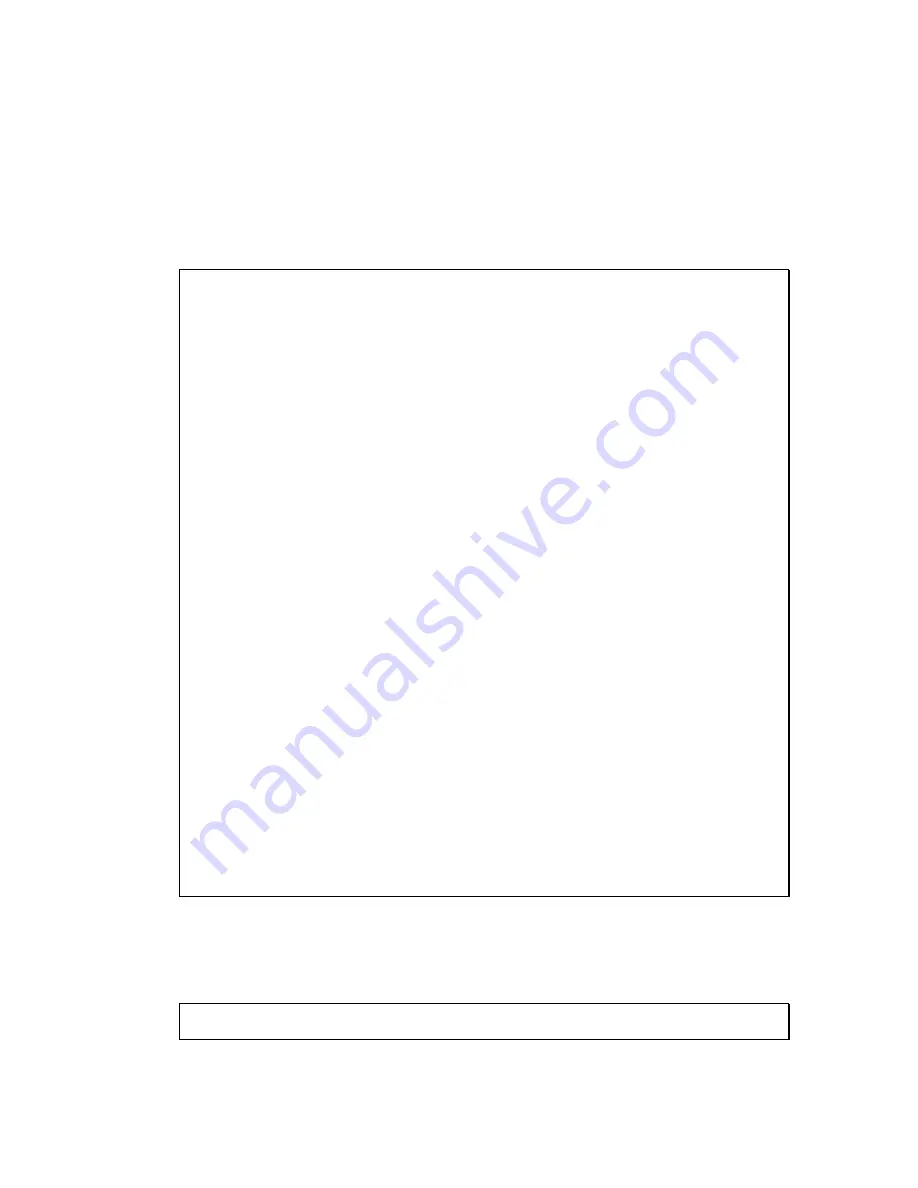
4-8
Real-Time Clock
The real-time clock can be used to keep track of real time. Backed up by a lithium-coin battery, the real
time clock can be accessed and programmed using two interface functions.
The real time clock only allows storage of two digits of the year code, as reflected below. As a result,
application developers should be careful to account for a roll-over in digits in the year 2000. One solution
might be to store an offset value in non-volatile storage such as the EEPROM.
A common data structure is used to access and use both interfaces.
typedef struct{
unsigned char sec1; One second digit.
unsigned char sec10; Ten second digit.
unsigned char min1; One minute digit.
unsigned char min10; Ten minute digit.
unsigned char hour1; One hour digit.
unsigned char hour10; Ten hour digit.
unsigned char day1; One day digit.
unsigned char day10; Ten day digit.
unsigned char mon1; One month digit.
unsigned char mon10; Ten month digit.
unsigned char year1; One year digit.
unsigned char year10; Ten year digit.
unsigned char wk; Day of the week.
} TIM;
int rtc_rd
Arguments: TIM *r
Return value: int error_code
This function places the current value of the real time clock within the argument r structure. The structure
should be allocated by the user. This function returns 0 on success and returns 1 in case of error, such as
the clock failing to respond.
Void rtc_init
Arguments: char* t
Return value: none
This function is used to initialize and set a value into the real-time clock. The argument t should be a null-
terminated byte array that contains the new time value to be used.
The byte array should correspond to { weekday, year10, year1, month10, month1, day10, day1, hour10,
hour1, minute10, minute1, second10, second1, 0 }.
If, for example, the time to be initialized into the real time clock is June 5, 1998, Friday, 13:55:30, the byte
array would be initialized to:
unsigned char t[14] = { 5, 9, 8, 0, 6, 0, 5, 1, 3, 5, 5, 3, 0 };
Delay
In many applications it becomes useful to pause before executing any further code. There are functions
provided to make this process easy. For applications that require precision timing, you should use the
hardware timers provided on-board for this purpose.
void delay0
Arguments: unsigned int t