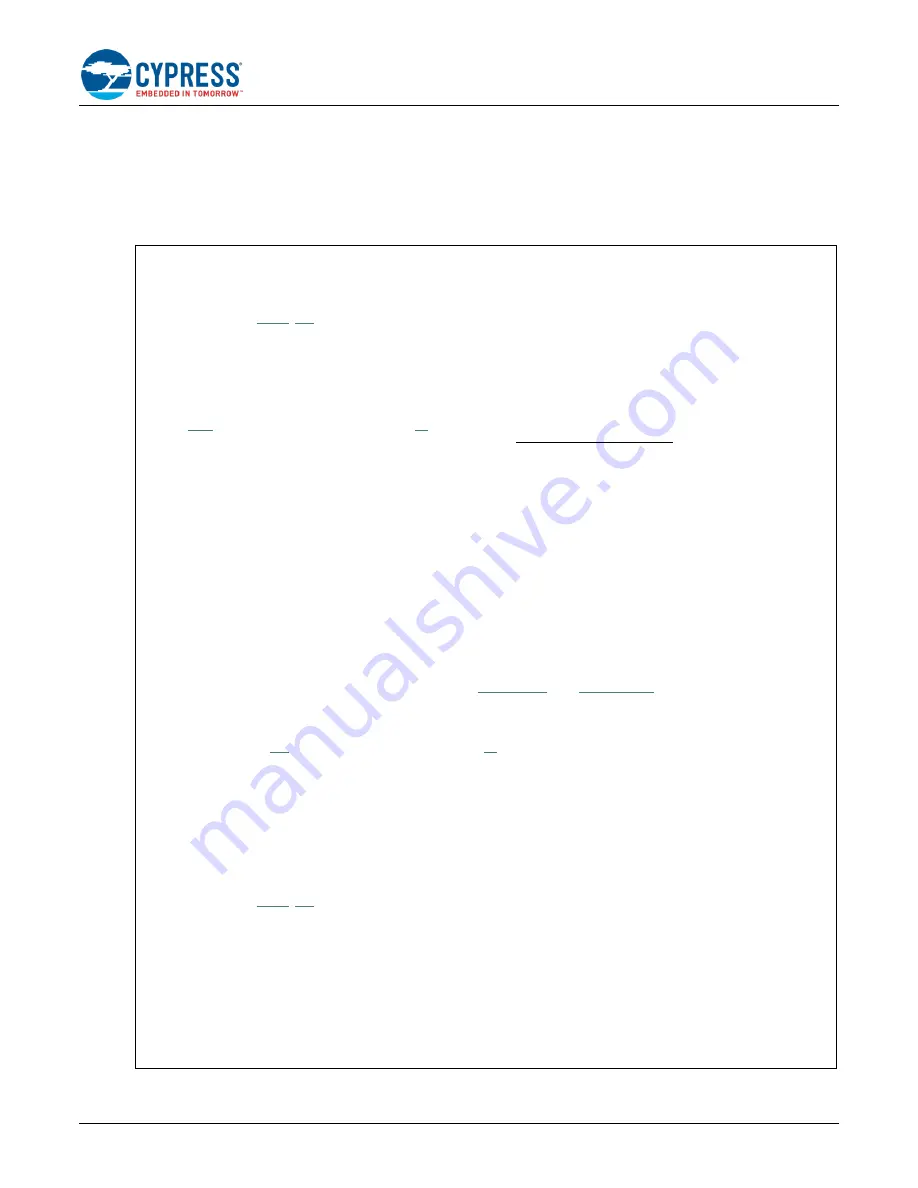
Getting Started with EZ-BT WICED Modules
Document Number: 002-23400 Rev. **
30
The next function to look at is
application_init
; it runs after the ROM-driven Bluetooth stack initialization is
succesful. (the
BTM_ENABLED_EVT
event is generated by the stack). This function sets up the application-specific
behavior, initializes the SPP library, initializes the SDP database for Bluetooth BR/EDR, initializes the GATT database,
registers application callbacks to occur when various link layer, GAP, or GATT events occur within the stack, initializes
timers, and configures the button and LED based on the application requirements as shown in
Code 16.
application_init
: Application-Driven Initialization
void
application_init
(
void
)
{
wiced_bt_gatt_status_t
gatt_status;
wiced_result_t
result;
/* Initialize wiced app */
wiced_bt_app_init();
#if
SEND_DATA_ON_INTERRUPT
/* Configure the button available on the platform */
wiced_hal_gpio_configure_pin( WICED_GPIO_BUTTON, WICED_GPIO_BUTTON_SETTINGS(
GPIO_EN_INT_RISING_EDGE
),
WICED_GPIO_BUTTON_DEFAULT_STATE );
wiced_hal_gpio_register_pin_for_interrupt(WICED_GPIO_BUTTON, app_interrupt_handler, NULL);
// init timer that we will use for the rx data flow control.
wiced_init_timer(&app_tx_timer, app_tx_ack_timeout, 0, WICED_MILLI_SECONDS_TIMER);
#endif
app_write_eir();
// Initialize RFCOMM. We will not be using application buffer pool and will rely on the
// stack pools configured in the wiced_bt_cfg.c
wiced_bt_rfcomm_init(MAX_TX_BUFFER, 1);
// Initialize SPP library
wiced_bt_spp_startup(&spp_reg);
#ifdef
HCI_TRACE_OVER_TRANSPORT
// There is a virtual HCI interface between upper layers of the stack and
// the controller portion of the chip with lower layers of the BT stack.
// Register with the stack to receive all HCI commands, events and data.
wiced_bt_dev_register_hci_trace(app_trace_callback);
#endif
/* create SDP records */
wiced_bt_sdp_db_init((
uint8_t
*)app_sdp_db,
sizeof
(app_sdp_db));
// This application will always configure device connectable and discoverable
wiced_bt_dev_set_discoverability(
BTM_GENERAL_DISCOVERABLE
, 0x0012, 0x0800);
wiced_bt_dev_set_connectability(
BTM_CONNECTABLE
, 0x0012, 0x0800);
#if
SEND_DATA_ON_TIMEOUT
/* Starting the app timers, seconds timer and the ms timer */
wiced_bt_app_start_timer(1, 0, app_timeout, NULL);
#endif
hello_sensor_application_init();
}
void
hello_sensor_application_init
(
void
)
{
wiced_bt_gatt_status_t
gatt_status;
wiced_result_t
result;
/* Initialize wiced app */
// wiced_bt_app_init();
wiced_bt_app_led_init();
/* Configure buttons available on the platform (pin should be configured before registering interrupt
handler ) */
// wiced_hal_gpio_configure_pin( WICED_GPIO_BUTTON, WICED_GPIO_BUTTON_SETTINGS( GPIO_EN_INT_RISING_EDGE
// ), WICED_GPIO_BUTTON_DEFAULT_STATE );
// wiced_hal_gpio_register_pin_for_interrupt( WICED_GPIO_BUTTON, hello_sensor_interrupt_handler, NULL );
/* Register with stack to receive GATT callback */
gatt_status = wiced_bt_gatt_register( hello_sensor_gatts_callback );
WICED_BT_TRACE(
"wiced_bt_gatt_register: %d\n"
, gatt_status );