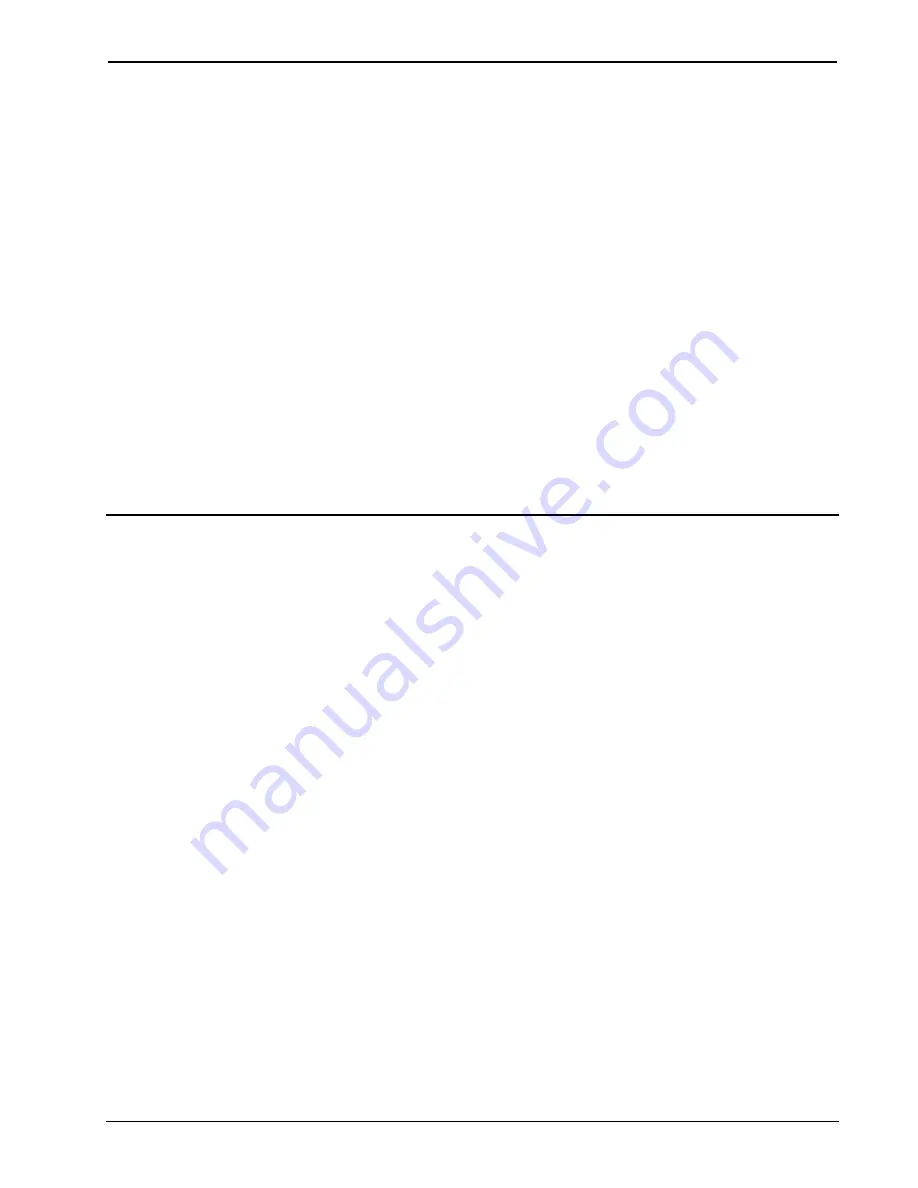
Software
Crestron
SIMPL+
Branching” and “Controlling Program Flow: Loops” on pages 24 and 27,
respectively.
Statements
Statements in SIMPL+ consist of function calls, expressions, assignments, or other
instructions. Statements can be of two types: simple or complex. Simple statements
end in a semicolon (;). Examples of simple statements are as follows:
x = MyInt / 10;
// An assignment
print("hello, world!\n");
// A function call
checksum = atoi(MyString) + 5;
/* Assignment using function
calls and operators */
A complex statement is a collection of simple statements surrounded with curly
braces ( {} ). An example of a complex statement would be as follows:
{ // start of a complex statement
x = MyInt / 10;
print("hello,
world!\n");
checksum = atoi(MyString) + 5;
} // end of a complex statement
Controlling Program Flow: Branching
In any substantial program, making decisions must control the program. SIMPL+
provides two constructs for branching the program based on the value of
expressions:
if-else
and the
switch-case
statement.
if–else
if-else
is the most commonly used branching construct. In its most basic form, it is
structured as follows.
if (expression1)
{
// do something here
}
Where expression1 represents any valid SIMPL+ expression, including variables,
function calls, and operators. If this expression evaluates to TRUE, then the code
inside the braces is executed. If this expression evaluates to FALSE, the code inside
the braces is skipped.
What is the definition of TRUE and FALSE in SIMPL+? As was discussed in
“Working with Data (Variables)” on page 13, expressions, which evaluate to a non-
zero result, are considered TRUE, and expressions that evaluate to 0 are considered
FALSE. For example, refer to the expressions in the table that follows.
24
•
SIMPL+
Programming Guide – DOC. 5789A