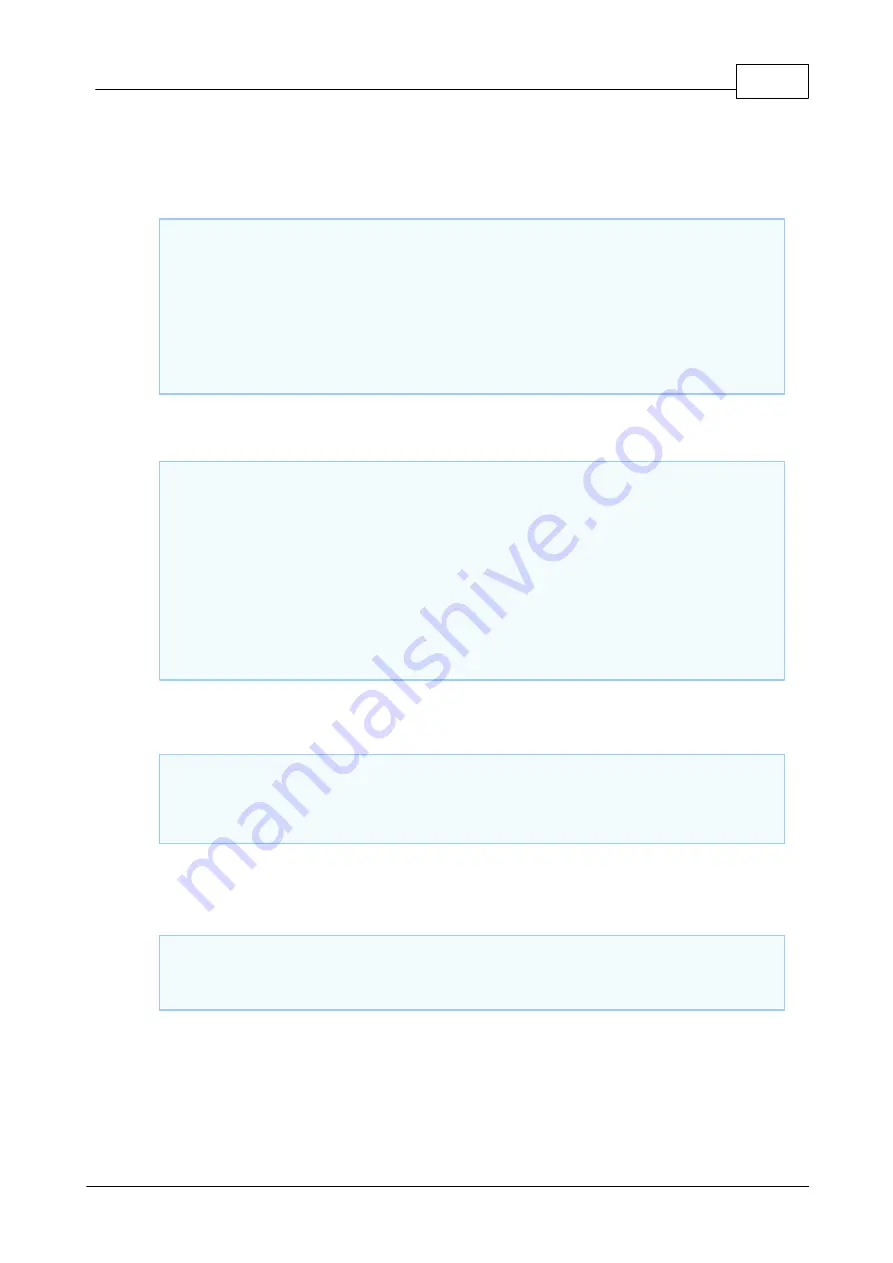
72
Programming with TIDE
©2000-2008 Tibbo Technology Inc.
#if - #else - #elif -- #endif
These directives are used to conditionally include certain portions of the source
code into the compilation process (or exclude from it). Here is an example:
#define OPTION 0
'set to 0, 1, or 2 to select different blocks of code for
compilation
...
#
If
OPTION=0
s="ABC"
'will be compiled when OPTION=0
#elif OPTION=1
s="DEF"
'will be compiled when OPTION=1
#
Else
s="123"
'will be compiled when OPTION=2, 3, etc.
#endif
You can improve on this example and add meaning to 0, 1, and 2:
#define RED 0
#define GREEN 1
#define BLUE 2
...
#define OPTION BLUE
...
#
If
OPTION=RED
s="ABC"
'will be compiled when OPTION=RED (0)
#elif OPTION=GREEN
s="DEF"
'will be compiled when OPTION=GREEN (1)
#
Else
s="123"
'will be compiled when neither RED, nor GREEN
#endif
You can also write like this:
#
If
OPTION
x=33
'will be compiled in if OPTION evaluates to any value except 0.
Will not be compiled in if OPTION evaluates to 0.
#endif
Preprocessor directives are not "strict". You don't have to define something before
using it. During #if evaluation, all undefined identifiers will be replaced with 0:
#
If
WEIRDNESS
'undefined identifier
x=33
'will not be compiled in
#endif
Do not confuse compile-time definitions such as "#define OPTION 2" and actual
application code like "const COLOR=2" or "x=3". They are from two different
worlds and you can't use the latter as part of #if directives. For example, the
following will not work: