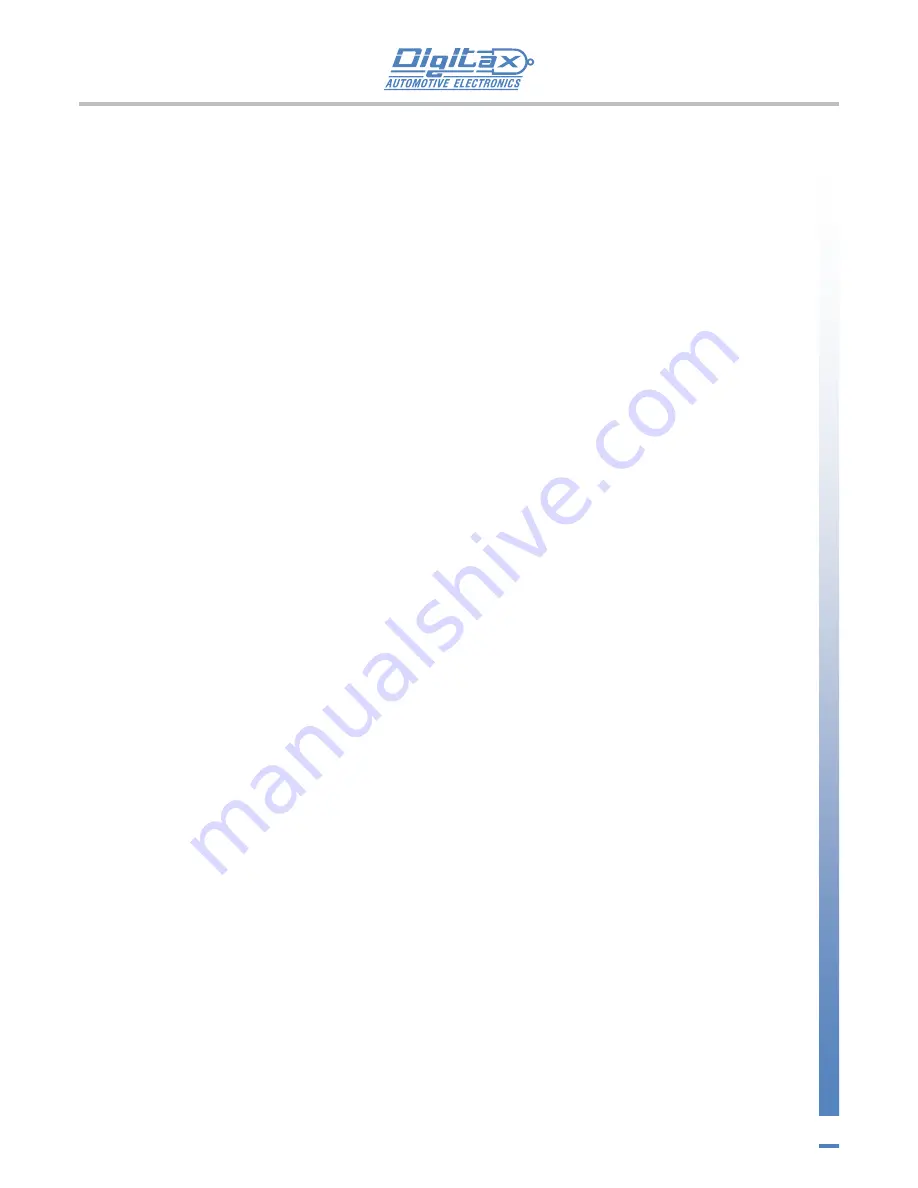
41
ComTax2 (Taximeter)
This application shows how to use COMTAX2 protocol to interact with a Digitax Taximeter. It
demonstrates how to ask for some data to the taximeter (e.g. Odometer, Status…) and how to
interpret taximeter answers. For a complete specification, please refer to the COMTAX2 protocol
guide.
Sample code:
using
System;
using
System.Collections.Generic;
using
System.ComponentModel;
using
System.Data;
using
System.Drawing;
using
System.Text;
using
System.Windows.Forms;
namespace
Digitax.Sample.ComTax2Test
{
//simple program that shows a simple way to interact with the embedded or external taximeter
public
partial
class
Comtax2TestForm
:
Form
{
//camtax 2 wrapper instance
ComTax2.Wrapper41.
ComTax2Core
_ct2 =
new
ComTax2.Wrapper41.
ComTax2Core
();
public
Comtax2TestForm()
{
InitializeComponent();
this
.C=
new
CancelEventHandler
(Comtax2TestForm_Closing);
_ct2.EvCT2TxRxCa=
new
ComTax2.Wrapper41.
ComTax2Core
.
delCT2SetTxRxCallback
(_ct2_EvCT2TxRxCallback);
bool
inited =
false
;
//inizialize comtax 2 wrapper
inited = _ct2.Init(
"COM3:"
, 38400,
true
, 1500, 1500, 2,
new
byte
[10000], 10000);
WriteLog(
"Comtax2 Inited: "
+ inited.ToString());
}
void
_ct2_EvCT2TxRxCallback(
ushort
RemoteDevID,
string
Command,
byte
EventCode,
byte
[] RxMsgBuff,
uint
RxMsgLen,
ushort
RxLastPackLen,
uint
lpParam)
{
// for the complete list of commands and their description please refer to the Comtax2 Protocol manual
if
(EventCode == (
byte
)ComTax2.Wrapper41.
EvtCodeEnum
.COMTAX2_RX_MSGOK &&
RemoteDevID == (
byte
)ComTax2.Wrapper41.
EnumDest
.DEST_TAX)
{
switch
(Command)
{
case
"AR"
:
//Extended status message
string
dispMain, dispSecond, dispTariff;
ASCIIEncoding
asc =
new
ASCIIEncoding
();
dispMain = asc.GetString(RxMsgBuff, 0 + 1, 8);
dispSecond = asc.GetString(RxMsgBuff, 8 + 1, 8);
dispTariff = asc.GetString(RxMsgBuff, 26 + 1, 1);
WriteLog(
"Ext. Status Recived: |"
+ disp
"|"
+ di
"|"
+ disp
"|"
);
break
;
case
"CD"
:
//Clock Data message
DateTime
taxTime =
new
DateTime
((RxMsgBuff[4]) | RxMsgBuff[5] << 8, RxMsgBuff[2], RxMsgBuff[3],
RxMsgBuff[1], RxMsgBuff[0], 0);
bool
legalHour = (RxMsgBuff[6] != 0x00);
WriteLog(
"Taximeter clock : "
+ taxTime.ToString(
"G"
) +
" Daylaight Saving: "
+
legalHour.ToString());
break
;
case
"SM"
:
//Taximeter Status message
string
status =
""
;
if
(RxMsgBuff[0] == 0x01) status =
"FOR HIRE"
;
else
if
(RxMsgBuff[0] == 0x02) status =
"HIRED"
;
else
if
(RxMsgBuff[0] == 0x03) status =
"STOPPED"
;
else
if
(RxMsgBuff[0] == 0x04) status =
"SPECIAL FUNCTION"
;
else
if
(RxMsgBuff[0] == 0xFF) status =
"DISABLED"
;
WriteLog(
"Taximiter Status : "
+ status);
break
;
case
"RQ"
:
//Odometer data message
uint
meters;
ushort
speed;
meters = (
uint
)(RxMsgBuff[0] | RxMsgBuff[1] << 8 | RxMsgBuff[2] << 16 | RxMsgBuff[3] << 24);
speed = (
ushort
)(RxMsgBuff[4] | RxMsgBuff[5] << 8);
WriteLog(
"Odometer - Meters: "
+ meters.ToString() +
" Speed: "
+ speed);
break
;
}
}
}
private
void
btExtStat_Click(
object
sender,
EventArgs
e)
{
bool
res;
//Request Extended status
res = _ct2.SendMsg((
ushort
)ComTax2.Wrapper41.
EnumDest
.DEST_TAX,
new
byte
[] { (
byte
)
'A'
, (
byte
)
'S'
},
new
byte
[0], 0);
WriteLog(
"Extended Status Request Deliverd: "
+ res.ToString());
}