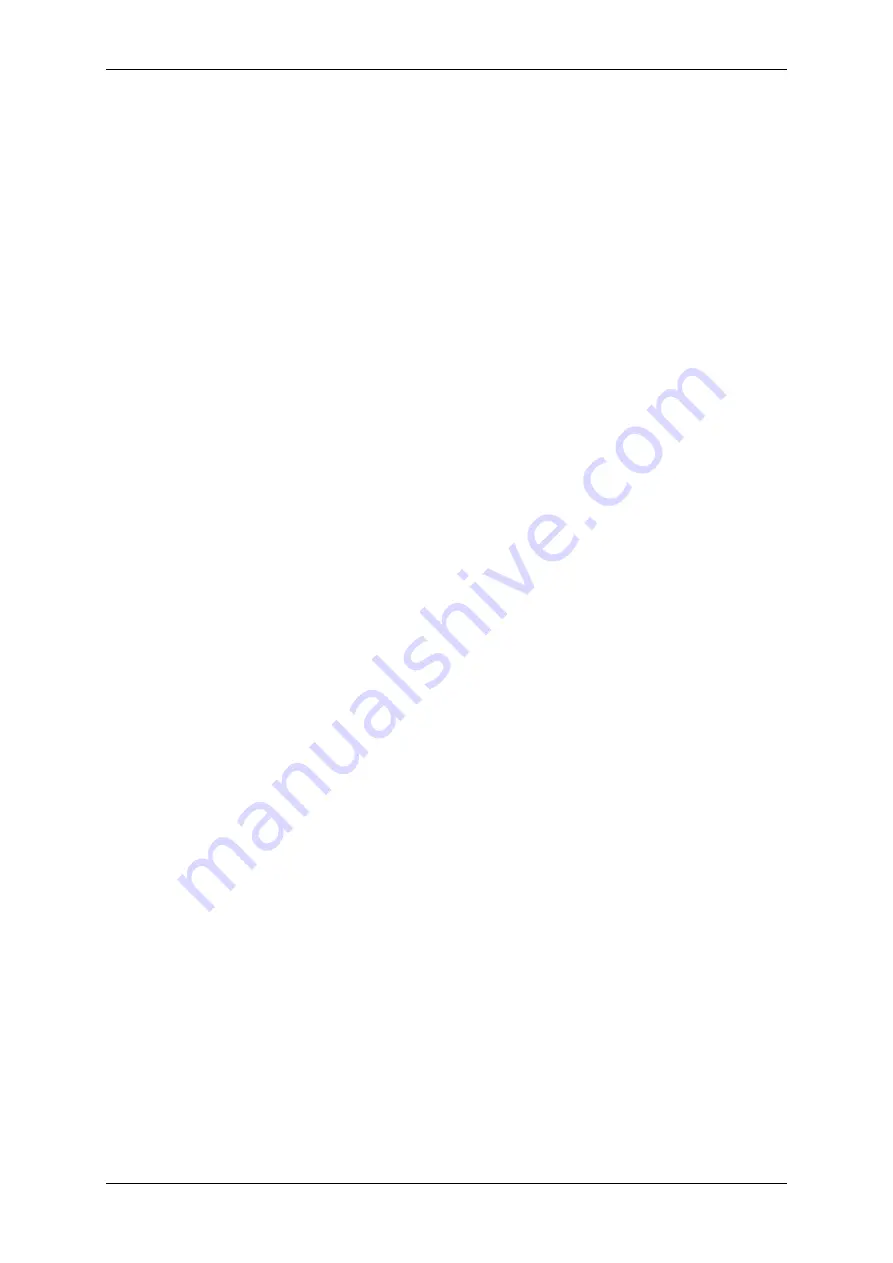
7 Scripting
37
You should not usually poll the same queue from different threads. Every event from a queue will be processed by
a single thread only. A single event can be placed into multiple queues though.
Like any other loop, a loop over
event.stream(...)
can be terminated via a
break
statement in the body.
The catch is that the loop body isn't executed until a matching event occurs. If you need to terminate a thread's
event loop from another thread, you may e.g. create and share an event queue between them, and place an event
into it when you want to terminate the loop, like this:
local function event_thread(cancel_queue)
...
for i,t,data in event.stream(cancel_queue,...) do
if i==1 then
break
else
...
end
end
...
end
function caller_thread()
local cancel_queue=event.queue()
thread.run(function() return event_thread(cancel_queue) end)
...
cancel_queue[#cance1]={}
...
end
There are obviously other ways to share a queue like this. The location of
cancel_queue
in the
event.
←
-
stream
argument list above affects whether the loop is terminated immediately after receiving the cancellation
message or after processing outstanding events in other queues.
You can also just use
thread.kill
if you don't need the looping thread at all any longer.
7.6.2.14
Utility functions
The global
util
table provides helpful utility constants and functions which usually have no state or side effects.
util.hex
,
util.base64
,
util.url
are tables with
encode
and
decode
members which perform hex,
Base64 and URL component encoding and decoding, respectively; they both accept and accept strings.
The
util.json
table also contains
encode
and
decode
members, which perform JSON encoding and decod-
ing, respectively.
util.json.encode
accepts any regular value (without cycles) and returns a string;
util.
←
-
json.decode
accepts a string and returns a value corresponding to it.
Due to how Lua handles tables, an empty JSON array
[]
cannot be distinguished from an empty JSON object
{}
once decoded. To help that,
util.json.encode
accepts an optional second argument, which is an "empty
array table", that is, a table with keys corresponding to empty Lua tables in the input which represent empty JSON
arrays. For example,
local data={a={},b={}}
log.notice("%s",util.json.encode(data,{[data.b]=true}))
produces:
{"a":{},"b":[]}
Conversely,
util.json.decode
returns an empty array table as a second value if it is nonempty (that is, if there
were empty arrays in the input).
DLI LPC9 User’s Guide: 1.7.24.0
Содержание LPC9
Страница 1: ...DLI LPC9 User s Guide 1 7 24 0 ...
Страница 81: ......