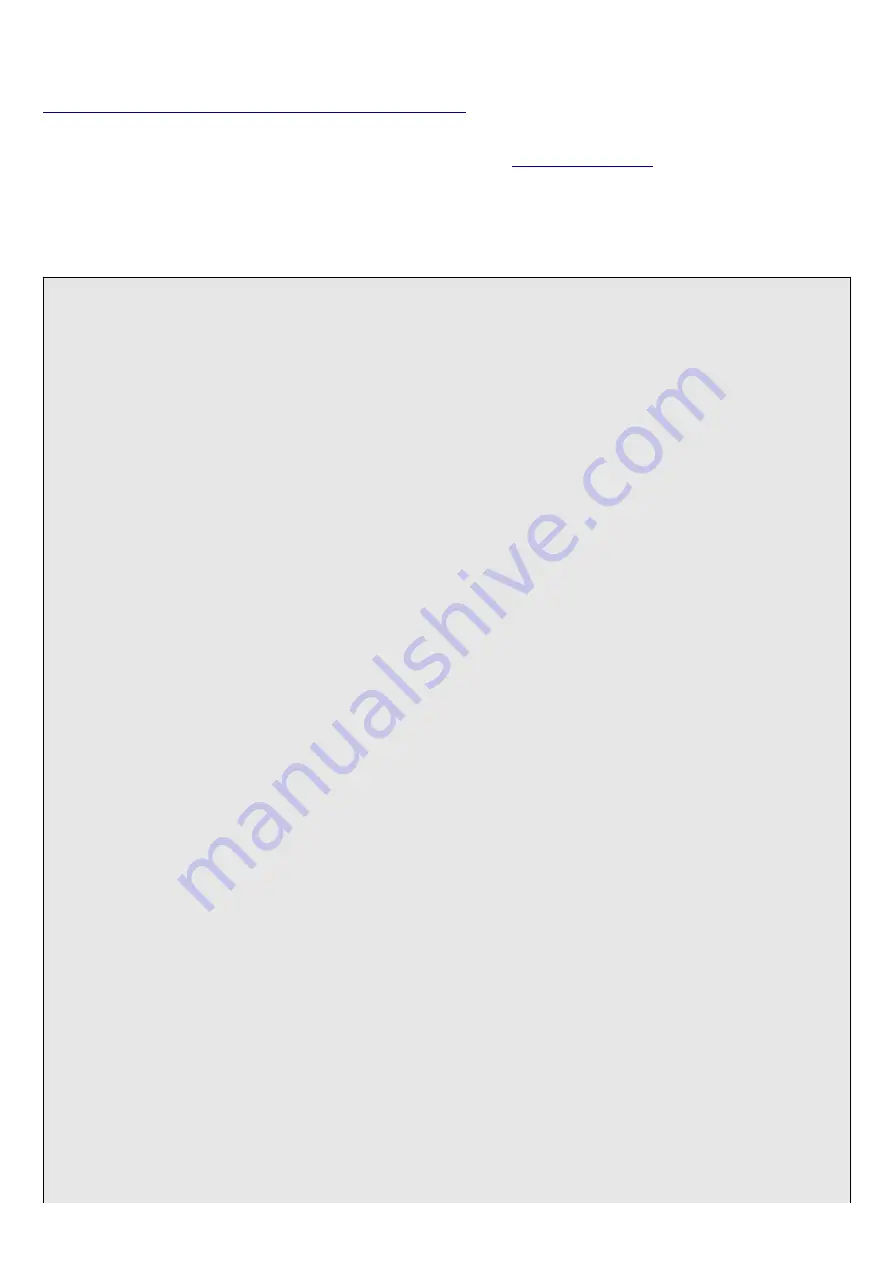
PROGRAMMING THE CPU WITH ARDUINO
You need a computer with Arduino IDE installed. Get the IDE from the URL
https://www.arduino.cc/en/Main/Software
and install it on your computer
following all the instructions given by the Arduino documentation.
If you haven't downloaded it yet, go to
Arduino sketch for the D9U from the Support section.
Below the complete sketch listing with comments.
////////////////////////////////////////////////////////////////////
// Crumar Drawbar Controller D9U
// by Guido Scognamiglio
// Runs on Atmel ATmega32U4 Arduino Leonardo (with MIDI USB Library)
// Reads 9 analog inputs from internal ADCs
// Sends MIDI CC numbers 12-20 or 21-29 according to selected mode
// Last update: July 2018
//
////////////////////////////////////////////////////////////////////
// This is where you can define your CC numbers for the Bank 0 or 1
int CCMap[2][9] =
{
{ 12, 13, 14, 15, 16, 17, 18, 19, 20 }, // Upper drawbars
{ 21, 22, 23, 24, 25, 26, 27, 28, 29 } // Lower drawbars
};
////////////////////////////////////////////////////////////////////
// You should not modify anything else below this line
// unless you know what you're doing.
////////////////////////////////////////////////////////////////////
// Define I/O pins
#define LED_RED 15
#define LED_GREEN 16
#define BUTTON 5
// Define global modes
#define DEBOUNCE_TIME 150
#define DEADBAND 8
// Include libraries
#include <EEPROM.h>
#include <MIDIUSB.h>
#include <MIDI.h>
MIDI_CREATE_DEFAULT_INSTANCE();
// Init global variables
int mode = 1; // Should be either 0 or 1
int prev_val[9] = { -1, -1, -1, -1, -1, -1, -1, -1, -1 };
int debounce_timer = DEBOUNCE_TIME;
// ADC reference map
int ADCmap[9] = { A0, A1, A2, A3, A6, A7, A8, A9, A10 };
int ADCcnt = 0;