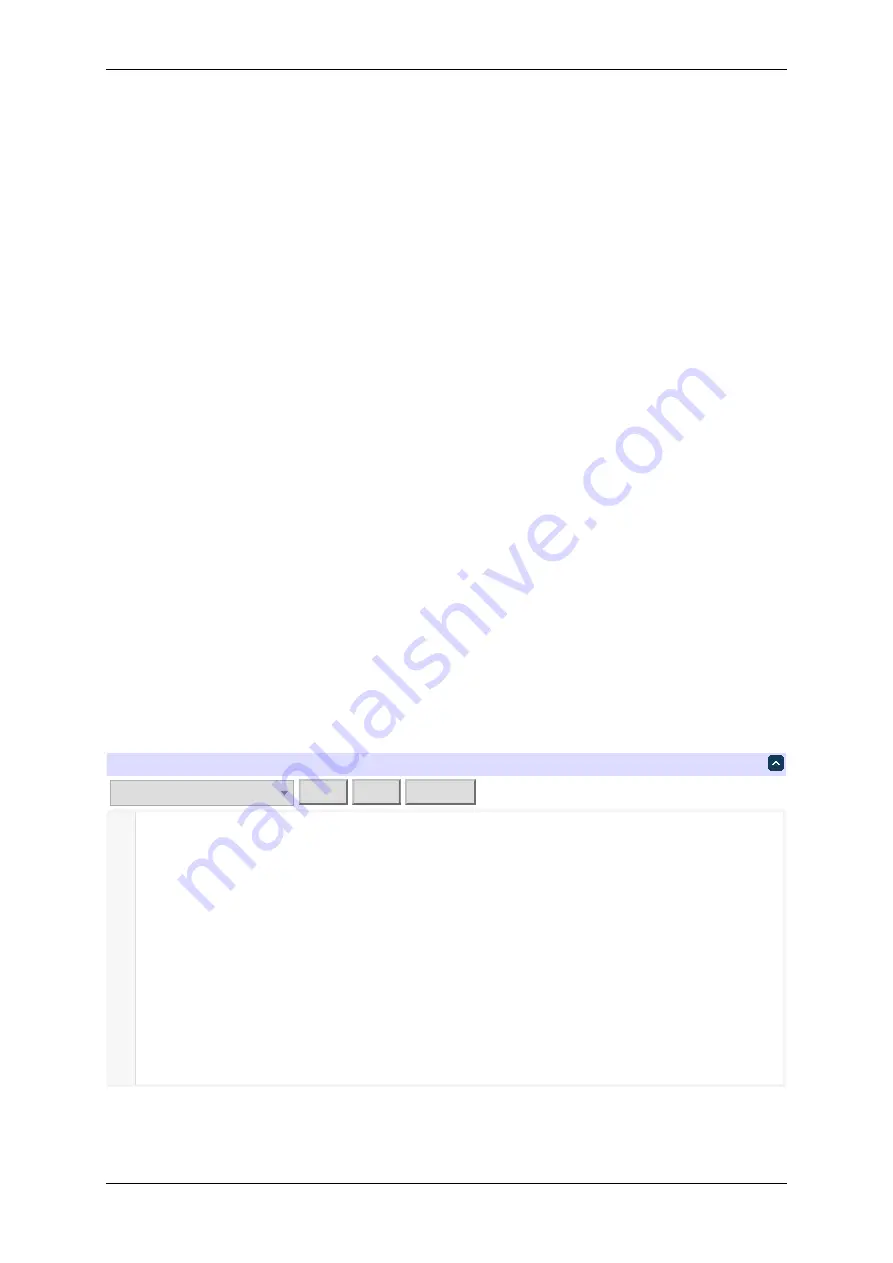
24
CONTENTS
This works as long as all calls to
my_internal_function
occur in other functions not immediately executed.
my_internal_function
is still local (not visible externally). This works because a function is just another type
of value, and
function fn()...end
is just like
fn=function()...
end
, so it works the same way as
local x
...
x=5
Here,
x
has the value
nil
until it's assigned the value
5
. Similarly,
local function x()...
end
is just
like
local x; x=function()...
end
.
Functions in Lua are called with their arguments parenthesized, e.g.
func(arg1,arg2)
. Functions with no
arguments are called with empty parentheses, like
func()
. However, according to Lua syntax, a single string
function argument doesn't need parentheses, thus allowing the BASIC-like commands to avoid them if there's only
one argument.
You can also create functions with arbitrary names (including Unicode) like this:
_G["Turn on lamp"]=function()
... statements go here ...
end
All the above declarations are essentially assignments to (global and local) variables. Therefore, if multiple scripts
functions have the same name, the one closest to the bottom will effectively mask preceding ones.
When you're prompted for a script function to choose, choices will appear in the order they're specified in the script
source. If you wish to change that, you can define a global
ui_order
table with a list of quoted function names to
appear at the top of the list, in the desired order (others will be at the bottom of the list in alphabetical order). E.g.
ui_order={"important_function","frequently_useful_function"}
Most APIs listed below are only available when used inside functions, not in the global context. That is, attempts to
write e.g.
ON(1)
without an enclosing function will fail (such actions cannot be taken at script load time).
7.4
Snippets
Snippets are script fragments not part of the main script, stored for later use or shipped with the device.
Script snippets
Load Save Remove
default.lua_table_demo
function
test_table_manipulations()
local
a={
"1"
,
"2"
,
"3"
}
assert
(a[
3
]==
"3"
)
assert
(a[
4
]==
nil
)
table.insert
(a,
"x"
)
assert
(a[
4
]==
"x"
)
table.insert
(a,
1
,
"y"
)
assert
(a[
1
]==
"y"
)
assert
(a[
2
]==
"1"
)
assert
(a[
5
]==
"x"
)
table.remove
(a,
2
)
assert
(a[
1
]==
"y"
)
assert
(a[
2
]==
"2"
)
assert
(a[
4
]==
"x"
)
assert
(a[
5
]==
nil
)
table.sort
(a,
function
(u,v)
return
u>v
end
)
assert
(
table.concat
(a)==
"yx32"
)
LOG(
"Table tests passed"
)
end
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
User script snippet editor
DLI LPC9 User’s Guide: 1.7.24.0
Содержание LPC9
Страница 1: ...DLI LPC9 User s Guide 1 7 24 0 ...
Страница 81: ......