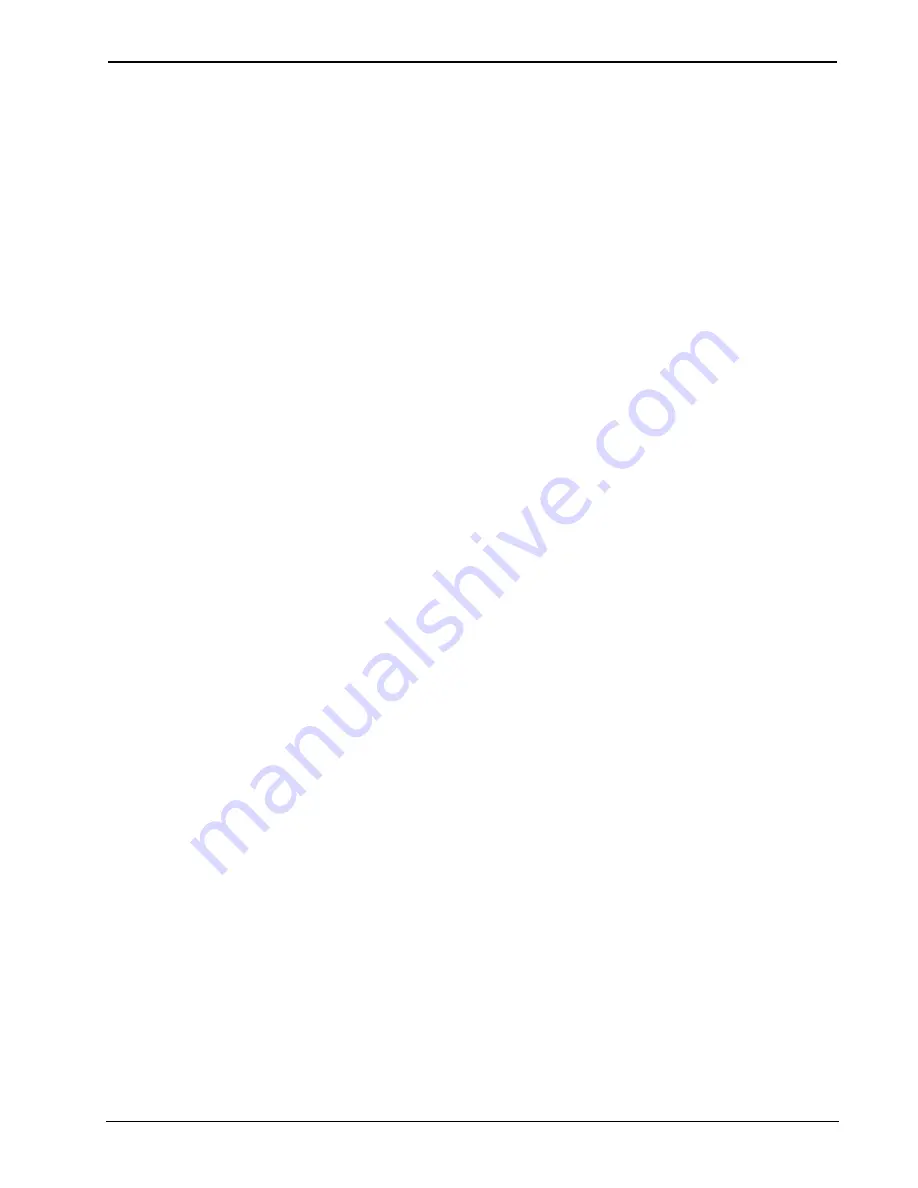
Crestron
SIMPL+
Software
Print("i = %d, j = %d, k = %d\n", i, j, k);
Call sillyFunction();
Print("i = %d, j = %d, k = %d\n", i, j, k);
Call anotherSillyFunction();
Print("i = %d, j = %d, k = %d\n", i, j, k);
}
In this program, it should be clear to see that both of the functions defined, as well as
the push event, have access to the three global integers
i
,
j
, and
k
.
Local variables, on the other hand, can only be accessed within the function in which
they are defined. If another user-defined function or event function tries to access a
local variable defined elsewhere, a compiler error will be generated. In addition, as
soon as the function completes execution, all of the functions' local variables are
"destroyed." This means that any value they contained is lost. The next time this
function is called the local variables are re-created.
Creating local variables is identical to creating global variables, except that the
declaration statement is placed inside of the function definition, as follows:
FUNCTION localExample()
{
INTEGER i, count, test;
STRING s[100], buf[50];
}
Passing Variables to Functions as Arguments
The last section describes how to make your programs easier to read and maintain by
defining variables local to functions. However, this does not change the fact that by
their very nature most functions require access to one or more variables that have
been declared elsewhere in the program, either as global variables or as local
variables in a different function. The question is, how can your function access these
variables.
As we have already seen, global variables are available everywhere in a program,
thus you can simply use such variables to share data between functions and the rest
of the program. This is considered bad programming practice, however, and often
leads to programs that are hard to read and even harder to debug. Functions can also
access any input/output signals defined in the program, but as these signals can be
thought of as global variables, this too is not considered good programming practice.
Instead of using global variables to share data, we instead use the concept of passing
arguments (also known as parameters) into functions. Arguments can be thought of
as an ordered list of variables that are passed to a function by the calling function
(the term calling function simply refers to the scope of the code statement which
calls the function in question). To define a function’s parameters, you list them
inside the parentheses following the function name. A typical function definition
would look like this:
FUNCTION some_function (INTEGER var1, INTEGER var2, STRING
var3)
{
INTEGER localInt;
STRING localStr[100];
var1 = var1 + 1;
localInt = var1 + var2;
Programming Guide – DOC. 5789A
SIMPL+
•
35