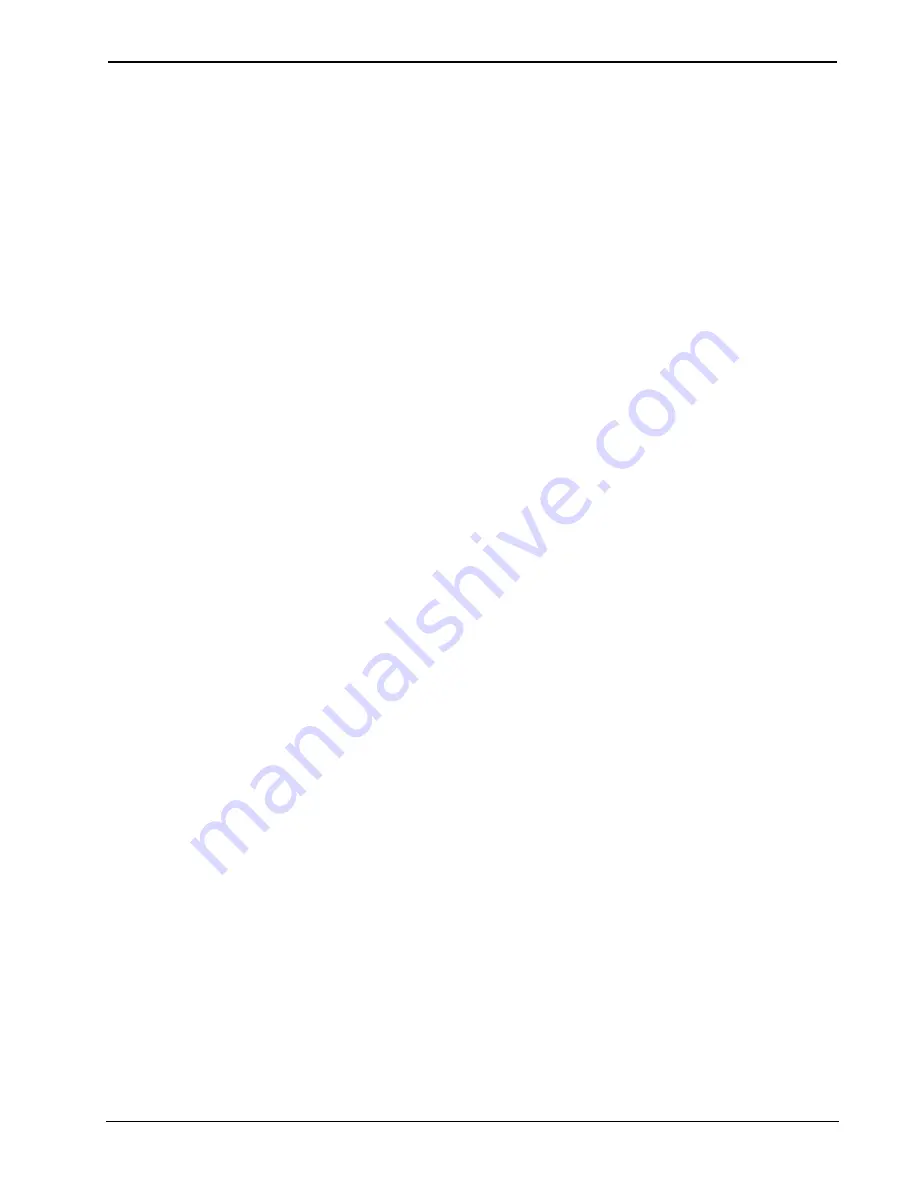
Crestron
SIMPL+
Software
The first two examples above define 1D and 2D integer arrays, respectively. The last
example looks like it declares a 2D array of strings, yet the comments states that it
actually declares a 1D array of strings. Recall that in “Strings”, it was necessary to
define the maximum size of the string in square brackets, which is the same notation
used for arrays. So, in the example above, nine-element array of 50-byte strings is
being declared. The user cannot declare a 2D array of strings in SIMPL+.
Another question should have come to mind from the above examples. That is, why
does declaring
myArray1[15]
create an array with 16 elements instead of 15? The
answer is that array elements start at 0 and go to the declared size (15 in this case).
This fact makes for an easy transition to SIMPL+ for programmers of other
languages (some of which start at 0 and others which start at 1). That is, if the user is
comfortable with treating arrays as starting with element 0, then the user can
continue programming in this manner. If however, the user has used languages,
which treat the first element in an array as element 1, then the user may want to use
that notation instead.
To reference a particular element of an array when programming, use the variable
name followed by the desired element in square brackets. Using the arrays declared
in the example above, the following statements are all valid in SIMPL+.
j = 5;
// set an integer variable to 5
myArray1[3] = j;
// set the 3rd element of the array to 5
myArray1[j*2] = 100; // set the 10th element of the array
// to 100
myArray2[j][1] = k; // set the j,1 element of myArray2 to
// the value of k
m = myArray2[j][k-1]; // set the variable m to the value in
// the j,k-1 element of the array
myArray3[2] = "test"; // set the 3rd element of the string
// array to "test"
From these examples, it should be clear that the user may use constants, variables, or
expressions (discussed in “Operators, Expressions, and Statements” on page 22)
inside of the brackets to access individual array elements. Array elements can appear
on either side of the assignment (=) operator. That is they can be written to (left side)
or read from (right side). Of special interest is the notation used for storing a value
into the string array myArray3. Notice that only one set of brackets was used here
even though two sets of brackets are needed when declaring the array. Remember
that the first set of brackets in the declaration specified the size (in characters) of
each string element. Also recall from earlier in this section, that the size field is not
included when referring to strings. For example, refer to the following.
STRING myString[50]; // declare a 50-character string
myString = "hello!"; // we do not use the size brackets here
// to assign a value to a string variable
As a result, when working with string arrays, only use one set of brackets, which
refer to the array element, not the string size.
Programming Guide – DOC. 5789A
SIMPL+
•
21