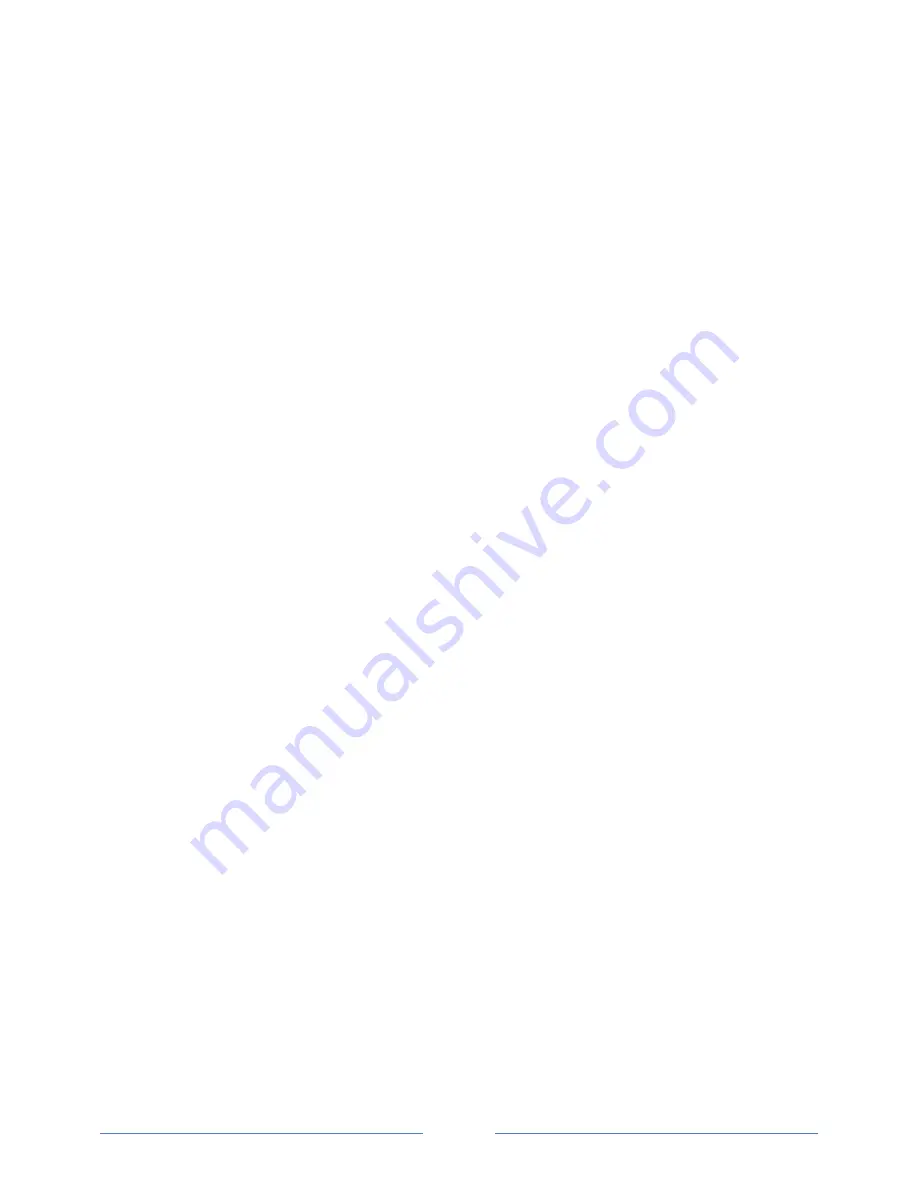
Page 65
side_button_clicked [Category: Sensors]
Format:
int
side_button_clicked();
Gets the Side button's state (pressed or not pressed). If pressed, blocks until released. Returns
1 for pressed, 0 for not pressed. The construction
while
(side_button()==0) {
while
(side_button()==1); . . .}
//debounce Side button
is equivalent to
while
(side_button_clicked()==0) {. . .}
sin [Category: Math]
Format:
double
sin(
double
angle);
Returns the sine of angle.
angle
is specified in radians; result is in radians.
sqrt [Category: Math]
Format:
double
sqrt(
double
num);
Returns the square root of num.
tan [Category: Math]
Format:
double
tan(
double
angle);
Returns the tangent of angle.
angle
is specified in radians; result is in radians.
thread_create [Category: Threads]
Format:
thread
thread_create(
<
function name
>
);
thread_create
is used to create a thread for running a function in parallel to
main
, returning a
thread ID value of type
thread
. The special data type
thread
is for the thread IDs used by the
system to keep track of active threads. Note that the returned value must be assigned to a
variable of type
thread
to remain available. When a function is run in a thread (via
thread_start
), the thread will remain active until the function finishes or the thread is
destroyed (via
thread_destroy
). If the thread hasn't been destroyed, it can be started again;
otherwise, a new thread has to be created for the function.
thread_destroy [Category: Threads]
Format:
void
thread_destroy(
thread
id);
thread_destroy
is used to destroy a thread created for a function. A thread is destroyed by
passing its thread ID to
thread_destroy
. The following example shows the
main
process
creating a check_sensor thread, running it in parallel via
thread_start
, and then destroying it
one second later (whether or not the thread is still active):
int
main() {
thread
tid;
tid = thread_create(check_sensor);
thread_start(tid);
msleep(1000);
thread_destroy(tid);
}
thread_start [Category: Threads]
Format:
void
thread_start(
thread
id);
thread_start
is used to activate a thread, running its associated function in parallel with
main
and any other active threads. The
thread
variable used in the argument must have a thread ID
value as returned by
thread_create
. Note that thread IDs generated by
thread_create
must be
retained in variables of type
thread
to remain available for later use. The thread is active until
its function finishes or until it is terminated by
thread_destroy
.
Содержание KIPR Link
Страница 1: ...KIPR Link Manual Version BB2014 1 1...