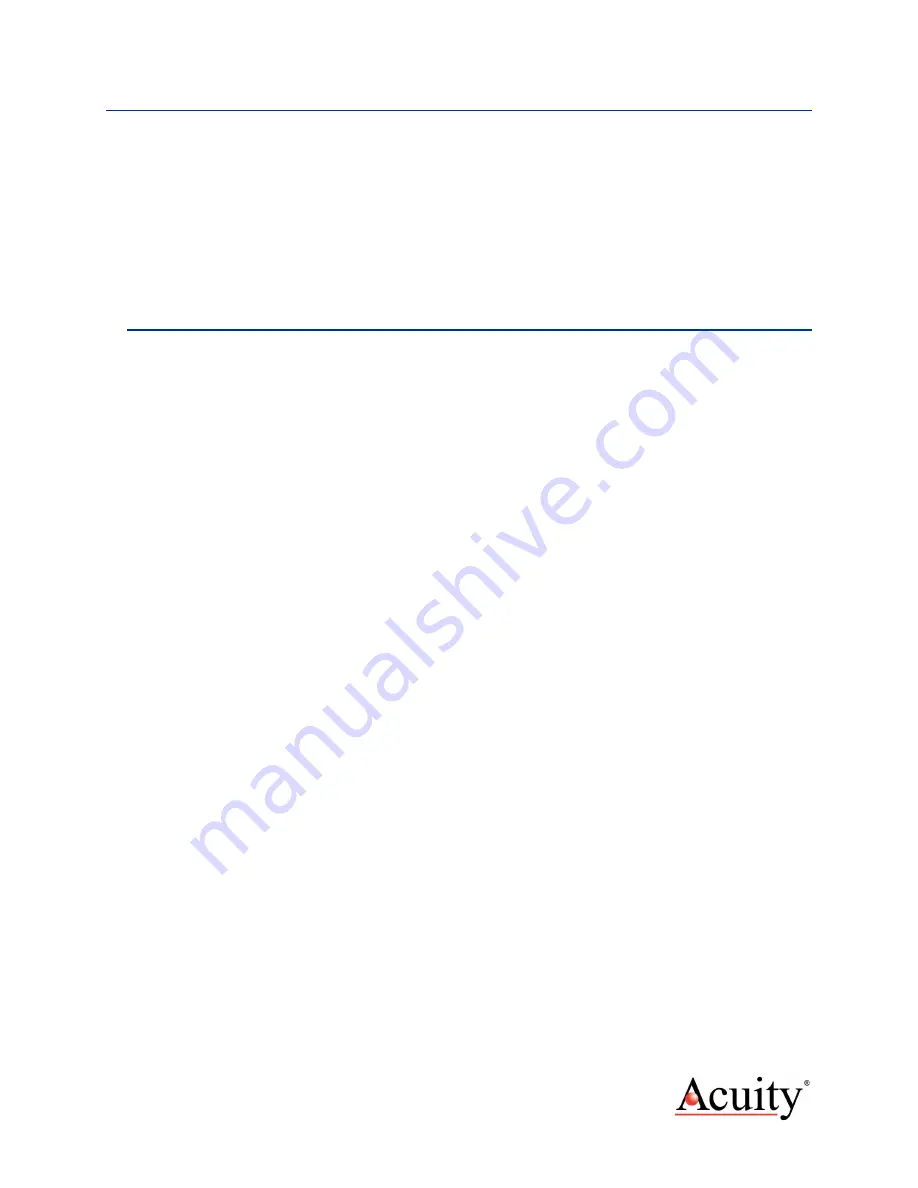
Acuity AP820 Laser Scanners
Rev. 3.3
31
11.
Programming using Windows DLL Calls
The following discussion is an attempt to show, step by step, what is required to set up,
program, and get results from an AP820 laser using Windows DLL function calls. To
illustrate the use of the Windows DLL functions, this document presents examples of how to
call each of the functions from the
Visual Basic DotNet
environment. Visual Basic DotNet
was selected because it is the most straightforward to use of the Windows programming
languages. Experienced C++ or C# programmers should not have difficulty translating the
methodology into their programming language. Note the variation between C++ and VB
hexadecimal expressions. The discussion below assumes familiarity with Visual Basic DotNet,
and associated terms and concepts such as managed and unmanaged code, etc.
11.1
Importing EthernetScanner.dll
The functions that access the AP820 are exported in a library called EthernetScanner.dll.
These functions are:
EthernetScanner_Connect
EthernetScanner_Disconnect
EthernetScanner_GetConnectStatus
EthernetScanner_GetScanRawData
EthernetScanner_GetInfo
EthernetScanner_WriteData
EthernetScanner_GetVersion
We will import these into VB.net using the DllImport attribute in the
System.Runtime.InteropServices namespace, which we will reference at the top of the
module containing the code to import the dll functions.
Imports
System.Runtime.InteropServices
Then, we use DllImport to import the functions from the dll.
<DllImport(
"EthernetScanner.dll"
)>
Private Function
EthernetScanner_Connect(
ByVal
IPAddr
As String
,
ByVal
Port
As String
,
ByVal
Timeout
As UInteger
)
As
IntPtr
End Function
<DllImport(
"EthernetScanner.dll"
)>
Private Function
EthernetScanner_Disconnect(
ByVal
pScanner
As
IntPtr)
As
UInteger
End Function
<DllImport(
"EthernetScanner.dll"
)> _
Private Sub
EthernetScanner_GetConnectStatus(
ByVal
pScanner
As
IntPtr,
ByRef
Status
As UInteger
)
End Sub
<DllImport(
"EthernetScanner.dll"
)> _
Private Function
EthernetScanner_GetInfo(
ByVal
pScanner
As
IntPtr,
ByRef
ScanInfo
As
ScanInfoStruct,
ByVal
ScanInfoSize
As UInteger
,
ByVal
Timeout