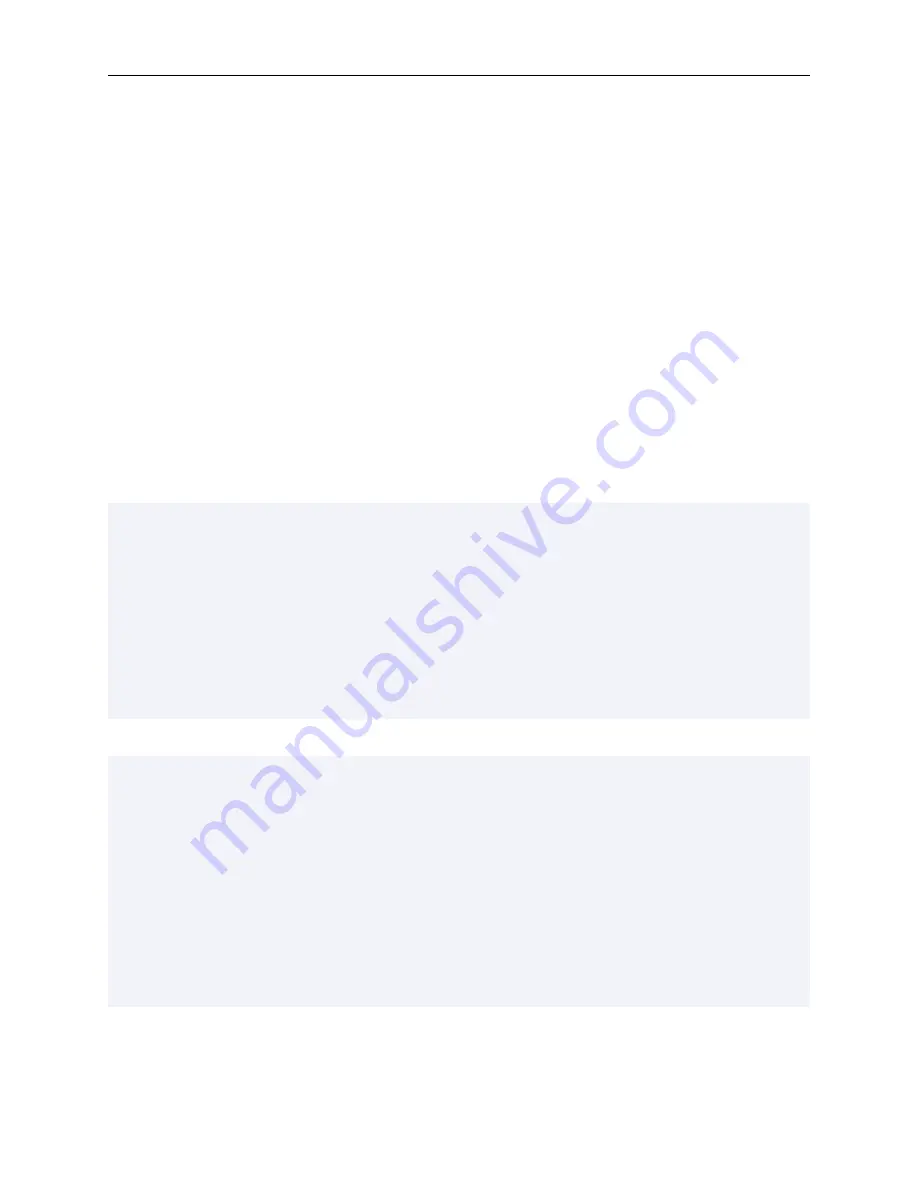
Memcheck: a memory error detector
To see information on the sources of uninitialised data in your program, use the
--track-origins=yes
option.
This makes Memcheck run more slowly, but can make it much easier to track down the root causes of uninitialised
value errors.
4.2.3. Use of uninitialised or unaddressable values in
system calls
Memcheck checks all parameters to system calls:
• It checks all the direct parameters themselves, whether they are initialised.
• Also, if a system call needs to read from a buffer provided by your program, Memcheck checks that the entire buffer
is addressable and its contents are initialised.
• Also, if the system call needs to write to a user-supplied buffer, Memcheck checks that the buffer is addressable.
After the system call, Memcheck updates its tracked information to precisely reflect any changes in memory state
caused by the system call.
Here’s an example of two system calls with invalid parameters:
#include <stdlib.h>
#include <unistd.h>
int main( void )
{
char* arr = malloc(10);
int* arr2 = malloc(sizeof(int));
write( 1 /* stdout */, arr, 10 );
exit(arr2[0]);
}
You get these complaints ...
Syscall param write(buf) points to uninitialised byte(s)
at 0x25A48723: __write_nocancel (in /lib/tls/libc-2.3.3.so)
by 0x259AFAD3: __libc_start_main (in /lib/tls/libc-2.3.3.so)
by 0x8048348: (within /auto/homes/njn25/grind/head4/a.out)
Address 0x25AB8028 is 0 bytes inside a block of size 10 alloc’d
at 0x259852B0: malloc (vg_replace_malloc.c:130)
by 0x80483F1: main (a.c:5)
Syscall param exit(error_code) contains uninitialised byte(s)
at 0x25A21B44: __GI__exit (in /lib/tls/libc-2.3.3.so)
by 0x8048426: main (a.c:8)
... because the program has (a) written uninitialised junk from the heap block to the standard output, and (b) passed an
uninitialised value to
exit
. Note that the first error refers to the memory pointed to by
buf
(not
buf
itself), but the
second error refers directly to
exit
’s argument
arr2[0]
.
52
Содержание BBV
Страница 176: ...Valgrind FAQ Release 3 8 0 10 August 2012 Copyright 2000 2012 Valgrind Developers Email valgrind valgrind org ...
Страница 177: ...Valgrind FAQ Table of Contents Valgrind Frequently Asked Questions 1 ii ...
Страница 302: ...README mips based on newer GCC versions if possible 95 ...
Страница 303: ...GNU Licenses ...
Страница 304: ...GNU Licenses Table of Contents 1 The GNU General Public License 1 2 The GNU Free Documentation License 8 ii ...