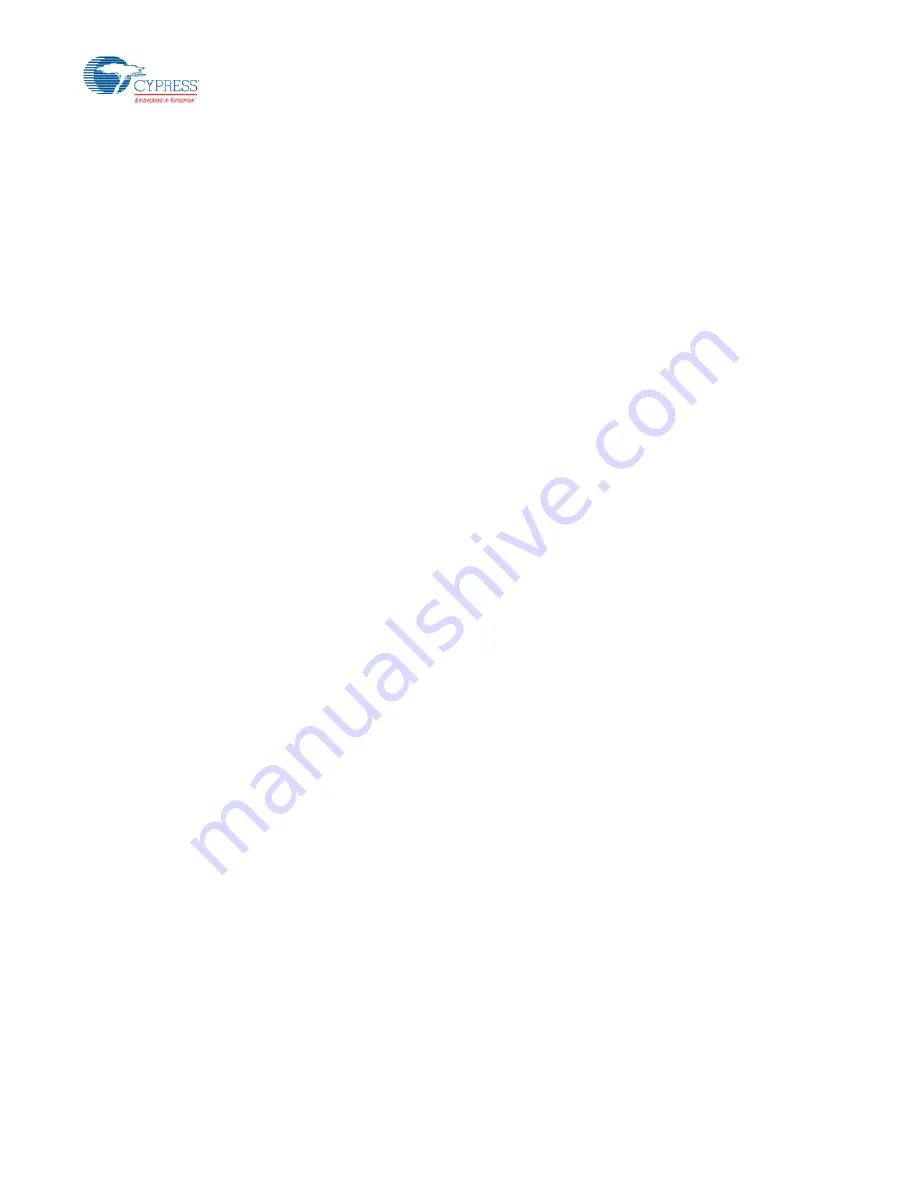
Overview
FM4, S6E2DH/S6E2DF/S6E2D5/S6E2D3 Series, 32-Bit Microcontroller, Graphic Driver User Manual, Doc. No. 002-04387 Rev. *A
23
4.5.3 Sample use cases
4.5.3.1
Double buffered window
A typical application must render a new frame content for each display loop. Double buffered frame buffers are used
to render the next frame in a background buffer while the foreground buffer memory is read by the display controller.
The following sample code shows how the application can use the 2D Graphics Driver Synchronization API to
realize a double buffered Window:
// This structure contains the objects required for a double buffered window.
struct
DOUBLE_BUFFERED_WINDOW
{
MML_GDC_DISP_WINDOW win; // the window handle
MML_GDC_SURFACE_CONTAINER sFramebuffer[2]; // Two buffers described as surface objects.
MML_GDC_SYNC_CONTAINER sync; // A sync object.
MM_U08 id; // An id storing which buffer is currently the foreground buffer.
};
// This is the draw function for the window including buffer swap and synchronization.
MM_ERROR draw(DOUBLE_BUFFERED_WINDOW *pdbWin)
{
// Return if the last render operation is still ongoing.
if
(mmlGdcSyncWait(&pdbWin->sync, 0) == MML_ERR_GDC_SYNC_TIMEOUT)
return
MML_OK;
// Bind new background buffer to render context.
mmlGdcPeBindSurface(&ctx, MML_GDC_PE_STORE |
MML_GDC_PE_DST, &pdbWin->sFramebuffer[pdbWin->id]);
// Render the next frame
mmlGdcPe..
mmlGdcPe..
mmlGdcPe..
// Get a sync object for the last blit operation ...
mmlGdcPeSync(&pdbWin->sync);
// ...and push it in the windows pipe. (It ensures that the new buffer becomes
//visible after the last blit is executed in the hardware.)
mmlGdcDispWinWaitSync(pdbWin->win, &pdbWin->sync));
// Swap the foreground and background layer on display.
mmlGdcDispWinSetSurface( pdbWin->win,
MML_GDC_DISP_BUFF_TARGET_COLOR_BUFF, &pdbWin->sFramebuffer[pdbWin->id] );
// Commit changes.
mmlGdcDispWinCommit( pdbWin->win );
// Get a sync object for this commit function for the next loop.
mmlGdcDispWinSync( pdbWin->win, &pdbWin->sync );