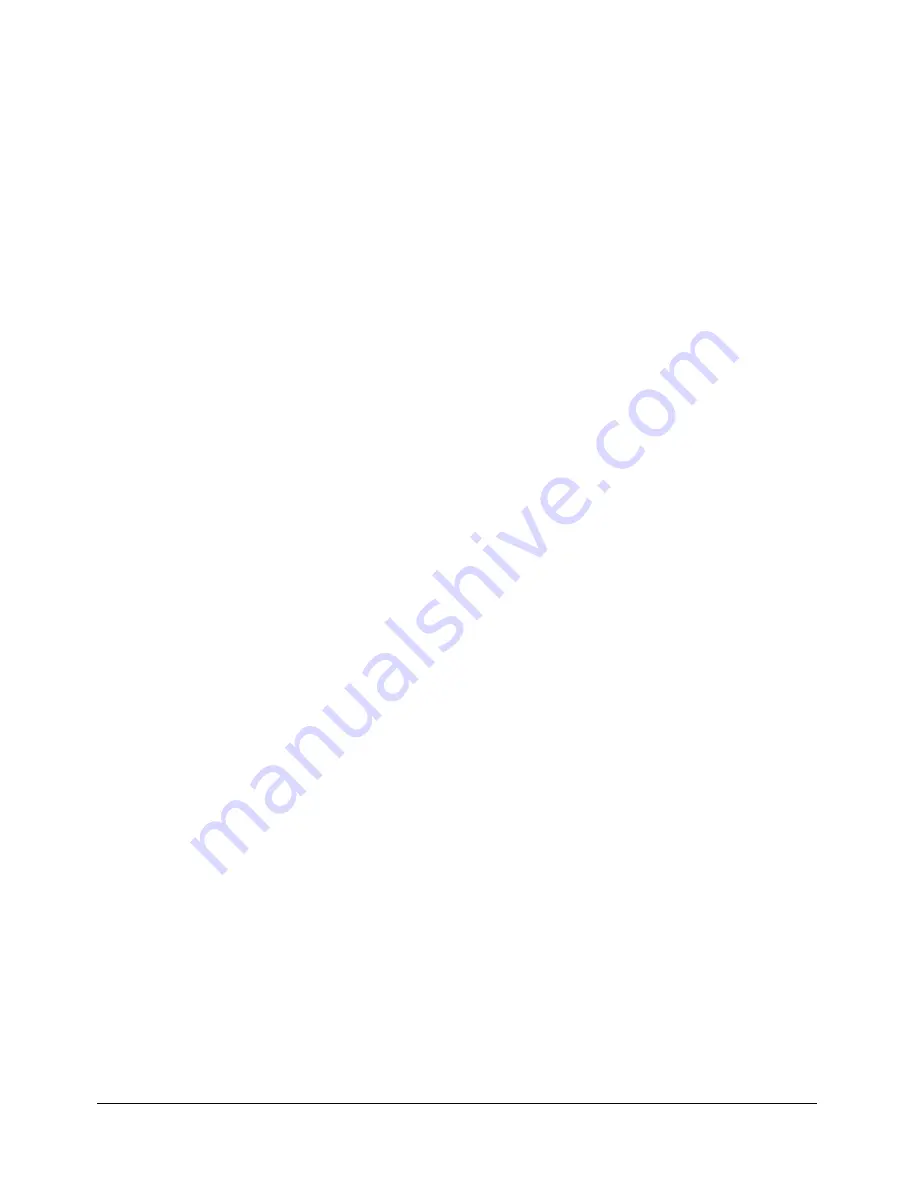
72
Chapter 4: Using Flash Remoting Data in ActionScript
The following example shows a Java class service function that creates a typed object and returns
it to Flash:
package mycompany.flash;
import flashgateway.io.ASObject;
public class MyFlashService
{
public MyFlashService()
{
}
public ASObject getFlashObject()
{
ASObject aso = new ASObject("MyFlashObject");
aso.put("first", "apple");
aso.put("second", "banana");
return aso;
}
}
Note that this example specifies the object type, MyFlashObject, in the constructor.
To create a Flash typed object in ColdFusion, use the
cfobject
tag or the
CreateObject
function, specifying the type as
Java
and class as
flashgateway.io.ASObject
. Then use the
object’s
setType()
method to set the Flash object type name. The following CFML code is the
equivalent to the Java code:
<cffunction access="remote" name="getFlashObject">
<cfobject type="JAVA" class="flashgateway.io.ASObject" name="myOb
" action="CREATE" >
<cfset myobj.setType("MyFlashObject")>
<cfset myobj.put("first", "apple")>
<cfset myobj.put("second", "banana")>
<cfreturn myobj>
</cffunction>
Working withJava serializable objects
If a service function returns an object that implements the Java Serializable interface, its public
and private properties are available as ActionScript properties. For example, a Java service method
might return the following JavaBean as the result of a Flash Remoting method invocation. In this
case, all three private properties, text, recipient, and server, are available to Flash.
public class Message implements java.io.Serializable
{
private String text;
private String recipient;
private String server;
public Message()
{
this.text = "Default message";
this.recipient = "[email protected]";
this.server = "smtp.macromedia.com";
}