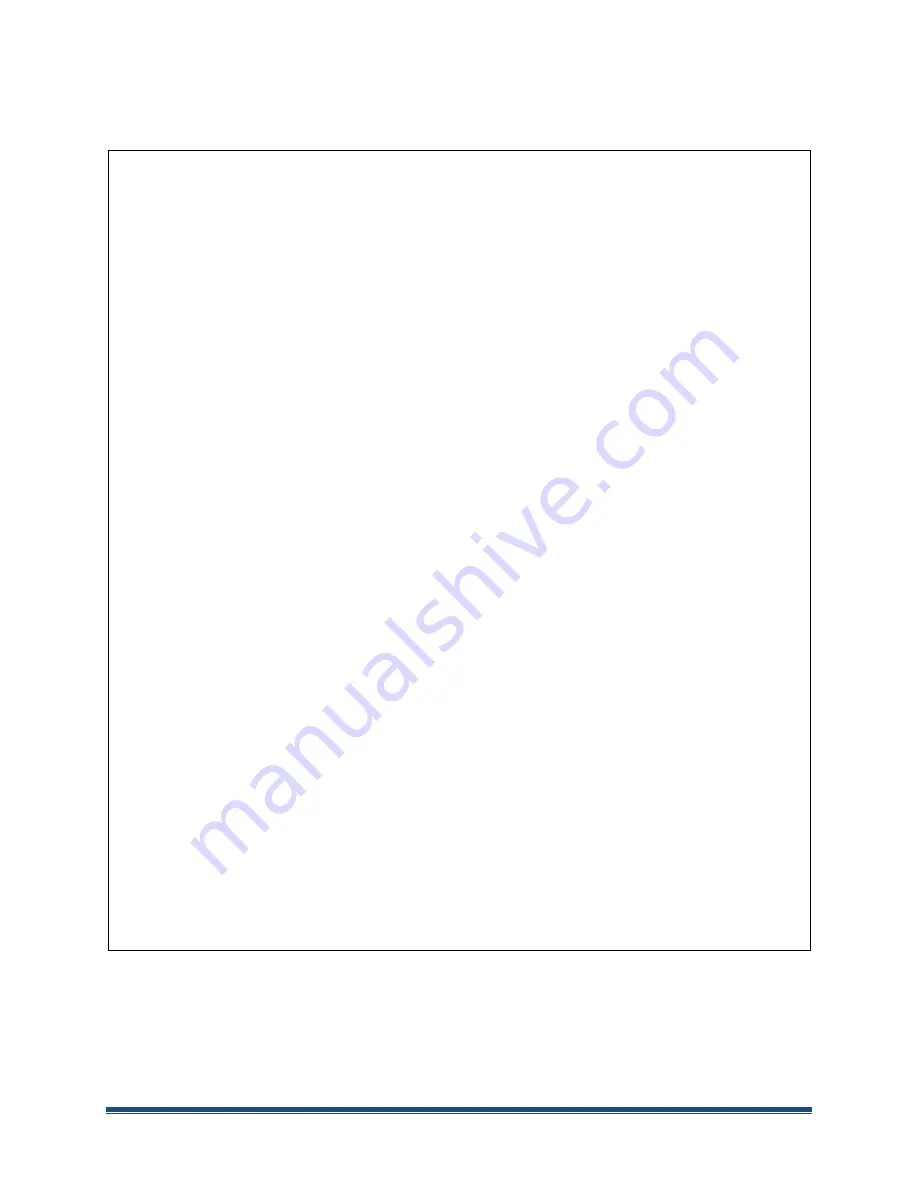
SC5305A Operating & Programming Manual
Rev 2.1.0
42
Example:
Allocating memory for the input and output parameters in C, and calling the function.
Similarly, allocated memory must be de-allocated when no longer used or when the
program quits.
//Declaring
calibrationData_t* calData;
deviceAttribute_t* devAttr;
unsigned
char
*rawCal;
//allocate memory for raw calibration
rawCal = (
unsigned
char
*)malloc(
sizeof
(
char
)*CALEEPROMSIZE);
// Allocate memory; the user may use malloc() instead of calloc()
devAttr->calDate = (
unsigned
int
*)calloc(4,
sizeof
(
unsigned
int
));
devAttr->manDate = (
unsigned
int
*)calloc(4,
sizeof
(
unsigned
int
));
calData->rfCal = (
float
**)calloc(RFCALPARAM,
sizeof
(
float
*));
for
(i = 0;i<RFCALPARAM;i++)
calData->rfCal[i] = (
float
*)calloc(RFCALFREQ,
sizeof
(
float
));
calData->ifAttenCal = (
float
**)calloc(IFATTENUATOR,
sizeof
(
float
*));
for
(i = 0;i<IFATTENUATOR;i++)
calData->ifAttenCal[i] = (
float
*)calloc(IFATTENCALVALUE,
sizeof
(
float
));
calData->ifFil0ResponseCal = (
float
**)calloc(IFRESPONSEPARAM,
sizeof
(
float
*));
for
(i = 0;i<IFRESPONSEPARAM;i++)
calData->ifFil0ResponseCal[i] = (
float
*)calloc(IFRESPONSEFREQ,
sizeof
(
float
));
calData->ifFil1ResponseCal = (
float
**)calloc(IFRESPONSEPARAM,
sizeof
(
float
*));
for
(i = 0;i<IFRESPONSEPARAM;i++)
calData->ifFil1ResponseCal[i] = (
float
*)calloc(IFRESPONSEFREQ,
sizeof
(
float
));
calData->tempCoeff = (
float
**)calloc(TEMPCOPARAM,
sizeof
(
float
*));
for
(i = 0;i<TEMPCOPARAM;i++)
calData->tempCoeff[i] = (
float
*)calloc(TEMPCOFREQ,
sizeof
(
float
));
//read in raw calibration
int
status = sc5305a_GetRawCal(deviceHandle, rawCal);
//Calling the function to structure the calibration data
status = sc5305a_convertRawCalData(rawCal, devAttr, calData);
//alternatively instead of calling the above 2 functions
status = sc5305a_GetCalData(deviceHandle, devAttr, calData);