to
malloc
/
new
et al. So when the program exits,
it knows which blocks have not been freed.
If
--leak-check
is set appropriately, for each remaining block, Memcheck determines if the block is reachable
from pointers within the root-set.
The root-set consists of (a) general purpose registers of all threads, and (b)
initialised, aligned, pointer-sized data words in accessible client memory, including stacks.
There are two ways a block can be reached. The first is with a "start-pointer", i.e. a pointer to the start of the block.
The second is with an "interior-pointer", i.e. a pointer to the middle of the block. There are three ways we know of
that an interior-pointer can occur:
• The pointer might have originally been a start-pointer and have been moved along deliberately (or not deliberately)
by the program. In particular, this can happen if your program uses tagged pointers, i.e. if it uses the bottom one,
two or three bits of a pointer, which are normally always zero due to alignment, in order to store extra information.
• It might be a random junk value in memory, entirely unrelated, just a coincidence.
54
Содержание BBV
Страница 176: ...Valgrind FAQ Release 3 8 0 10 August 2012 Copyright 2000 2012 Valgrind Developers Email valgrind valgrind org ...
Страница 177: ...Valgrind FAQ Table of Contents Valgrind Frequently Asked Questions 1 ii ...
Страница 302: ...README mips based on newer GCC versions if possible 95 ...
Страница 303: ...GNU Licenses ...
Страница 304: ...GNU Licenses Table of Contents 1 The GNU General Public License 1 2 The GNU Free Documentation License 8 ii ...