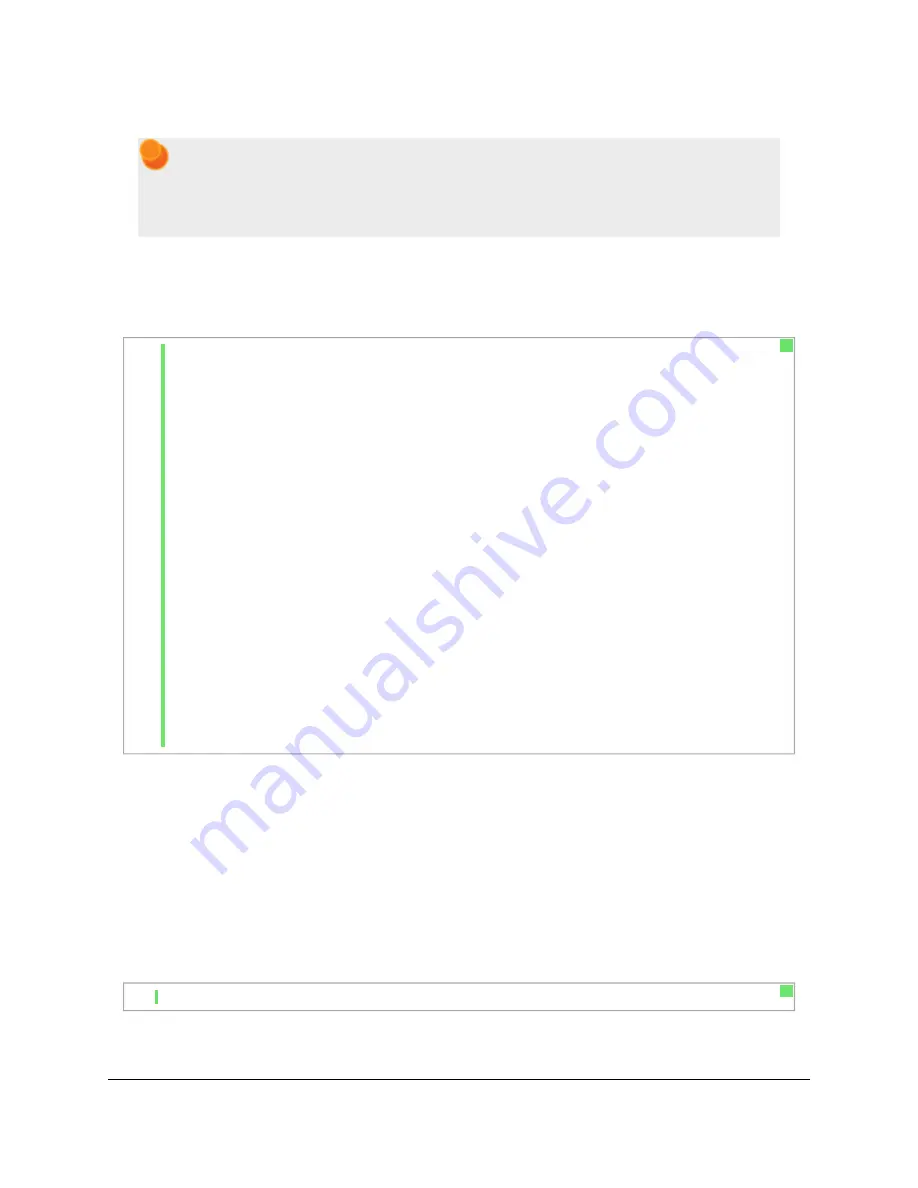
Note:
An Arduino-compatible version of this sample program can be downloaded as part
of the
[https://www.pololu.com/docs/0J17]
(see
) The
Arduino sample sketch is all contained within a single file.
This program is much more complicated than the examples you have seen so far, so we have split
it up into multiple files. Using multiple files makes it easier for you to keep track of your code. For
example, the file
turn.c
contains only a single function, used to make turns at the intersections:
The first line of the file, like any C file that you will be writing for the 3pi, contains an include command
that gives you access to the functions in the Pololu AVR Library. Within
turn()
, we then use the library
functions
delay_ms()
and
set_motors()
to perform left turns, right turns, and U-turns. Straight “turns”
are also handled by this function, though they don’t require us to take any action. The motor speeds
and the timings for the turns are parameters that needed to be adjusted for the 3pi; as you work on
making your maze solver faster, these are some of the numbers that you might need to adjust.
To access this function from other C files, we need a “header file”, which is called
turn.h
. The header
file just contains a single line:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
#include <pololu/3pi.h>
// Turns according to the parameter dir, which should be 'L', 'R', 'S'
// (straight), or 'B' (back).
void
turn(
char
dir)
{
switch
(dir)
{
case
'L'
:
// Turn left.
set_motors(-80,80);
delay_ms(200);
break
;
case
'R'
:
// Turn right.
set_motors(80,-80);
delay_ms(200);
break
;
case
'B'
:
// Turn around.
set_motors(80,-80);
delay_ms(400);
break
;
case
'S'
:
// Don't do anything!
break
;
}
}
1
void
turn(
char
dir);
Pololu 3pi Robot User’s Guide
© 2001–2019 Pololu Corporation
8. Example Project #2: Maze Solving
Page 38 of 85
Содержание 0J5840
Страница 24: ...Pololu 3pi Robot User s Guide 2001 2019 Pololu Corporation 5 How Your 3pi Works Page 24 of 85...
Страница 67: ...Source code Pololu 3pi Robot User s Guide 2001 2019 Pololu Corporation 10 Expansion Information Page 67 of 85...
Страница 77: ...Source code Pololu 3pi Robot User s Guide 2001 2019 Pololu Corporation 10 Expansion Information Page 77 of 85...