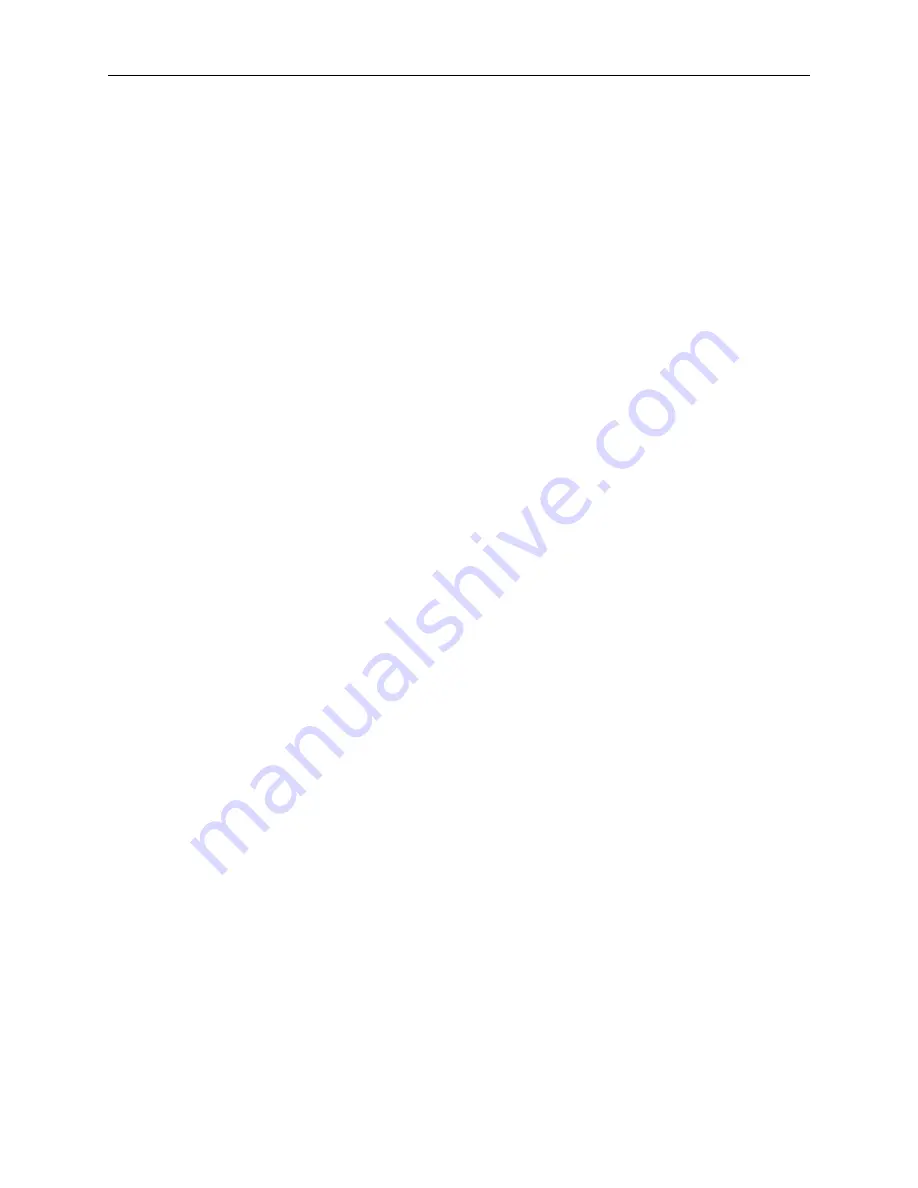
DRD: a thread error detector
In complex applications it is not always clear from beforehand which mutex will be locked recursively and which
mutex will not be locked recursively. Attempts lock a non-recursive mutex recursively will result in race conditions
that are very hard to find without a thread checking tool. So either use the error checking mutex type and consistently
check the return value of Pthread API mutex calls, or use the recursive mutex type.
8.3.2. Condition variables
A condition variable allows one thread to wake up one or more other threads. Condition variables are often used to
notify one or more threads about state changes of shared data. Unfortunately it is very easy to introduce race conditions
by using condition variables as the only means of state information propagation. A better approach is to let threads
poll for changes of a state variable that is protected by a mutex, and to use condition variables only as a thread wakeup
mechanism. See also the source file
drd/tests/monitor_example.cpp
for an example of how to implement
this concept in C++. The monitor concept used in this example is a well known and very useful concept -- see also
Wikipedia for more information about the monitor concept.
8.3.3. pthread_cond_timedwait and timeouts
Historically the function
pthread_cond_timedwait
only allowed the specification of an absolute timeout, that is
a timeout independent of the time when this function was called. However, almost every call to this function expresses
a relative timeout. This typically happens by passing the sum of
clock_gettime(CLOCK_REALTIME)
and a
relative timeout as the third argument. This approach is incorrect since forward or backward clock adjustments by e.g.
ntpd will affect the timeout. A more reliable approach is as follows:
• When initializing a condition variable through
pthread_cond_init
,
specify that the timeout of
pthread_cond_timedwait
will use the clock
CLOCK_MONOTONIC
instead of
CLOCK_REALTIME
.
You can do this via
pthread_condattr_setclock(..., CLOCK_MONOTONIC)
.
• When calling
pthread_cond_timedwait
, pass the sum of
clock_gettime(CLOCK_MONOTONIC)
and a
relative timeout as the third argument.
See also
drd/tests/monitor_example.cpp
for an example.
8.4. Limitations
DRD currently has the following limitations:
• DRD, just like Memcheck, will refuse to start on Linux distributions where all symbol information has been removed
from
ld.so
. This is e.g. the case for the PPC editions of openSUSE and Gentoo. You will have to install the glibc
debuginfo package on these platforms before you can use DRD. See also openSUSE bug 396197 and Gentoo bug
214065.
• With gcc 4.4.3 and before, DRD may report data races on the C++ class
std::string
in a multithreaded program.
This is a know
+
issue -- see also GCC bug 40518 for more information.
• If you compile the DRD source code yourself, you need GCC 3.0 or later. GCC 2.95 is not supported.
• Of the two POSIX threads implementations for Linux, only the NPTL (Native POSIX Thread Library) is supported.
The older LinuxThreads library is not supported.
8.5. Feedback
If you have any comments, suggestions, feedback or bug reports about DRD, feel free to either post a message on the
Valgrind users mailing list or to file a bug report. See also http://www.valgrind.org/ for more information.
135
Содержание BBV
Страница 176: ...Valgrind FAQ Release 3 8 0 10 August 2012 Copyright 2000 2012 Valgrind Developers Email valgrind valgrind org ...
Страница 177: ...Valgrind FAQ Table of Contents Valgrind Frequently Asked Questions 1 ii ...
Страница 302: ...README mips based on newer GCC versions if possible 95 ...
Страница 303: ...GNU Licenses ...
Страница 304: ...GNU Licenses Table of Contents 1 The GNU General Public License 1 2 The GNU Free Documentation License 8 ii ...