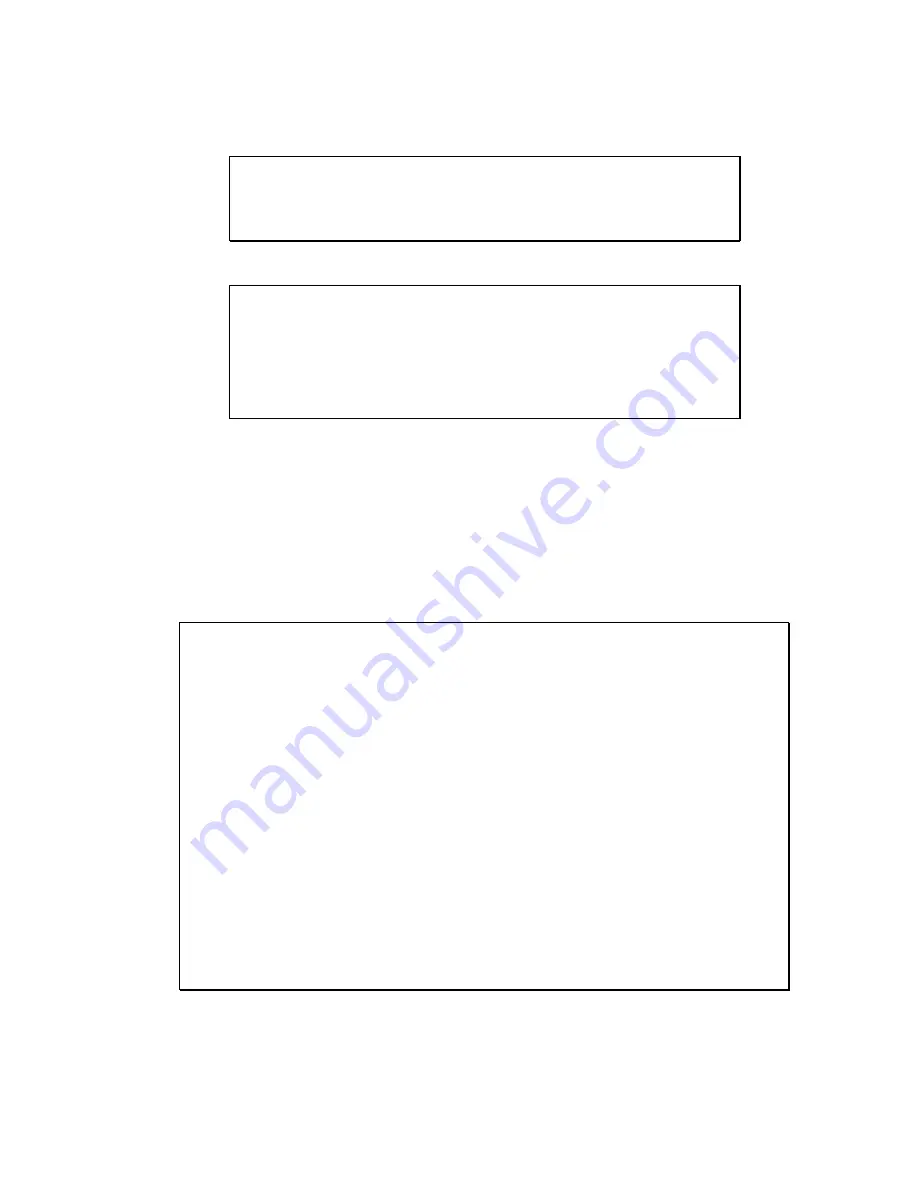
4-6
/* assume pm2 has been correctly maintained. The following code will modify the pm2
register such that bits 0 through 3 are low. Use AND to set bits low */
pokeb(0xfff0, 0x11, ( pm2 = ( pm2 & 0xF0 ) ) ); //pm2 must be set to a new register
value
Example 4.6
Set port 2 pins 0 through 3 as input.
// The following global variable defines the pm2 register
unsigned char pm2;
/* assume pm2 has been correctly maintained. The following code will modify the pm2
register such that bits 0 through 3 are high. Use OR to set bits high */
pokeb(0xfff0, 0x11, ( pm2 = ( pm2 | 0x0F ) ) ); //pm2 must be set to new register value
4.2.4
Port T
Port T is an 8-bit input port whose threshold voltage can be changed in 16 steps. Comparator operation is
performed through this port. Each Port T input is compared with the selected threshold voltage (Vth). PTn
> Vth results in a value 1, PTn < Vth results in a value 0. All eight results from PT0 to PT7 are latched to
the port T input latches.
The resulting 8-bit latch can be accessed by the function portt_rd(void) which returns the 8-bit result. Vth
can be changed by the function portt_wr(char vref). The variable vref {0 .. 15} sets the reference voltage
by the following equation: Reference = Vth * vref/16. vref = 0 sets Reference = Vth. Vth is connected to
a 10 K pullup resistor network and Vth
≈
3.57V. PT0 – PT6 are on J2. PT0 – PT2 are pulled up by 10k
resistors.
void portt_wr(char vref)
where vref is a number to select VREF
vref = 0 VTHx 1
vref = 1 VTHx 1/16
vref = 2 VTHx 2/16
vref = 3 VTHx 3/16
vref = 4 VTHx 4/16
vref = 5 VTHx 5/16
vref = 6 VTHx 6/16
vref = 7 VTHx 7/16
vref = 8 VTHx 8/16
vref = 9 VTHx 9/16
vref = 10 VTHx 10/16
vref = 11 VTHx 11/16
vref = 12 VTHx 12/16
vref = 13 VTHx 13/16
vref = 14 VTHx 14/16
vref = 15 VTHx 15/16
char portt_rd(void)
returns an 8-bit character representing the comparator output if the voltage at PT0 < Vref, bit 0=0 else 1.