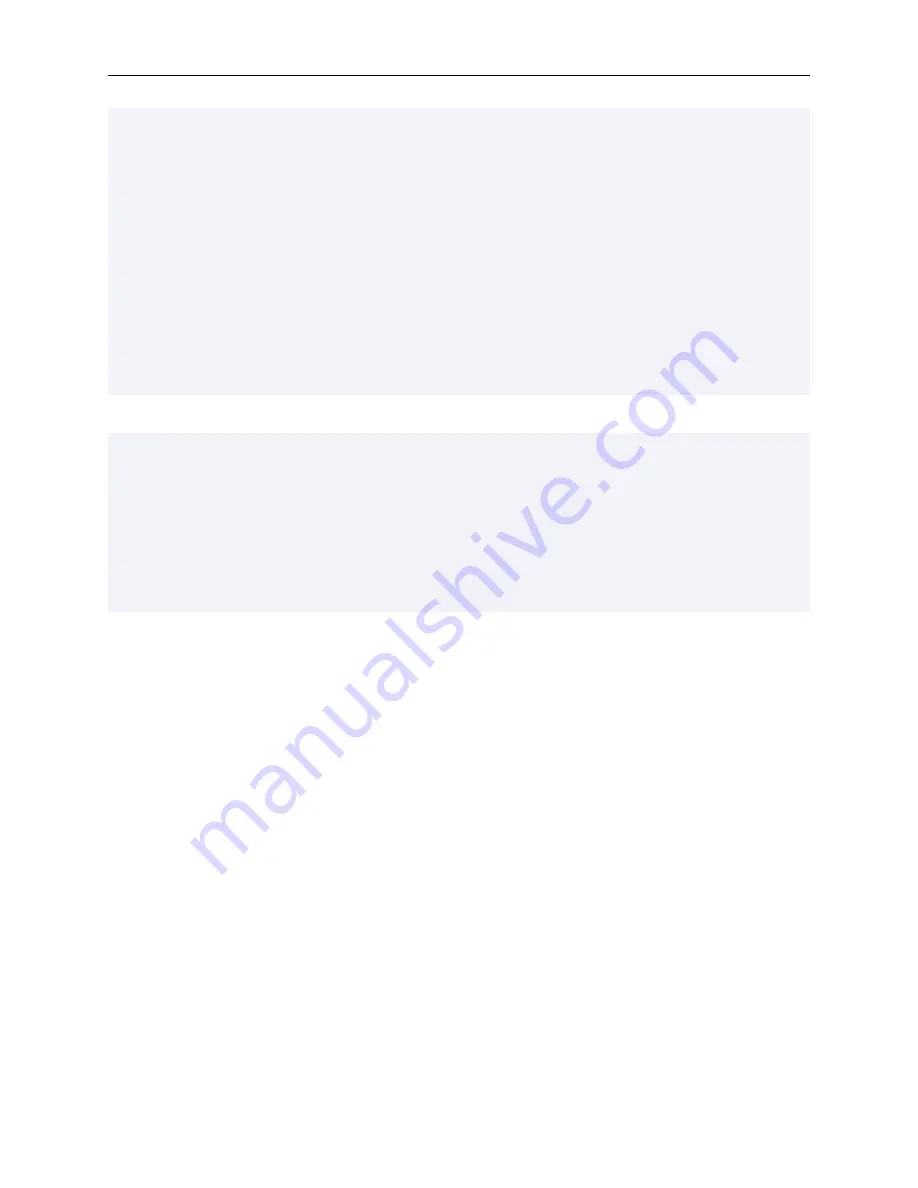
The Valgrind Quick Start Guide
#include <stdlib.h>
void f(void)
{
int* x = malloc(10 * sizeof(int));
x[10] = 0;
// problem 1: heap block overrun
}
// problem 2: memory leak -- x not freed
int main(void)
{
f();
return 0;
}
Most error messages look like the following, which describes problem 1, the heap block overrun:
==19182== Invalid write of size 4
==19182==
at 0x804838F: f (example.c:6)
==19182==
by 0x80483AB: main (example.c:11)
==19182==
Address 0x1BA45050 is 0 bytes after a block of size 40 alloc’d
==19182==
at 0x1B8FF5CD: malloc (vg_replace_malloc.c:130)
==19182==
by 0x8048385: f (example.c:5)
==19182==
by 0x80483AB: main (example.c:11)
Things to notice:
• There is a lot of information in each error message; read it carefully.
• The 19182 is the process ID; it’s usually unimportant.
• The first line ("Invalid write...") tells you what kind of error it is.
Here, the program wrote to some memory it
should not have due to a heap block overrun.
• Below the first line is a stack trace telling you where the problem occurred. Stack traces can get quite large, and be
confusing, especially if you are using the C++ STL. Reading them from the bottom up can help. If the stack trace
is not big enough, use the
--num-callers
option to make it bigger.
• The code addresses (eg. 0x804838F) are usually unimportant, but occasionally crucial for tracking down weirder
bugs.
• Some error messages have a second component which describes the memory address involved.
This one shows
that the written memory is just past the end of a block allocated with malloc() on line 5 of example.c.
2
Содержание BBV
Страница 176: ...Valgrind FAQ Release 3 8 0 10 August 2012 Copyright 2000 2012 Valgrind Developers Email valgrind valgrind org ...
Страница 177: ...Valgrind FAQ Table of Contents Valgrind Frequently Asked Questions 1 ii ...
Страница 302: ...README mips based on newer GCC versions if possible 95 ...
Страница 303: ...GNU Licenses ...
Страница 304: ...GNU Licenses Table of Contents 1 The GNU General Public License 1 2 The GNU Free Documentation License 8 ii ...