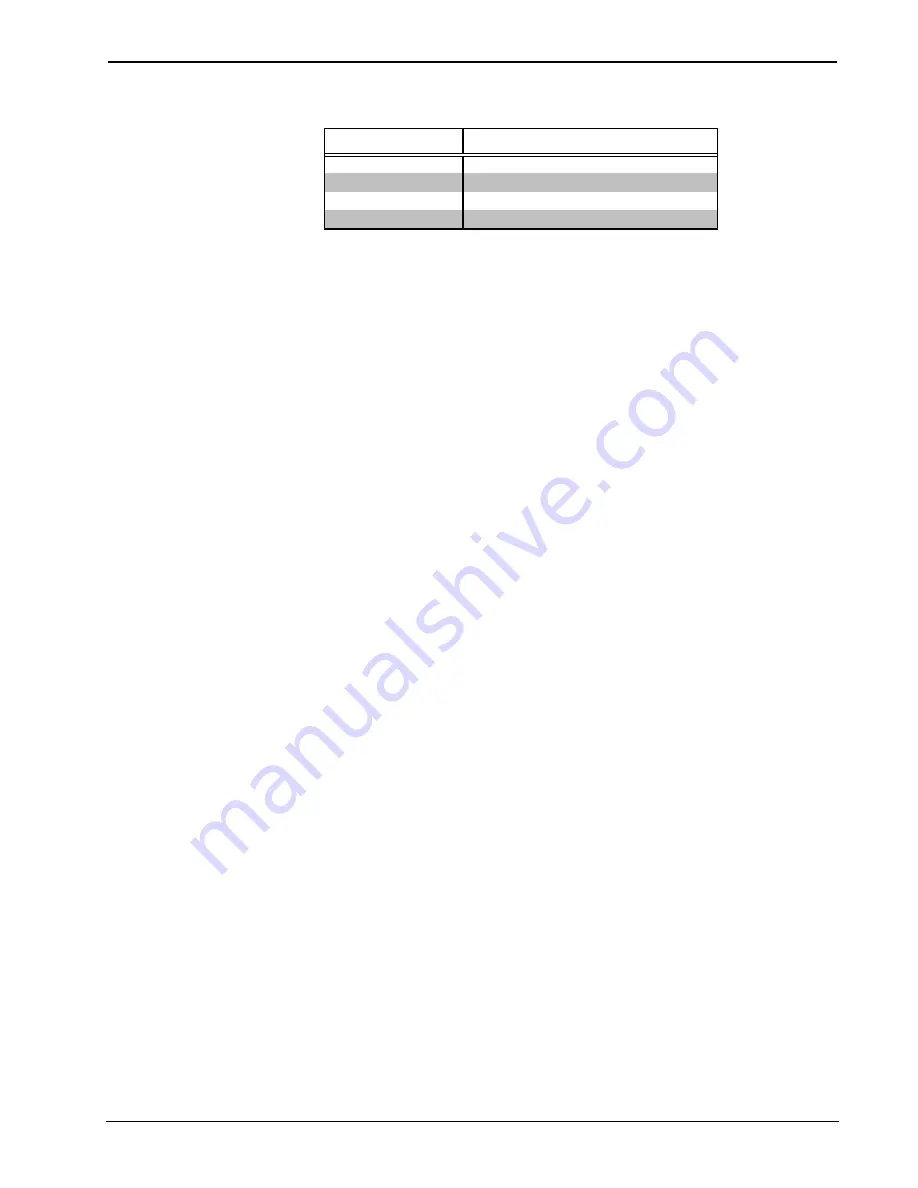
Crestron
SIMPL+
Software
Expressions
EXPRESSION
EVALUATES TO
a = 3
true if a=3, false otherwise
b*4 - a/3
true as long as the result is non-zero
1
always true
0
always false
One limitation with the
if
construct, as shown above, is that the code inside the
if
is
run whenever expression1 evaluates as TRUE, but any code after the closing braces
runs regardless. It is often useful to execute one set of code when a condition is
TRUE and then another set of code if that same condition is FALSE. For this
application, use the
if-else
construct, which looks like the following.
if (expression1)
{
// do something if expression1 is true
}
else
{
// do something else if expression1 is false
}
Programming to anticipate
user errors and handle them
appropriately is called error-
trapping. It is a
recommended programming
practice.
It should be clear that the code following the
if
runs whenever expression1 evaluates
to TRUE and the code following the
else
executes whenever expression1 evaluates
to FALSE. Obviously, there can never be a case where both sections of code execute
together.
The following example is designed to control a CD changer. Before telling the CD
player to go to a particular disc number, it checks to see that the analog value, which
represents the disc number, does not exceed the maximum value.
#DEFINE_CONSTANT NUMDISCS 100
ANALOG_INPUT disc_number;
STRING_OUTPUT CD_command, message;
CHANGE disc_number
{
if (disc_number <= NUMDISCS)
{
CD_command = "DISC " + itoa(disc_number) + "\r";
message = "Changing to disc " + itoa(disc_number) +
"\n";
}
else
{
message = "Illegal disc number\n";
}
}
There is one last variation on the
if-else
statement. In the example above, the
decision to be made is binary. That is, do one thing if this is true, otherwise do
something else. In some cases decisions are not that straight forward. For example,
to check the current day of the week, execute one set of code if it is Saturday,
another set of code if it is Sunday, and yet some other code if it is any other day of
the week. One way to accomplish this is by using a series of
if-else
statements. For
this example, the code might look like the following.
Programming Guide – DOC. 5789A
SIMPL+
•
25