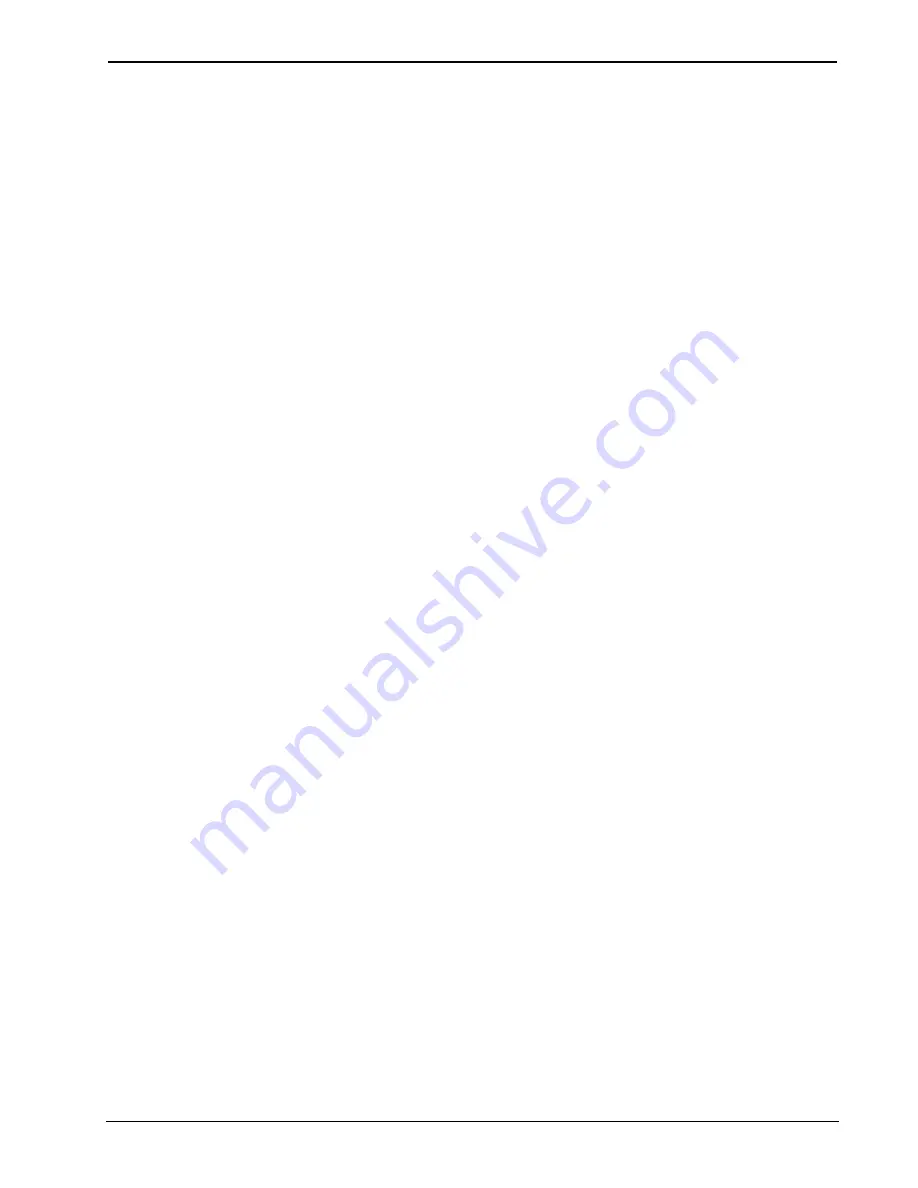
Crestron
SIMPL+
Software
String1 = itoa(int1);
position = find(“Artist”,CD_data);
int3 = max(int1, int2);
For clarity, here are some example statements using system functions that do not
have return values:
Print(“Variable int1 = %d\n”,int1);
ProcessLogic();
CancelWait(VCRWait);
It should be clear that, at least as far as system functions go, whether or not a
function returns a value depends largely upon what that function is designed to do.
For example, the
itoa
function would not be very valuable if it did not return a string,
which could then be assigned to a variable, or used inside of an expression. On the
other hand, the
function simply outputs text to the console for viewing, and
thus no return value is needed.
Allowing a user-defined function to return a value is extremely useful, as it allows
such functions to be used in flexible ways, such as inside of an expression. To enable
a function to return a value, use a modified version of the function keyword, as
shown below:
STRING_FUNCTION strfunc1(); //function returns a string
INTEGER_FUNCTION intfunc1(); //function returns an integer
FUNCTION func1(); //function has no return value
Clearly, functions defined using the STRING_FUNCTION keyword will return a
string value, and those defined using the INTEGER_FUNCTION keyword will
return an integer value. Function declared using the function keyword would have no
return value.
Once a function has been declared using the appropriate function type, it is the
responsibility of the programmer to ensure that the proper value is returned. This is
accomplished using the
return
function. To illustrate this, examine the following
function example, which raises one number to the power determined by the second
argument, and returns the result.
INTEGER_FUNCTION power(INTEGER base, INTEGER exponent)
{
INTEGER i, result;
if (base = 0)
return (0);
else if (exponent = 0)
return (1);
else {
result = 0; // initialize result
for (i = 1 to exponent)
result = result * base;
return (result);
}
}
To use this function in a program, simply call the function just like you would any
built-in system function. Here are a few usage examples:
Programming Guide – DOC. 5789A
SIMPL+
•
37