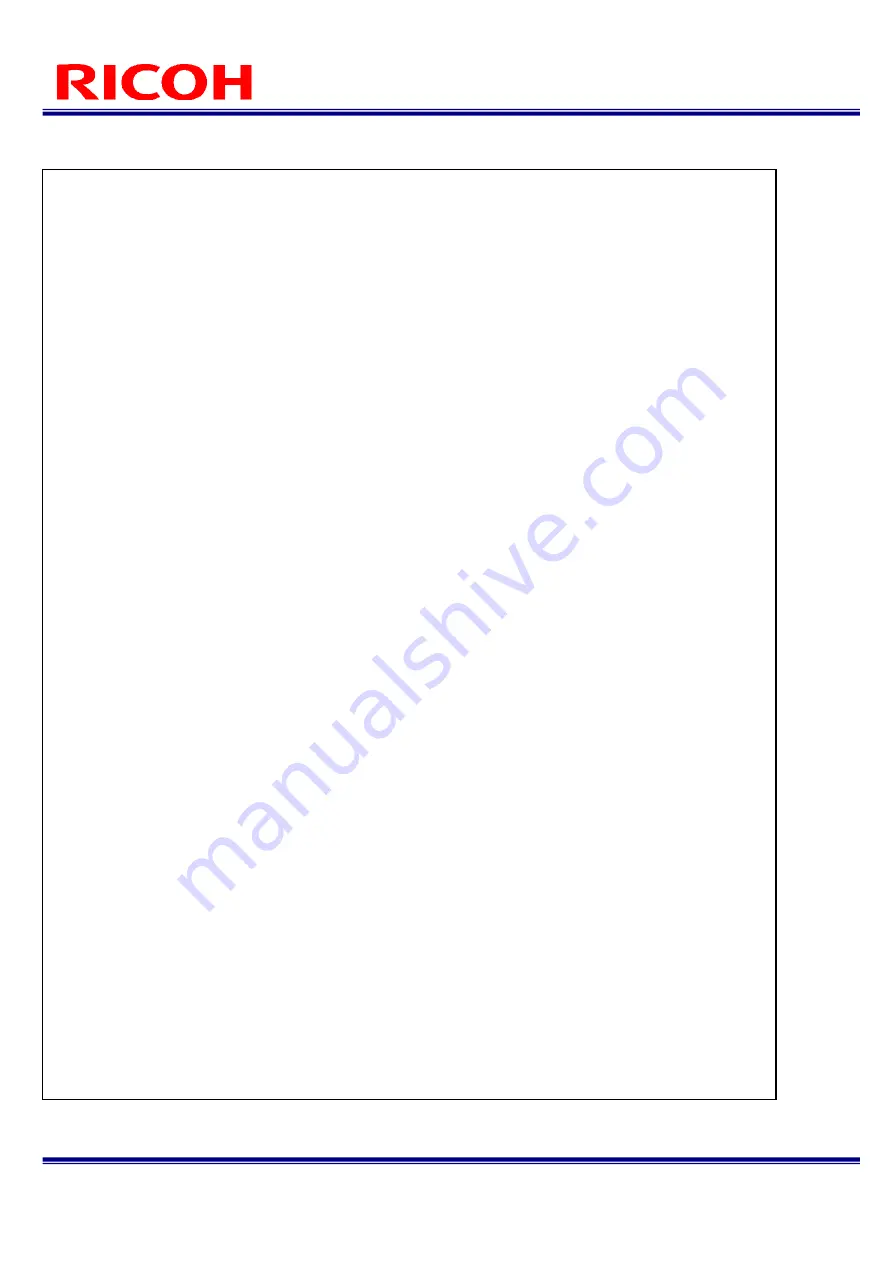
RICOH SC-10 SERIES
Socket Mode Function Operating Instructions Ver.1.0.0
62/66
Receive program
static
void
Main()
{
IPAddress
thisip =
IPAddress
.Parse(
"192.168.183.50"
);
int
port = 56109;
var
myserver =
new
Server
(thisip, port);
myserver.Run();
//Synchronous operation
}
public
class
Server
{
private
class
StateObject
{
public
Socket
ClientSocket {
get
;
set
; }
public
const
int
BufferSize = 1536;
public
byte
[] Buffer {
get
; } =
new
byte
[BufferSize];
}
// For waiting for thread
private
ManualResetEvent
AllDone =
new
ManualResetEvent
(
false
);
// End point of server
private
IPEndPoint
{
get
; }
// Client to which connected (thread safe collection)
private
SynchronizedCollection
<
Socket
> ClientSockets {
get
; } =
new
SynchronizedCollection
<
Socket
>();
public
Server(
IPAddress
myip,
int
port)
{
this
.IPEndPoint =
new
IPEndPoint
(myip, port);
}
// Start up server
public
void
Run()
{
using
(
var
listenerSocket =
new
Socket
(
AddressFamily
.InterNetwork,
SocketType
.Stream,
ProtocolType
.Tcp))
{
try
{
// Bind socket to address
listenerSocket.SetSocketOption(
SocketOptionLevel
.Socket,
SocketOptionName
.ReuseAddress,
true
);
listenerSocket.Bind(
this
.IPEndPoint);
// Connection wait start
listenerSocket.Listen(10);
Console
.WriteLine(
"Server started up.\ nIP address:Port number\n"
+
listenerSocket.LocalEndPoint);
// Connection wait loop
while
(
true
)
{
AllDone.Reset();
listenerSocket.BeginAccept(
new
AsyncCallback
(AcceptCallback), listenerSocket);
AllDone.WaitOne();
}
}
catch
(
Exception
e)
{
Console
.WriteLine(
"Communication port connection failed. Check the setting and then restart.\ n"
+
e);
return
;
}
}
}
// Callback process when connection accepted
private
void
AcceptCallback(
IAsyncResult
asyncResult)
{
// Set signal so that wait thread progresses
AllDone.Set();
// Acquire socket
var
listenerSocket = asyncResult.AsyncState
as
Socket
;
var
clientSocket = listenerSocket.EndAccept(asyncResult);
// Add client during connection
ClientSockets.Add(clientSocket);