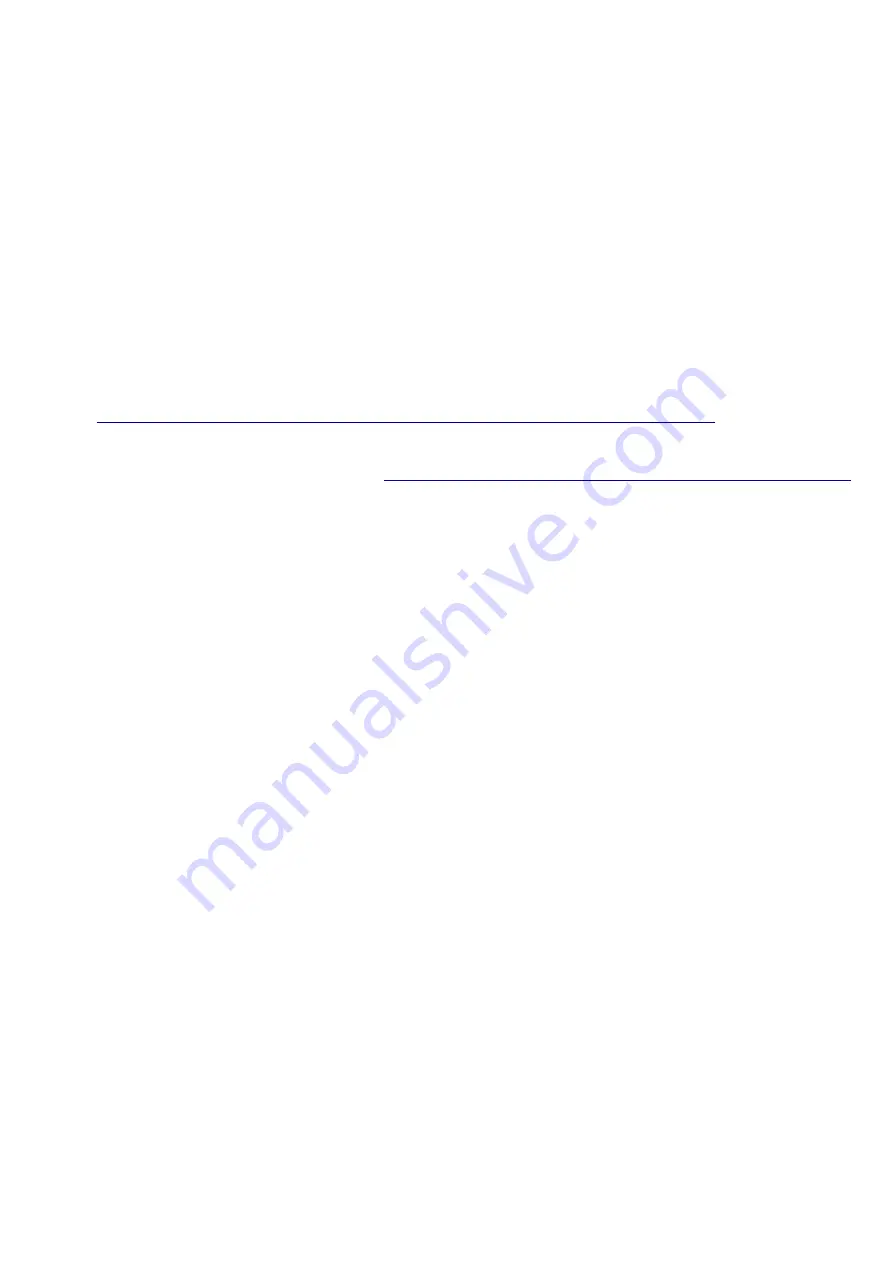
THE ARDUINO SKETCH EXPLAINED
The Atmel Atmega32U4 processor used on the Arduino Leonardo (or Pro Micro)
board has 9 built-in ADC inputs (Analog-to-digital converters), just what
we need to read 9 linear 10K potentiometers, that in our case are the
drawbars. All this sketch has to do is to read the drawbars and generate
MIDI CC messages. Optionally, it can read a push-button and lit 2 LEDs
that are used to swtich between two banks of pre-defined CC numbers, that
we can use to control either the Upper or the Lower manual of a Clonewheel
organ.
The MIDI stream is output both from the USB-MIDI port, using the 32u4
built-in USB controller, and from the UART port clocked at 31250 Baud as
the MIDI specs require.
So this sketch uses the following libraries (that should be installed
separately using the IDE library functions, in case they aren't pre-
installed):
–
MIDI
Library
by
Fourty
Seven
Effects
-
https://github.com/FortySevenEffects/arduino_midi_library
Used to
generate MIDI messages to be sent to the UART PORT
–
https://www.arduino.cc/en/Reference/MIDIUSB
Used to generate MIDI messages to be sent via USB
–
EEPROM (built-in) Used to store and recall the selected CC bank into
the internal EEPROM
The loop() function cycles through the 9 ADCs by reading their current
values and storing them into an array; the same function also "listens"
whether the push button has been depressed (contact to ground, value LOW).
The pin to which the button is connected has an internal pull-up resistor.
The DoDrawbar() function compares the value of a given ADC read by loop()
with the previous value of the same ADC, if the difference is greater than
the value set by DEADBAND, it is considered as a potentiometer value
change and generates the MIDI event to be sent. The DEADBAND value is set
to 8, because the ADCs have a 10 bit resolution (2^10 = 1024 values), but
we need to scale it down to 7 bits (2^7 = 128 value), so we can discard 7
values each 8. In other words, if previous value was 1000 and current is
1004, nothing changes, but if the new value is 1009, then this is
interpreted as a value change. This mainly prevents unwanted messages to
be sent when drawbars aren't actually moved by the user.
The SendMidiCC() function generates the actual MIDI CC message, while
set_mode() is called each time the button is depressed, switches the LED,
sets the value of the "mode" variable and stores it into the internal
EEPROM.
The CC numbers to be used are stored into the two-dimensional array
CCMap[2][9], which, as the numbers in the brackets suggest, contains 2
banks of 9 values each.