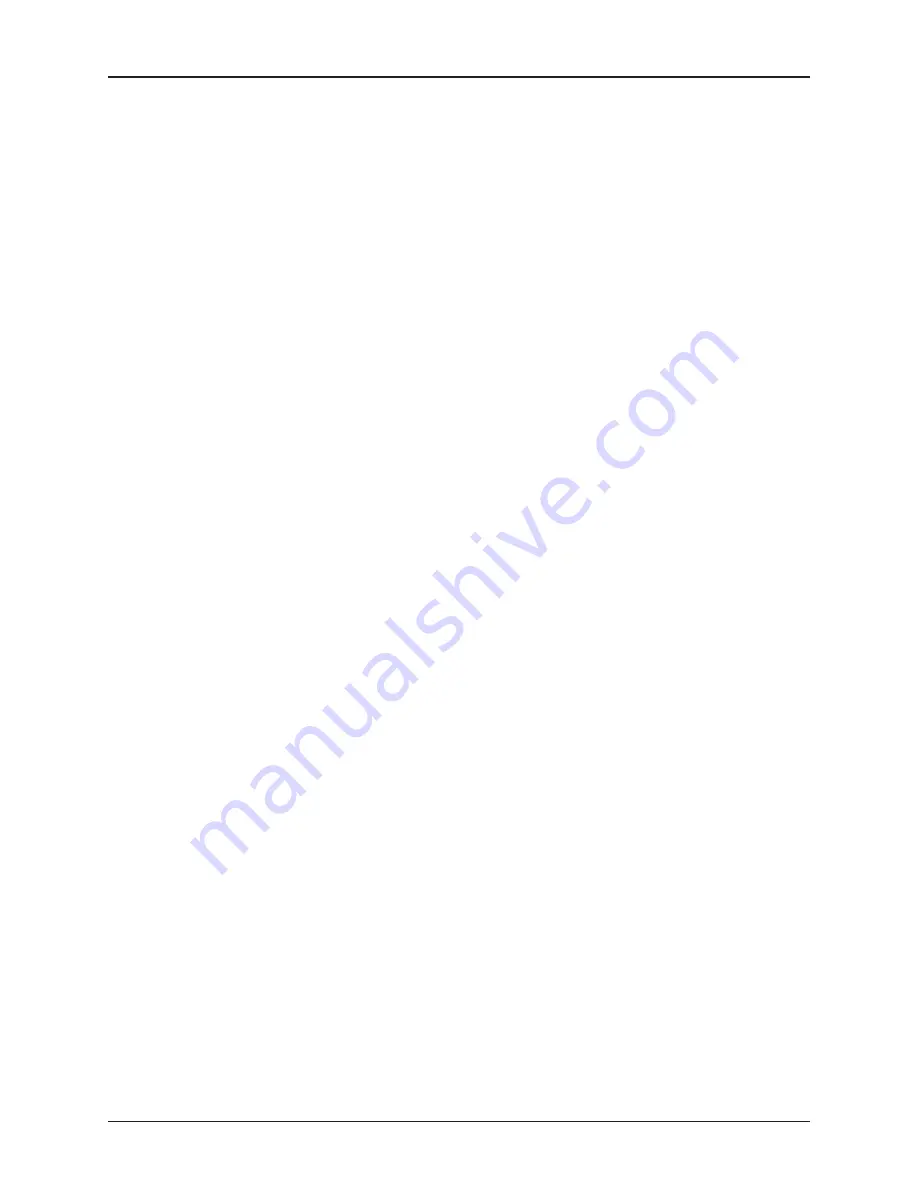
7.5
Programming Examples
7.5.1
Using the SMIC Interface
The PP 110/01x single board utilizes the Server Management Interface Controller (SMIC) as its
interface with the I/O ports at the standard addresses (i.e. 0CA9h, 0CAAh and 0CABh). The
IPMI specification has a complete description of the SMIC interface. The following C program
fragment reads and writes IPMI messages using the SMIC interface:
/* Addresses of SMIC registers */
#define SMIC_DATA
0x0CA9 /* Data Register */
#define SMIC_CONTROL
0x0CAA /* Control & Status Register */
#define SMIC_FLAGS
0x0CAB /* Flag Register */
/* SMS Transfer Stream Control Codes */
#define CC_SMS_GET_STATUS
0x40
#define CC_SMS_WR_START
0x41
#define CC_SMS_WR_NEXT
0x42
#define CC_SMS_WR_END
0x43
#define CC_SMS_RD_START
0x44
#define CC_SMS_RD_NEXT
0x45
#define CC_SMS_RD_END
0x46
/* SMS Transfer Stream Status Codes */
#define SC_SMS_RDY
0xC0
#define SC_SMS_WR_START
0xC1
#define SC_SMS_WR_NEXT
0xC2
#define SC_SMS_WR_END
0xC3
#define SC_SMS_RD_START
0xC4
#define SC_SMS_RD_NEXT
0xC5
#define SC_SMS_RD_END
0xC6
/* Masks for SMIC Flags Registers Bits */
#define FLAG_RX_DATA_RDY
0x80
#define FLAG_TX_DATA_RDY
0x40
#define FLAG_SMI
0x10
#define FLAG_EVT_ATN
0x08
#define FLAG_SMS_ATN
0x04
#define FLAG_BUSY
0x01
#define FLAGS_BUSY
0x01
#define FLAGS_TX_DATA_READY 0x40
#define FLAGS_RX_DATA_READY 0x80
/* macros */
#define bReadSmicFlags (inb (SMIC_FLAGS))
#define bReadSmicStatus (inb (SMIC_CONTROL))
#define bReadSmicData
(inb (SMIC_DATA))
#define vWriteSmicControl(data)
outb(SMIC_CONTROL, data)
#define vWriteSmicData(data)
outb(SMIC_DATA, data)
#define vWriteSmicFlags(data)
outb(SMIC_FLAGS, data)
/******************************************************************************
*
* vBmcSmicSmsMessageWrite
*
* This function writes a SMS (System Managment Software) messages
* to the IPMI using the standard BMC-SMIC interface.
PP 110/01x
7-13
Intelligent Platform Manager Interface
Artisan Technology Group - Quality Instrumentation ... Guaranteed | (888) 88-SOURCE | www.artisantg.com