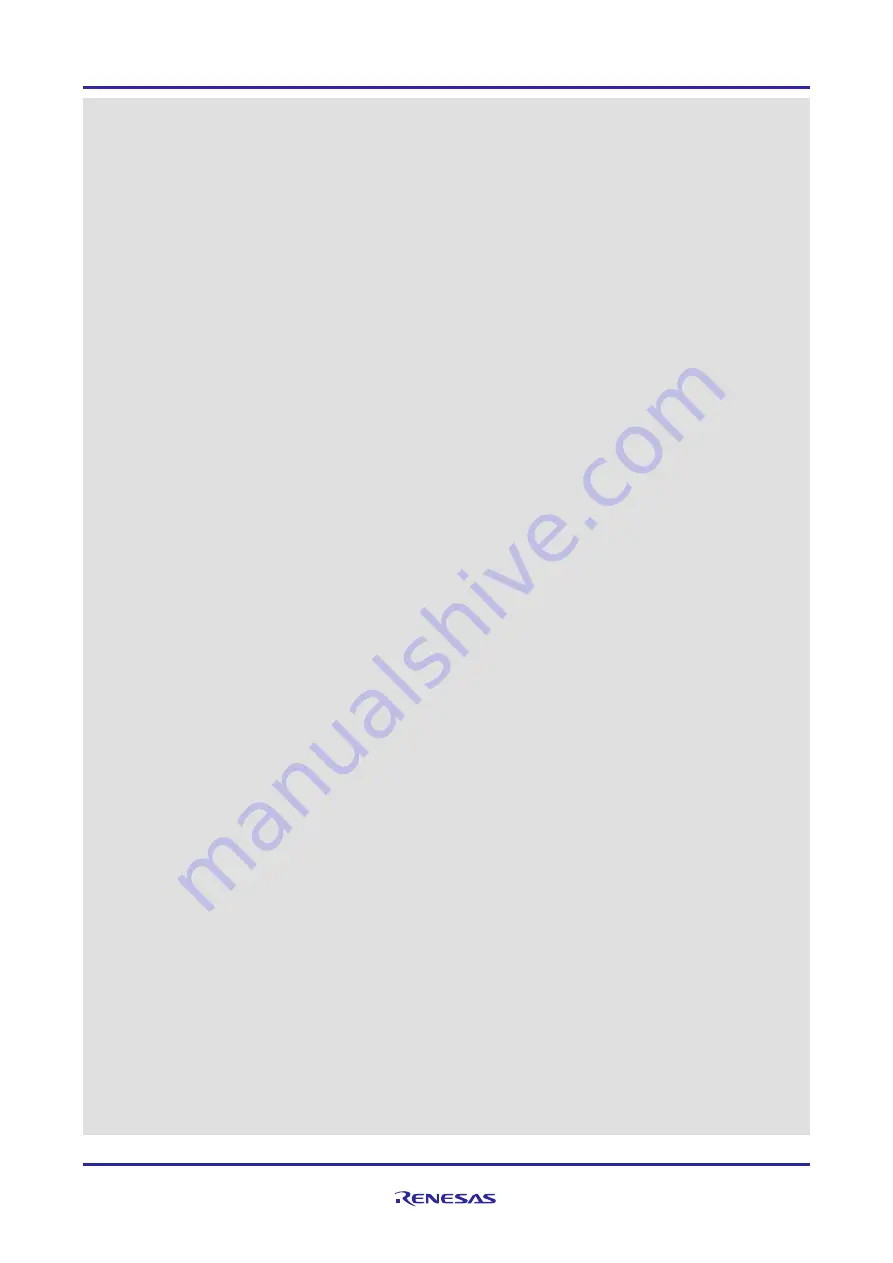
RX Family
ADC Module Using Firmware Integration Technology
R01AN1666EJ0220 Rev. 2.20
Page 48 of 74
Dec 01, 2016
#define
ADC_MASK_CH13 (
1
<<
13
)
#define
ADC_MASK_CH14 (
1
<<
14
)
#define
ADC_MASK_CH15 (
1
<<
15
)
#define
ADC_MASK_CH16 (
1
<<
16
)
#define
ADC_MASK_CH17 (
1
<<
17
)
#define
ADC_MASK_CH18 (
1
<<
18
)
#define
ADC_MASK_CH19 (
1
<<
19
)
#define
ADC_MASK_CH20 (
1
<<
20
)
#define
ADC_MASK_TEMP (
1
<<
21
)
// temperature sensor
#define
ADC_MASK_VOLT (
1
<<
22
)
// internal reference voltage sensor
#define
ADC_MASK_SENSORS (ADC_MASK_TEMP | ADC_MASK_VOLT)
#define
ADC_MASK_GROUPB_OFF (
0
)
#define
ADC_MASK_GROUPC_OFF (
0
)
#define
ADC_MASK_ADD_OFF (
0
)
#define
ADC_MASK_SAMPLE_HOLD_OFF (
0
)
typedef
enum
e_adc_grpa
// action when groupa interrupts groupb
scan
{
ADC_GRPA_PRIORITY_OFF =
0
,
// groupa ignored;does not interrupt groupb
ADC_GRPA_GRPB_GRPC_WAIT_TRIG = 1,
ADC_GRPA_GRPB_GRPC_TOP_RESTART_SCAN = 3,
ADC_GRPA_GRPB_GRPC_RESTART_TOP_CONT_SCAN = 0x8003,
ADC_GRPA_GRPB_GRPC_RESTART_SCAN = 0x4003,
ADC_GRPA_GRPB_GRPC_TOP_CONT_SCAN = 0x8001,
ADC_GRPA_GRPB_GRPC_RESTART_CONT_SCAN = 0xC003,
}
adc_grpa_t;
typedef
enum
e_adc_diag
// Self-Diagnosis Channel
{
ADC_DIAG_OFF =
0x00
,
ADC_DIAG_0_VOLT =
0x01
,
ADC_DIAG_HALF_VREFH0 =
0x2
,
ADC_DIAG_VREFH0 =
0x3
,
ADC_DIAG_ROTATE_VOLTS =
0x4
,
ADC_DIAG_END_ENUM
}
adc_diag_t;
#define
ADC_SST_SH_CNT_MIN (
4
)
// minimum sample&hold states
#define
ADC_SST_SH_CNT_MAX (
255
)
// maximum sample&hold states
#define
ADC_SST_SH_CNT_DEFAULT (
24
)
// default sample&hold states
typedef
struct
st_adc_ch_cfg
// bit 0 is ch0; bit 15 is ch15
{
uint32_t scan_mask;
// channels/bits 0-15
uint32_t scan_mask_groupb;
// valid for group modes
uint32_t scan_mask_groupc;
// valid for group modes
adc_grpa_t priority_groupa;
// valid for group modes
uint32_t add_mask;
// valid if add enabled in Open()
adc_diag_t diag_method;
// self-diagnosis virtual channel
bool
anex_enable;
// unit1: use external amplifier
uint8_t sample_hold_mask;
// channels/bits 0-2
uint8_t sample_hold_states;
// minimum .4us
}
adc_ch_cfg_t;
typedef
enum
e_adc_comp_cond
// Window A/B Complex Conditions
{
ADC_COND_OR =
0x00
,
ADC_COND_EXOR =
0x01
,
ADC_COND_AND =
0x02
,