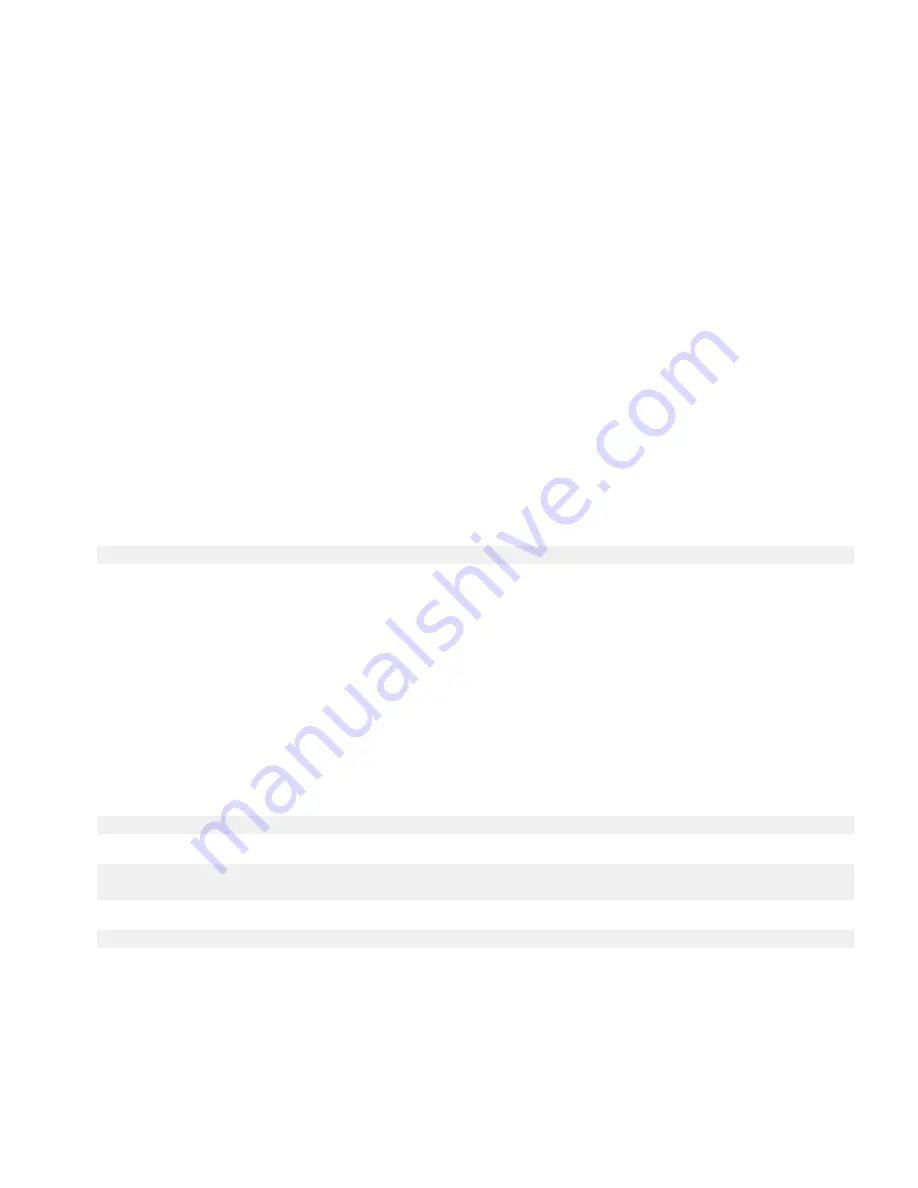
To use a datagram connection, you must have your own infrastructure to connect to the wireless network, including an APN for
GPRS networks. Using UDP connections requires that you work closely with service providers. Verify that your service provider
supports UDP connections.
1.
Import the following classes and interfaces:
•
net.rim.device.api.system.CoverageInfo
•
javax.microedition.io.Connector
•
java.lang.String
2.
Import the following interfaces:
•
net.rim.device.api.system.CoverageStatusListener
•
javax.microedition.io.DatagramConnection
•
javax.microedition.io.Datagram
3.
Use the
CoverageInfo
class and the
CoverageStatusListener
interface of the
net.rim.device.api.system
package to make sure that the BlackBerry device is in a wireless network coverage
area.
4.
Invoke
Connector.open()
, specify udp as the protocol and cast the returned object as a
DatagramConnection
object to open a datagram connection.
(DatagramConnection)Connector.open("udp://host:dest_port[;src_port]/apn");
where:
•
host
is the host address in dotted ASCII-decimal format.
•
dest-port
is the destination port at the host address (optional for receiving messages).
•
src-port
is the local source port (optional).
•
apn
is the network APN in string format.
5.
To receive datagrams from all ports at the specified host, omit the destination port in the connection string.
6.
To open a datagram connection on a non-GPRS network, specify the source port number, including the trailing slash mark.For
example, the address for a CDMA network connection would be udp://121.0.0.0:2332;6343/. You can send and receive
datagrams on the same port.
7.
To create a datagram, invoke
DatagramConnection.newDatagram()
.
Datagram outDatagram = conn.newDatagram(buf, buf.length);
8.
To add data to a diagram, invoke
Datagram.setData()
.
byte[] buf = new byte[256];
outDatagram.setData(buf, buf.length);
9.
To send data on the datagram connection, invoke
send()
on the datagram connection.
conn.send(outDatagram);
If a BlackBerry®Java® Application attempts to send a datagram on a datagram connection and the recipient is not listening
on the specified source port, an
IOException
is thrown. Make sure that the BlackBerry Java Application implements
exception handling.
Development Guide
Connections
59