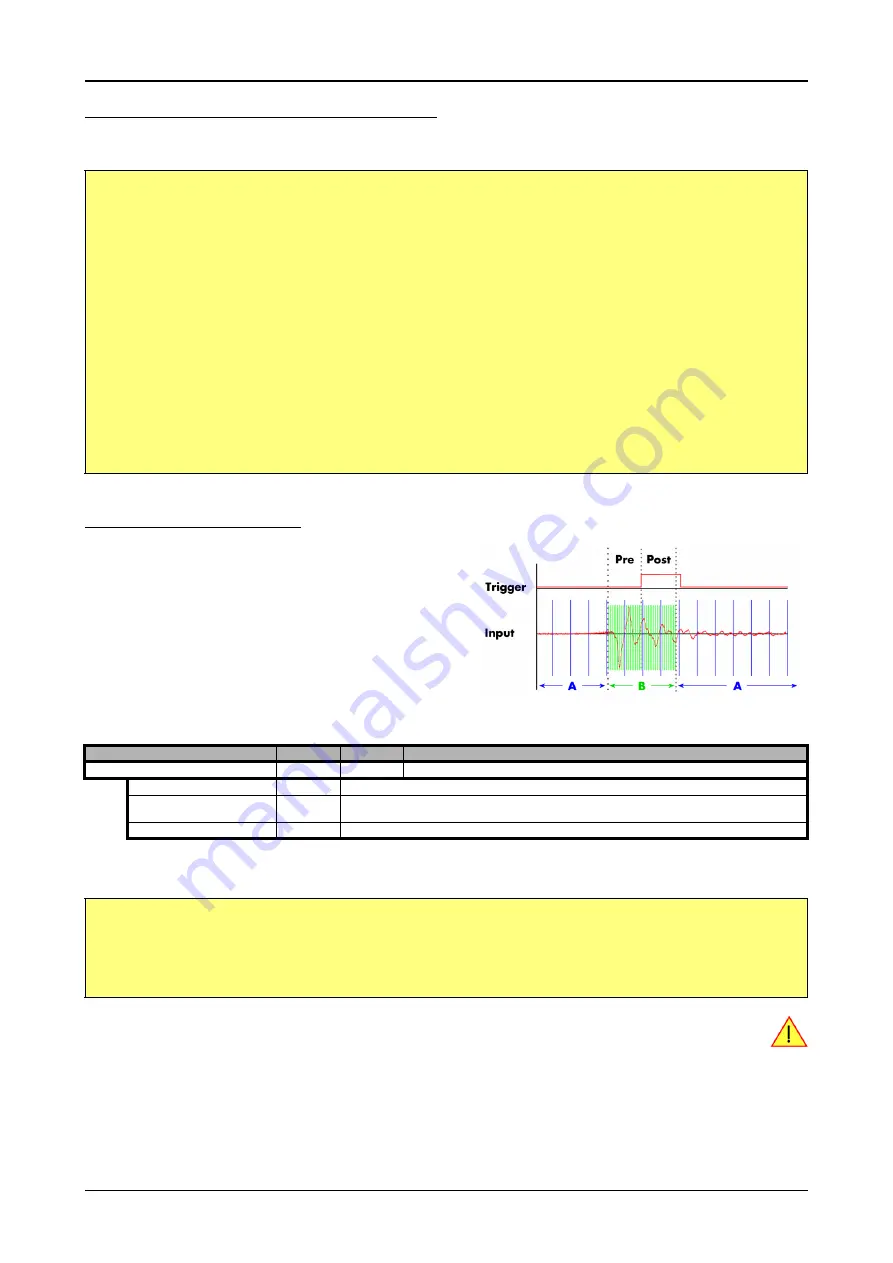
Timestamps
Combination of Memory Segmentation Options with Timestamps
(c) Spectrum GmbH
115
Example Multiple Recording and Timestamps
The following example shows the setup of the Multiple Recording mode together with activated timestamps recording and a short display of
the acquired timestamps. The example doesn’t care for the acquired data itself and doesn’t check for error:
ABA Mode and Timestamps
The ABA mode is well matching with the timestamp option. If timestamp
recording is activated, each trigger event and therefore each B time base
segment will get time tamped as shown in the drawing on the right.
Please keep in mind that the trigger events - located in the B area - are time
tamped, not the beginning of the acquisition. The first B sample that is
available is at the time position of [Timestamp - Pretrigger].
The first A area sample is related to the card start and therefore in a fixed
but various settings dependant relation to the timestamped B sample. To
bring exact relation between the first A area sample (and therefore all
area A samples) and the B area samples it is possible to let the card stamp
the first A area sample automatically after the card start. The following table shows the register to enable this mode:
This mode is compatible with all existing timestamp modes. Please keep in mind that the timestamp counter is running with the B area time-
base.
Using the cards in ABA mode with the timestamp feature to stamp the first A are sample requires the follow-
ing driver and firmware version depending on your card:
M2i: driver version V2.06 (or newer) and firmware version V16 (or newer)
M3i: driver version V2.06 (or newer) and firmware version V6 (or newer)
Please update your system to the newest versions to run this mode.
The programming details of the ABA mode and timestamp modes are each explained in a dedicated chapter in this manual.
// setup of the Multiple Recording mode
spcm_dwSetParam_i32 (hDrv, SPC_CARDMODE, SPC_REC_STD_MULTI); // Enables Standard Multiple Recording
spcm_dwSetParam_i32 (hDrv, SPC_SEGMENTSIZE, 1024); // Segment size is 1 kSample, Posttrigger is 768
spcm_dwSetParam_i32 (hDrv, SPC_POSTTRIGGER, 768); // samples and pretrigger therefore 256 samples.
spcm_dwSetParam_i32 (hDrv, SPC_MEMSIZE, 4096); // 4 kSamples in total acquired -> 4 segments
// setup the Timestamp mode and make a reset of the timestamp counter
spcm_dwSetParam_i32 (hDrv, SPC_TIMESTAMP_CMD, SPC_TSMODE_STANDARD | SPC_TSCNT_INTERNAL);
spcm_dwSetParam_i32 (hDrv, SPC_TIMESTAMP_CMD, SPC_TSMODE_RESET);
// now we define a buffer for timestamp data and start acquistion, each timestamp is 64 bit = 8 bytes
int64* pllStamps = new int64[4];
spcm_dwDefTransfer_i64 (hDrv, SPCM_BUF_TIMESTAMP, SPCM_DIR_CARDTOPC, 0, (void*) pllStamps, 0, 4 * 8);
spcm_dwSetParam_i32 (hDrv, SPC_M2CMD, M2CMD_CARD_START | M2CMD_CARD_ENABLETRIGGER | M2CMD_EXTRA_STARTDMA);
// we wait for the end timestamps transfer which will be received if all segments have been recorded
spcm_dwSetParam_i32 (hDrv, SPC_M2CMD, M2CMD_EXTRA_WAITDMA);
// as we now have the timestamps we just print them and calculate the time in milli seconds
int32 lSamplerate, lOver, i;
spcm_dwGetParam_i32 (hDrv, SPC_SAMPLERATE, &lSamplerate);
spcm_dwGetParam_i32 (hDrv, SPC_OVERSAMPLINGFACTOR, &lOver);
for (i = 0; i < 4; i++)
printf ("#%d: %I64d samples = %.3f ms\n", i, pllStamps[i], 1000.0 * pllStamps[i] / lSamplerate / lOver);
Register
Value
Direction
Description
SPC_TIMESTAMP_CMD
47000
read/write
Programs a timestamp setup including mode and additional features
SPC_TSFEAT_MASK
F0000h
Mask for the feature relating bits of the SPC_TIMESTAMP_CMD bitmask.
SPC_TSFEAT_STORE1STABA
10000h
Enables storage of one additional timestamp for the first A area sample (B time base related) in addition to the trigger
related timestamps.
SPC_TSFEAT_NONE
0h
No additional timestamp is created. The total number of stamps is only trigger related.
// normal timestamp setup (e.g. setting timestamp mode to standard using internal clocking)
uint32 dwTimestampMode = (SPC_TSMODE_STANDARD | SPC_TSMODE_DISABLE);
// additionally enable index of the first A area sample
dwTimestampMode |= SPC_TSFEAT_STORE1STABA;
spcm_dwSetParam_i32 (hDrv, SPC_TIMESTAMP_CMD, dwTimestampMode);