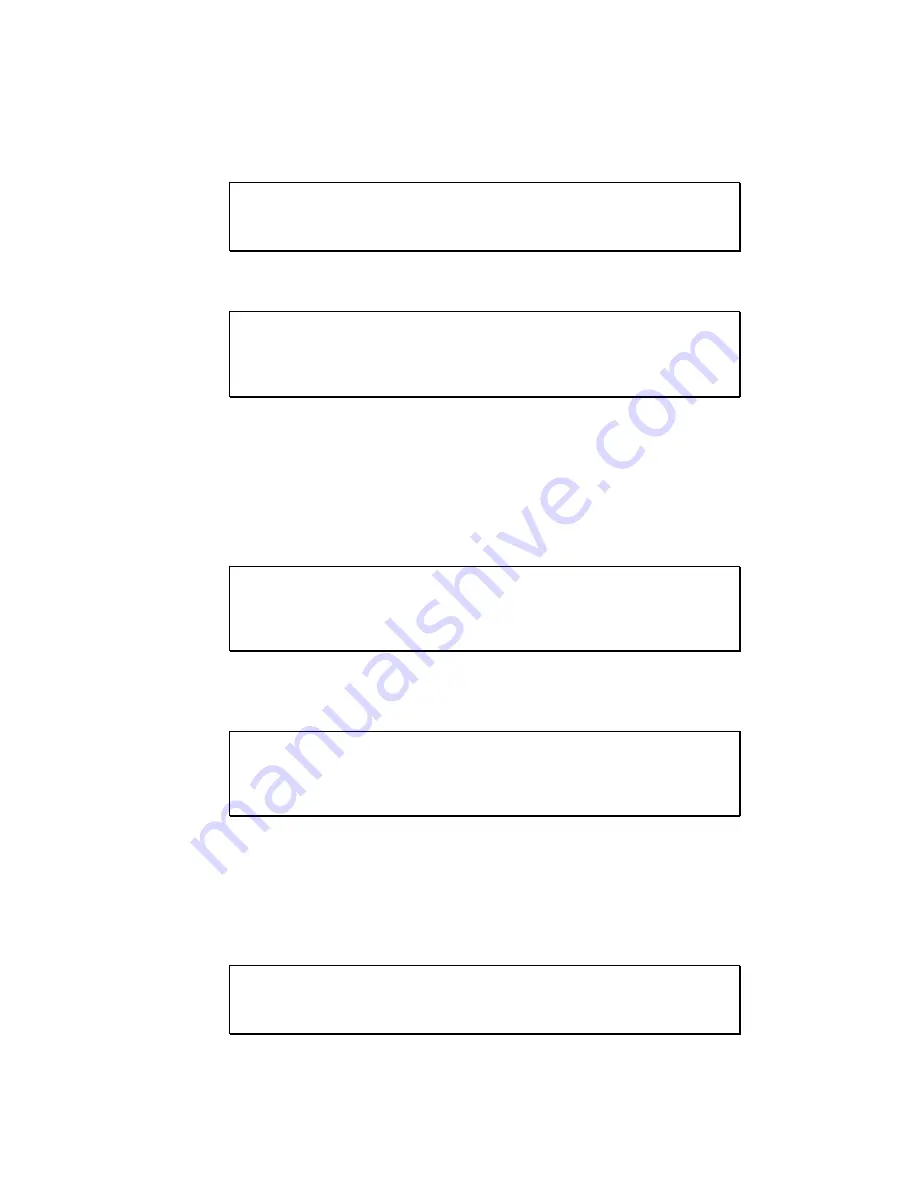
4-5
Example 4.1
Set port 0 as I/O, bits 0 – 3 as input, 4 – 7 as output.
port_init(0, 0x00, 0xf0);
p = Port 0,
pmc = 0 (I/O),
pm 0-3 = 1, pm 4-7 = 0.
Example 4.2
Set pins 20 and 23 as DMA Request. All other port 2 pins as output.
port_init(2, 0x09, 0x00);
p = Port 2,
pmc = bit 0 and 3 = 1 (Control), all others = 0 (I/O)
pm = all 0s. Since pins 20 and 23 are control functions, the pm field is not
relevant.
In most cases it is only necessary to change the value of one or two pins in the port data register Since the
port data register is a read/write register, it is possible to mask the pins that do not need to change. In this
case, the port_init function cannot be used. Instead, the port data register can be directly accessed using the
poke and peek functions.
Example 4.3
Using bitwise OR to set a single bit high, set pin 23 high without
modifying the other pins. Assume all port 2 is output and that all pins
are low.
pokeb(0xfff0, 0x10, (unsigned char) (peekb( 0xfff0, 0x10) | 0x08) );
Assuming that all of port 2 is outputting low, the peekb function will return a value of
0x00. A bitwise ‘OR’ with the value 0x00 and the mask 0x08 equals 0x08.
Port 2 now outputs 0x08.
Example 4.4
Using bitwise AND to reset a single bit low, set pin 23 low without
modifying the other pins. Assume settings are the same after executing
Example 4.3.
pokeb(0xfff0, 0x10, (unsigned char) (peekb( 0xfff0, 0x10) & 0xF7 ) );
Assuming the settings from Example 4.3 are still present, the peekb function should return
a value of 0x08. A bitwise ‘AND’ with the value 0x08 and the mask 0xF7
equals 0x00. Port is again set to 0x00 (all pins low).
While the port data registers are read/write registers, the port control registers pmc and pm are not.
Modifying only certain pins in these registers requires the use of global variables to store the values of these
registers. This means that any changes to the pmc or pm registers must be accounted for in the global
variable. As in the previous example, the bitwise OR and AND expressions can be used to mask the
register bits.
Example 4.5
Set port 2 pins 0 through 3 as output.
// The following global variable defines the pm2 register
unsigned char pm2;