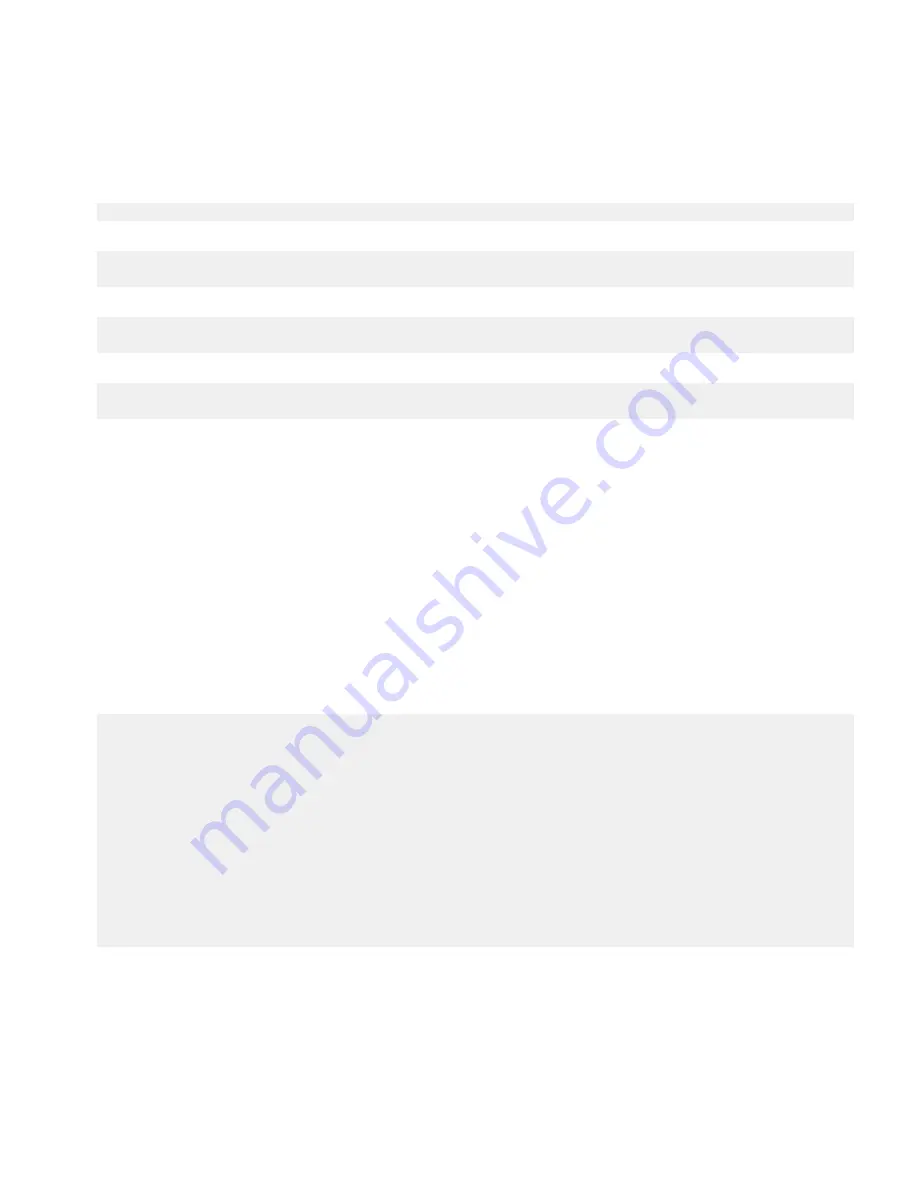
6.
Create an instance of a
ListCallback
.
ListCallback myCallback = new ListCallback();
7.
Set the call back of the
ListField
to be the
ListCallback
.
myList.setCallback(myCallback);
8.
Use the
ListCallBack
object to add items to the
ListField
.
myCallback.add(myList, fieldOne);
myCallback.add(myList, fieldTwo);
9.
Add the
ListField
to the
MainScreen
.
mainScreen.add(myList);
Create a field to display a parent and child relationship between items
A
TreeField
contains parent and child nodes.
1.
Import the following classes:
•
net.rim.device.api.ui.component.TreeField
•
java.lang.String
•
net.rim.device.api.ui.container.MainScreen
2.
Import the
net.rim.device.api.ui.component.TreeFieldCallback
interface.
3.
Implement the
TreeFieldCallback
interface.
4.
Invoke
TreeField.setExpanded()
on the
TreeField
object to specify whether a folder is collapsible. In the
following code sample, we create a
TreeField
object and multiple child nodes to the
TreeField
object. We then
invoke
TreeField.setExpanded()
using node4 as a parameter to collapse the folder.
String fieldOne = new String("Main folder");
...
TreeCallback myCallback = new TreeCallback();
TreeField myTree = new TreeField(myCallback, Field.FOCUSABLE);
int node1 = myTree.addChildNode(0, fieldOne);
int node2 = myTree.addChildNode(0, fieldTwo);
int node3 = myTree.addChildNode(node2, fieldThree);
int node4 = myTree.addChildNode(node3, fieldFour);
...
int node10 = myTree.addChildNode(node1, fieldTen);
myTree.setExpanded(node4, false);
...
mainScreen.add(myTree);
Development Guide
UI components
22