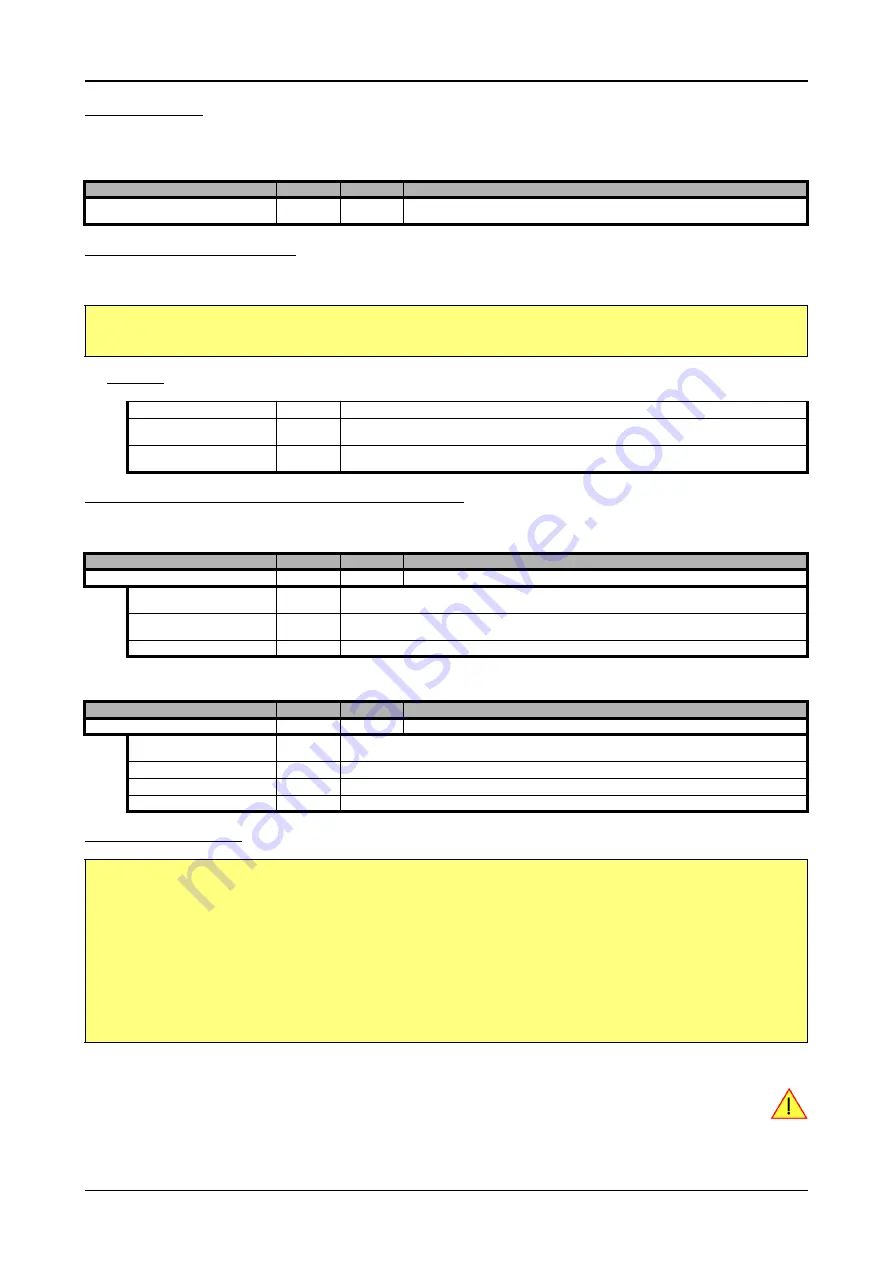
Acquisition modes
Commands
(c) Spectrum GmbH
73
Memory test mode
In some cases it might be of interest to transfer data in the opposite direction. Therefore a special memory test mode is available which allows
random read and write access of the complete on-board memory. While memory test mode is activated no normal card commands are pro-
cessed:
Invalidation of the transfer buffer
The command can be used to invalidate an already defined buffer if the buffer is about to be deleted by user. This function is automatically
called if a new buffer is defined or if the transfer of a buffer has completed
The dwBufType parameter need to be the same parameter for which the buffer has been defined:
Commands and Status information for data transfer buffers.
As explained above the data transfer is performed with the same command and status registers like the card control. It is possible to send
commands for card control and data transfer at the same time as shown in the examples further below.
The data transfer can generate one of the following status information:
Example of data transfer
To keep the example simple it does no error checking. Please be sure to check for errors if using these command in real world programs!
Users should take care to explicitly send the M2CMD_DATA_STOPDMA command prior to invalidating the
buffer, to avoid crashes due to race conditions when using higher-latency data transportation layers, such
as to remote Ethernet devices.
Register
Value
Direction
Description
SPC_MEMTEST
200700
read/write
Writing a 1 activates the memory test mode, no commands are then processed.
Writing a 0 deactivates the memory test mode again.
uint32 _stdcall spcm_dwInvalidateBuf ( // invalidate the transfer buffer
drv_handle hDevice, // handle to an already opened device
uint32 dwBufType); // type of the buffer to invalidate as listed above under SPCM_BUF_XXXX
SPCM_BUF_DATA
1000
Buffer is used for transfer of standard sample data
SPCM_BUF_ABA
2000
Buffer is used to read out slow ABA data. Details on this mode are described in the chapter about the ABA mode
option. The ABA mode is only available on analog acquisition cards.
SPCM_BUF_TIMESTAMP
3000
Buffer is used to read out timestamp information. Details on this mode are described in the chapter about the times-
tamp option. The timestamp mode is only available on analog or digital acquisition cards.
Register
Value
Direction
Description
SPC_M2CMD
100
write only
Executes a command for the card or data transfer
M2CMD_DATA_STARTDMA
10000h
Starts the DMA transfer for an already defined buffer. In acquisition mode it may be that the card hasn’t received a
trigger yet, in that case the transfer start is delayed until the card receives the trigger event
M2CMD_DATA_WAITDMA
20000h
Waits until the data transfer has ended or until at least the amount of bytes defined by notify size are available. This
wait function also takes the timeout parameter described above into account.
M2CMD_DATA_STOPDMA
40000h
Stops a running DMA transfer. Data is invalid afterwards.
Register
Value
Direction
Description
SPC_M2STATUS
110
read only
Reads out the current status information
M2STAT_DATA_BLOCKREADY
100h
The next data block as defined in the notify size is available. It is at least the amount of data available but it also can
be more data.
M2STAT_DATA_END
200h
The data transfer has completed. This status information will only occur if the notify size is set to zero.
M2STAT_DATA_OVERRUN
400h
The data transfer had on overrun (acquisition) or underrun (replay) while doing FIFO transfer.
M2STAT_DATA_ERROR
800h
An internal error occurred while doing data transfer.
void* pvData = (void*) new int8[1024];
// transfer data from PC memory to card memory (on replay cards) ...
spcm_dwDefTransfer_i64 (hDrv, SPCM_BUF_DATA, SPCM_DIR_PCTOCARD , 0, pvData, 0, 1024);
spcm_dwSetParam_i32 (hDrv, SPC_M2CMD, M2CMD_DATA_STARTDMA | M2CMD_DATA_WAITDMA);
// ... or transfer data from card memory to PC memory (acquisition cards)
spcm_dwDefTransfer_i64 (hDrv, SPCM_BUF_DATA, SPCM_DIR_CARDTOPC , 0, pvData, 0, 1024);
spcm_dwSetParam_i32 (hDrv, SPC_M2CMD, M2CMD_DATA_STARTDMA | M2CMD_DATA_WAITDMA);
// explicitely stop DMA tranfer prior to invalidating buffer
spcm_dwSetParam_i32 (hDrv, SPC_M2CMD, M2CMD_DATA_STOPDMA);
spcm_dwInvalidateBuf (hDrv, SPCM_BUF_DATA);
delete [] (int8*) pvData;