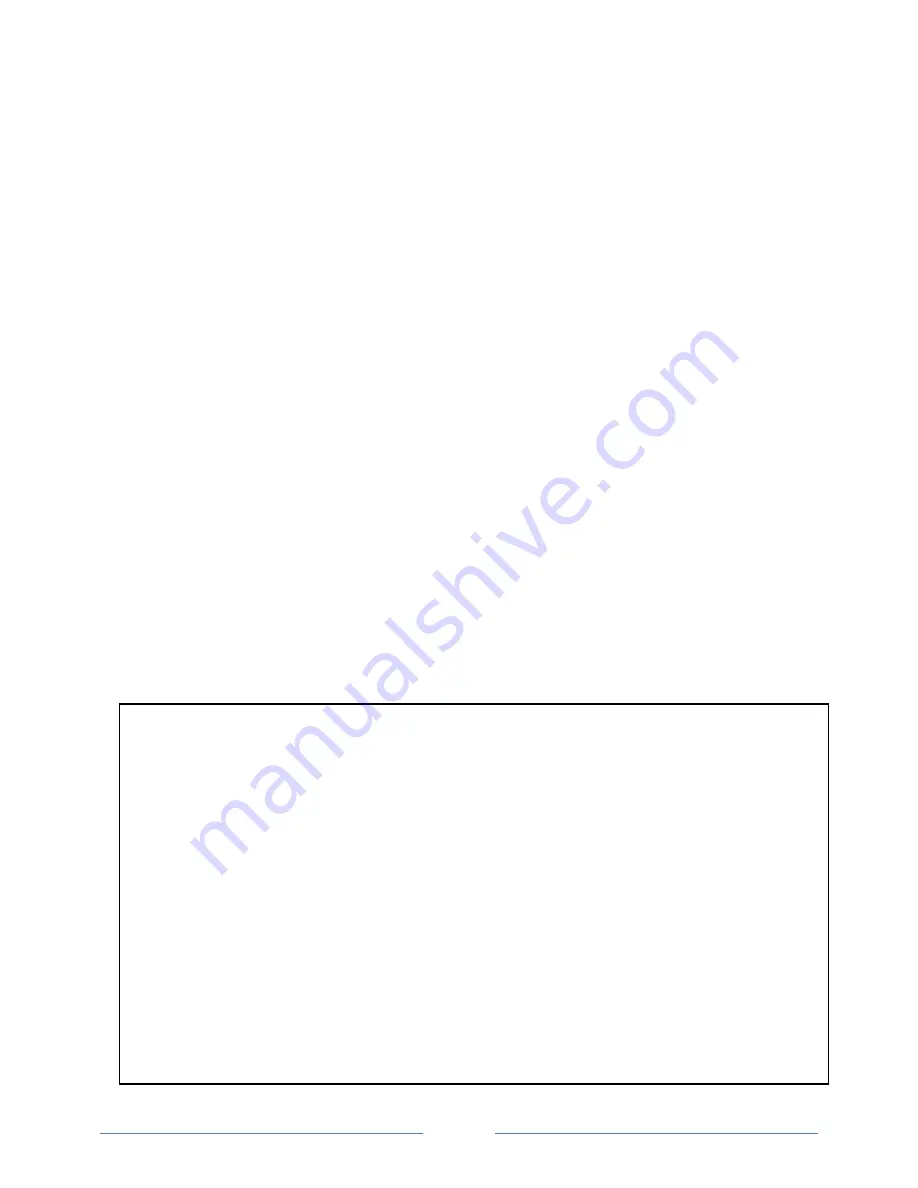
Page 49
Sample Program Using a Thread for Monitoring a Sensor
In computer usage, the term thread is short for the phrase "thread of execution", and represents a
sequence of instructions to be managed by the system as it schedules processing time among running
processes. On a single processor machine, like the KIPR Link, the instructions running in separate
threads appear to be operating in parallel. Each thread, once started, will continue until its process
finishes or until it is forcibly terminated by another process using the
thread_destroy
function. Each
active thread gets a small amount of time in turn until all of its statements have been executed or it is
forcibly terminated. If a thread's process cannot complete all of its statements before its turn is over, it
is paused temporarily for the next thread to gets its share of time. This continues until all the active
threads have gotten their slice of time and then it all repeats. Assuming the processor is fast enough,
from the user's viewpoint it appears that all of the active processes are running in parallel.
Functions running in threads can communicate with one another by reading and modifying global
variables. The global variables can be used as semaphores so that one process can signal another.
Process IDs may also be stored in global variables so that one process can destroy another one's thread
if that is necessary program logic (think in terms of a process that is in an indefinite loop monitoring
sensors, so it would never finish otherwise).
There are four KIPR Link Library functions for controlling threads,
thread_create
,
thread_destroy
,
thread_start
, and
thread_wait
. A variable used for retaining a thread's system id must have the special
data type,
thread
.
In the following example a thread is employed for running a function that monitors the side_button,
raising a flag if it has been pressed. This way, a button press that occurs while the main function is in
sleep mode is still captured. The flag is implemented using a global variable.
Code
int
flag =
0
;
// global flag to signal when side button pressed
void
chksens() {
while
(
1
) {
if
(side_button()) flag =
1
;
// if button pressed, flag it
msleep(
100
);
// keep checking side button (every 1/10th second)
}
}
int
main() {
int
cnt =
0
;
thread
tid;
// thread variable for holding thread id
tid = thread_create(chksens);
// create a thread for chksens
thread_start(tid);
// start chksens running in its thread
while
(flag ==
0
) {
// thread is still running while main sleeps
display_printf(
1
,
1
,
"elapsed time %d "
,++cnt);
msleep(
1000
);
}
thread_destroy(tid);
// remove the thread
printf(
"\ndone\n"
);
}
Содержание KIPR Link
Страница 1: ...KIPR Link Manual Version BB2014 1 1...