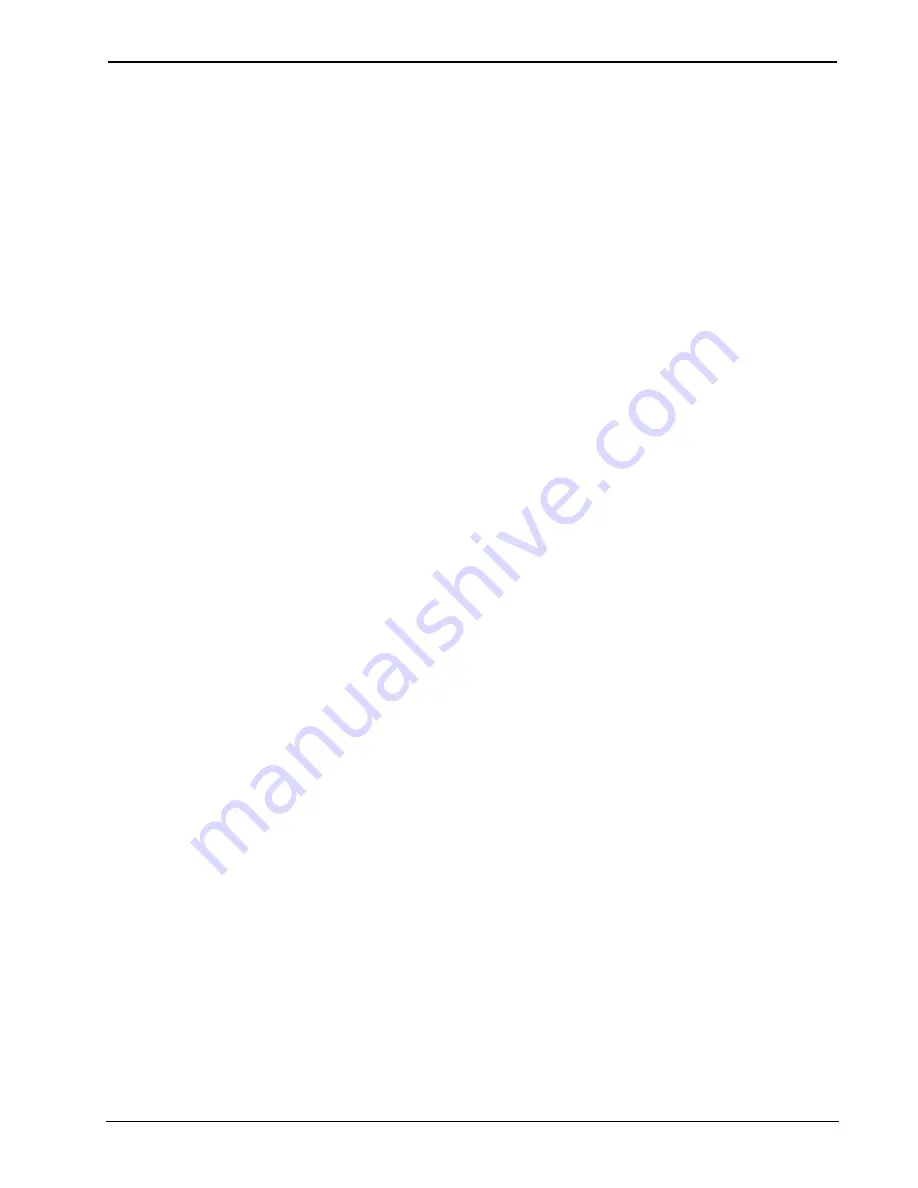
Software
Crestron
SIMPL+
Examine one more example:
INTEGER a, b, c, d;
Function Main()
{
a = 100;
b = -4;
c = a / b; // c = 0 (100/65532)
d = a S/ b; // d = -25 (100/-4)
}
Notice that c calculates to zero because the / operator is unsigned. It treats the
variable b as +65,532. Since the / operator truncates the decimal portion of the result,
c becomes zero. In regard to the variable d, since the signed division operator S/ was
used, b is treated as –4 and the result is –25.
A final note regarding signed/unsigned integers, if an operation results in a number
that is greater than 65,535 that number “overflows” and the value wraps around
again starting at zero. This allows certain operators (e.g. +, -, and *) to operate with
no regard to sign (the result is accurate when thinking of the numbers as signed or
unsigned). This also means that when trying to add (or multiply) two unsigned
numbers and the result is greater than 65,535, the answer may not be what is
expected.
Strings
String variables are used to hold multiple characters in a single variable. The term
string is used to illustrate the act of “stringing” together a number of characters to
produce words, sentences, etc. Typically strings in SIMPL+ are used to hold things
such as serial commands, database records, and so on.
Strings are declared as follows:
STRING <string
1
[size]>, <string
2
[size]>,…,<string
n
[size]>;
The number in square brackets following the variable name defines the size of the
string variable. When declaring strings, choose a size that is large enough to hold any
amount of data that might be needed, but that is not overly large so as to waste space.
That is, it is unnecessary to set the variable size to 100 characters when a given
variable in an application does not contain more than 50 characters.
Working with strings is not unlike working with other variable or signal types. To
assign a value to a string, for example, do the following:
STRING myString[50];
myString = "Tiptoe, through the tulips\n";
In the example above, a variable called
myString
is declared, which can contain up to
50 characters of data. The value,
“Tiptoe, through the tulips\n”
, is the value being
assigned to the variable. The double-quotation marks surrounding the data defines a
literal string expression. That is, a string expression which is defined at compile-
time (when the program is compiled) and cannot change during run-time (while the
program is running). Also note the “
\n
” at the end of this string literal. This
represents a “newline,” or a carriage return followed by a line feed. This character
combination is used often, so a shortcut was developed. For a complete list of similar
shortcuts, refer to the latest revision of the SIMPL+ Language reference Guide (Doc.
5797). Finally, note that the square brackets were not included after the variable
18
•
SIMPL+
Programming Guide – DOC. 5789A